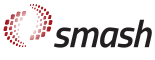 |
Version: SMASH-2.0
|
|
Go to the documentation of this file.
10 #ifndef SRC_INCLUDE_SMASH_CLOCK_H_
11 #define SRC_INCLUDE_SMASH_CLOCK_H_
25 static constexpr
int LClock = LogArea::Clock::id;
88 virtual void reset(
double start_time,
const bool is_output_clock) = 0;
106 if (
counter_ >= std::numeric_limits<Representation>::max() - 1) {
107 throw std::overflow_error(
"Too many timesteps, clock overflow imminent");
122 if (
counter_ >= std::numeric_limits<Representation>::max() -
123 advance_several_timesteps) {
124 throw std::overflow_error(
"Too many timesteps, clock overflow imminent");
126 counter_ += advance_several_timesteps;
153 virtual ~Clock() =
default;
200 throw std::range_error(
"No negative time increment allowed");
217 throw std::overflow_error(
"Too many timesteps, clock overflow imminent");
232 throw std::range_error(
"No negative time increment allowed");
245 void reset(
const double start_time,
const bool is_output_clock)
override {
247 if (is_output_clock) {
251 reset_time = start_time;
255 reset_time,
" fm/c");
271 template <
typename T>
272 typename std::enable_if<std::is_floating_point<T>::value,
Clock&>::type
274 if (big_timestep < 0.) {
275 throw std::range_error(
"The clock cannot be turned back.");
289 if (
counter_ >= std::numeric_limits<Representation>::max() -
290 advance_several_timesteps) {
291 throw std::overflow_error(
"Too many timesteps, clock overflow imminent");
293 counter_ += advance_several_timesteps;
337 throw std::runtime_error(
"Trying to access undefined zeroth output time");
347 void reset(
double start_time,
bool)
override {
359 [start_time](
double t) {
360 if (t <= start_time) {
361 logg[
LClock].warn(
"Removing custom output time ", t,
362 " fm since it is earlier than the "
363 "starting time of the simulation");
379 #endif // SRC_INCLUDE_SMASH_CLOCK_H_
double current_time() const override
static constexpr int LClock
bool operator>(double time) const
Compares the time of the clock against a fixed time.
double timestep_duration() const override
void reset(double start_time, bool) override
reset the clock to the starting time of the simulation
Representation counter_
Internally used to count the number of time steps.
virtual double timestep_duration() const =0
std::array< einhard::Logger<>, std::tuple_size< LogArea::AreaTuple >::value > logg
An array that stores all pre-configured Logger objects.
virtual void remove_times_in_past(double start_time)=0
Remove output times before the starting time of the simulation if this is a custom clock.
void remove_times_in_past(double start_time) override
Remove all custom times before start_time.
CustomClock(std::vector< double > times)
Initialises a custom clock with explicitly given output times.
bool operator<(double time) const
Compares the time of the clock against a fixed time.
Clock tracks the time in the simulation.
virtual void reset(double start_time, const bool is_output_clock)=0
reset the clock to the starting time of the simulation
double start_time_
Starting time of the simulation.
bool operator<(const Clock &rhs) const
Compares the times between two clocks.
Clock & operator+=(Representation advance_several_timesteps)
advances the clock by an arbitrary number of ticks.
virtual double next_time() const =0
std::vector< double > custom_times_
Vector of times where output is generated.
virtual double current_time() const =0
Clock with explicitly defined time steps.
double next_time() const override
std::int64_t Representation
The type used for counting ticks/time.
Clock & operator++()
Advances the clock by one tick.