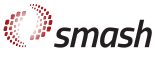 |
Version: SMASH-2.0.2
|
|
Go to the documentation of this file.
12 #include "HepMC3/Print.h"
13 #include "HepMC3/Setup.h"
19 const int total_N,
const int proj_N)
21 event_(HepMC3::Units::GEV, HepMC3::Units::MM),
27 full_event_(full_event) {
28 logg[
LOutput].debug() <<
"Name of output: " << name <<
" "
29 << (
full_event_ ?
"full event" :
"final state only")
30 <<
" output" << std::endl;
31 ion_ = std::make_shared<HepMC3::GenHeavyIon>();
32 xs_ = std::make_shared<HepMC3::GenCrossSection>();
34 HepMC3::Setup::set_debug_level(
logg[
LOutput].isEnabled<einhard::DEBUG>() ? 5
39 const int event_number,
45 ion_->impact_parameter =
event.impact_parameter;
46 xs_->set_cross_section(1, 1);
47 event_.set_event_number(event_number);
52 ip_ = std::make_shared<HepMC3::GenVertex>();
62 for (
auto& data : particles) {
64 if (!data.is_neutron() && !data.is_proton()) {
65 throw std::runtime_error(
66 "Particle of PID=" + std::to_string(data.pdgcode().get_decimal()) +
67 " is not a valid HepMC beam particle!");
70 bool isproj = data.id() <
proj_N_;
72 AZ& az = (isproj ? az_proj : az_targ);
74 az.second += data.is_proton();
90 ip_->add_particle_out(op);
92 ip_->add_particle_in(op);
101 ip_->add_particle_in(proj);
102 ip_->add_particle_in(targ);
108 HepMC3::GenVertexPtr vp;
114 vp = std::make_shared<HepMC3::GenVertex>(
115 HepMC3::FourVector(v.
x1(), v.
x2(), v.
x3(), v.
x0()));
116 vp->add_attribute(
"weight", std::make_shared<HepMC3::FloatAttribute>(
129 ip->set_status(status);
130 vp->add_particle_in(ip);
153 ion_->Ncoll_hard = -1;
158 ion_->Npart_proj = -1;
162 ion_->Npart_targ = -1;
167 for (
auto&
p : particles) {
170 ip_->add_particle_out(h);
171 }
else if (
map_.find(
p.id()) ==
map_.end()) {
172 throw std::runtime_error(
"Dangling particle " + std::to_string(
p.id()));
191 return static_cast<int>(t) +
static_cast<int>(Status::off);
197 auto p = std::make_shared<HepMC3::GenParticle>(
198 HepMC3::FourVector(mom.
x1(), mom.
x2(), mom.
x3(), mom.
x0()), pid, status);
200 p->set_generated_mass(mass);
207 auto h =
make_gen(
p.pdgcode().get_decimal(), status,
p.momentum(),
219 auto it =
map_.find(
id);
220 if (it !=
map_.end()) {
229 if (az.second == 1 && az.first == 1)
231 if (az.second == 1 && az.first == 0)
234 return 1
'000'000
'000 + az.second * 10'000 + az.first * 10;
virtual ProcessType get_type() const
Get the process type.
static constexpr int LOutput
IdMap map_
Mapping from ID to particle.
int ncoll_hard_
counter of hard binary collisions (e.g., where both incoming particles are from the beams.
void at_interaction(const Action &action, const double density) override
Writes collisions to event.
HepMC3::GenParticlePtr find_or_make(const ParticleData &p, int status=Status::fnal, bool force_new=false)
Find particle in mapping or generate it.
HepMC3::GenEvent event_
The event.
const int total_N_
Total number of nucleons in projectile and target, needed for converting nuclei to single particles.
virtual double get_partial_weight() const =0
Return the specific weight for the chosen outgoing channel, which is mainly used for the partial weig...
int ncoll_
counter of binary collisions (e.g., where both incoming particles are from the beams.
HepMC3::GenParticlePtr make_gen(int pid, int status, const smash::FourVector &mom, double mass=-1)
Make an HepMC particle.
Structure to contain custom data for output.
std::array< einhard::Logger<>, std::tuple_size< LogArea::AreaTuple >::value > logg
An array that stores all pre-configured Logger objects.
const ParticleList & outgoing_particles() const
Get the list of particles that resulted from the action.
HepMcInterface(const std::string &name, const bool full_event, const int total_N, const int proj_N)
Create HepMC particle event in memory.
HepMC3::GenCrossSectionPtr xs_
Dummy cross-section.
HepMC3::GenParticlePtr make_register(const ParticleData &p, int status=Status::fnal)
Find particle in mapping or generate it.
bool full_event_
Whether the full event or only final-state particles are in the output.
void at_eventend(const Particles &particles, const int32_t event_number, const EventInfo &event) override
Add the final particles information of an event to the central vertex.
Abstraction of generic output.
virtual double get_total_weight() const =0
Return the total weight value, which is mainly used for the weight output entry.
HepMC3::GenVertexPtr ip_
The interaction point.
int ion_pdg(const AZ &az) const
Encode ion PDG.
bool empty_event
True if no collisions happened.
int get_status(const ProcessType &t) const
Convert SMASH process type to HepMC status.
void clear()
Clear before an event.
HepMC3::GenHeavyIonPtr ion_
The heavy-ion structure.
void at_eventstart(const Particles &particles, const int event_number, const EventInfo &event) override
Add the initial particles information of an event to the central vertex.
FourVector get_interaction_point() const
Get the interaction point.
CollCounter coll_
Collision counter.
const ParticleList & incoming_particles() const
Get the list of particles that go into the action.
const int proj_N_
Total number of nucleons in projectile, needed for converting nuclei to single particles.
std::pair< int, int > AZ
Pair of Atomic weight and number.
ProcessType
Process Types are used to identify the type of the process.