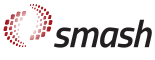 |
Version: SMASH-2.0
|
|
Go to the documentation of this file.
7 #ifndef SRC_INCLUDE_SMASH_FOURVECTOR_H_
8 #define SRC_INCLUDE_SMASH_FOURVECTOR_H_
47 :
x_({y0, y1, y2, y3}) {}
56 :
x_({y0, vec.
x1(), vec.
x2(), vec.
x3()}) {}
70 double inline x0()
const;
72 void inline set_x0(
double t);
74 double inline x1()
const;
76 void inline set_x1(
double x);
78 double inline x2()
const;
80 void inline set_x2(
double y);
82 double inline x3()
const;
84 void inline set_x3(
double z);
109 double inline sqr()
const;
118 double inline abs()
const;
125 double inline sqr3()
const;
132 double inline abs3()
const;
138 double inline tau()
const;
144 double inline eta()
const;
298 std::array<double, 4>
x_;
327 return !(*
this == a);
331 return (
x_[0] < a.
x_[0]) && (
x_[1] < a.
x_[1]) && (
x_[2] < a.
x_[2]) &&
351 this->
x_[0] += a.
x_[0];
352 this->
x_[1] += a.
x_[1];
353 this->
x_[2] += a.
x_[2];
354 this->
x_[3] += a.
x_[3];
372 this->
x_[0] -= a.
x_[0];
373 this->
x_[1] -= a.
x_[1];
374 this->
x_[2] -= a.
x_[2];
375 this->
x_[3] -= a.
x_[3];
425 const double a_inv = 1.0 / a;
426 this->
x_[0] *= a_inv;
427 this->
x_[1] *= a_inv;
428 this->
x_[2] *= a_inv;
429 this->
x_[3] *= a_inv;
447 return x_[0] * a.
x_[0] -
x_[1] * a.
x_[1] -
x_[2] * a.
x_[2] -
x_[3] * a.
x_[3];
456 return std::sqrt(std::abs(this->
sqr()));
458 throw std::runtime_error(
459 "Absolute value of 4-vector could not be "
460 "determined, taking sqrt of negative value.");
469 return std::sqrt(this->
x0() * this->
x0() - this->
x3() * this->
x3());
473 return std::atanh(this->
x3() / this->
x0());
486 #endif // SRC_INCLUDE_SMASH_FOURVECTOR_H_
double Dot(const FourVector &a) const
calculate the scalar product with another four-vector
EnergyMomentumTensor operator+(EnergyMomentumTensor a, const EnergyMomentumTensor &b)
Direct addition operator.
std::array< double, 4 >::const_iterator const_iterator
iterates over the components
double & operator[](std::size_t i)
access the component at offset i.
bool operator<=(const FourVector &a) const
logical complement to FourVector::operator>(const FourVector&) const
EnergyMomentumTensor operator/(EnergyMomentumTensor a, const double b)
Direct division operator.
FourVector operator-=(const FourVector &a)
subtracts
FourVector operator/=(const double &a)
divides by
bool operator==(const FourVector &a) const
Check if all four vector components are almost equal (accuracy ).
EnergyMomentumTensor operator-(EnergyMomentumTensor a, const EnergyMomentumTensor &b)
Direct subtraction operator.
std::ostream & operator<<(std::ostream &out, const ActionPtr &action)
double sqr() const
calculate the square of the vector (which is a scalar)
std::array< double, 4 > x_
internal storage of this vector's components
FourVector(double y0, double y1, double y2, double y3)
copy constructor
const_iterator cend() const
constexpr double really_small
Numerical error tolerance.
double eta() const
calculate the space-time rapidity from the given four vector
bool operator!=(const FourVector &a) const
checks inequality (logical complement to FourVector::operator==(const FourVector&) const)
FourVector(double y0, ThreeVector vec)
construct from time-like component and a ThreeVector.
std::array< double, 4 >::iterator iterator
iterates over the components
double tau() const
calculate the proper time from the given four vector
bool operator<(const FourVector &a) const
checks if for all (all four vector components are below comparison vector)
FourVector operator*=(const double &a)
multiplies by
const_iterator end() const
ThreeVector velocity() const
Get the velocity (3-vector divided by zero component).
bool operator>(const FourVector &a) const
checks if for all (all four vector components are above comparison vector)
double abs() const
calculate the lorentz invariant absolute value
double abs3() const
calculate the absolute value of the spatial three-vector
FourVector operator+=(const FourVector &a)
adds
const_iterator cbegin() const
FourVector lorentz_boost(const ThreeVector &v) const
Returns the FourVector boosted with velocity v.
const_iterator begin() const
EnergyMomentumTensor operator*(EnergyMomentumTensor a, const double b)
Direct multiplication operator.
double sqr3() const
calculate the square of the spatial three-vector
bool operator>=(const FourVector &a) const
logical complement to FourVector::operator<(const FourVector&) const
ThreeVector threevec() const
double operator[](std::size_t i) const
const overload of the [] operator
FourVector()
default constructor nulls the fourvector components