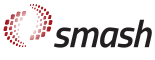 |
Version: SMASH-2.0
|
|
Go to the documentation of this file.
10 #ifndef SRC_INCLUDE_SMASH_DECAYACTIONSFINDER_H_
11 #define SRC_INCLUDE_SMASH_DECAYACTIONSFINDER_H_
44 const ParticleList &search_list,
double dt,
const double,
45 const std::vector<FourVector> &)
const override;
49 const ParticleList &,
const ParticleList &,
double,
50 const std::vector<FourVector> &)
const override {
56 const ParticleList &,
const Particles &,
double,
57 const std::vector<FourVector> &)
const override {
71 bool only_res =
false)
const override;
79 #endif // SRC_INCLUDE_SMASH_DECAYACTIONSFINDER_H_
const double res_lifetime_factor_
Multiplicative factor to be applied to resonance lifetimes.
ActionList find_actions_with_surrounding_particles(const ParticleList &, const Particles &, double, const std::vector< FourVector > &) const override
Ignore the surrounding searches for decays.
ActionList find_actions_with_neighbors(const ParticleList &, const ParticleList &, double, const std::vector< FourVector > &) const override
Ignore the neighbor searches for decays.
DecayActionsFinder(double res_lifetime_factor)
Initialize the finder.
ActionList find_actions_in_cell(const ParticleList &search_list, double dt, const double, const std::vector< FourVector > &) const override
Check the whole particle list for decays.
ActionList find_final_actions(const Particles &search_list, bool only_res=false) const override
Force all resonances to decay at the end of the simulation.