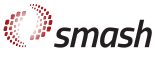 |
Version: SMASH-2.0.2
|
|
Go to the documentation of this file.
12 #include <Rivet/Rivet.hh>
13 #include <Rivet/Tools/Logging.hh>
147 const bool full_event,
const int total_N,
151 filename_(path / (name +
".yoda")),
153 rivet_confs_(out_par.subcon_for_rivet) {
154 handler_ = std::make_shared<Rivet::AnalysisHandler>();
166 const int32_t event_number,
172 logg[
LOutput].debug() <<
"Initialising Rivet" << std::endl;
177 logg[
LOutput].debug() <<
"Analysing event " << event_number << std::endl;
187 Rivet::addAnalysisLibPath(path);
188 Rivet::addAnalysisDataPath(path);
196 logg[
LOutput].info() <<
"Ignore beams? " << (ignore ?
"yes" :
"no")
202 const std::string& level) {
203 std::string fname(name);
204 if (fname.rfind(
"Rivet", 0) != 0) {
205 fname =
"Rivet." + fname;
208 auto upcase = [](
const std::string& s) {
210 std::transform(out.begin(), out.end(), out.begin(),
211 [](
char c) { return std::toupper(c); });
216 Rivet::Log::setLevel(fname, Rivet::Log::getLevelFromName(upcase(level)));
226 logg[
LOutput].debug() <<
"Setting up from configuration:\n"
231 logg[
LOutput].info() <<
"Processing paths" << std::endl;
239 logg[
LOutput].info() <<
"Processing preloads" << std::endl;
247 logg[
LOutput].info() <<
"Processing analyses" << std::endl;
279 if (wconf.has_value({
"No_Multi"})) {
284 if (wconf.has_value({
"Nominal"})) {
289 if (wconf.has_value({
"Cap"})) {
294 if (wconf.has_value({
"NLO_Smearing"})) {
299 if (wconf.has_value({
"Select"})) {
300 std::vector<std::string> sel = wconf.take({
"Select"});
304 s << (comma++ ?
"," :
"") << w;
309 if (wconf.has_value({
"Deselect"})) {
310 std::vector<std::string> sel = wconf.take({
"Deselect"});
314 s << (comma++ ?
"," :
"") << w;
318 logg[
LOutput].debug() <<
"After processing configuration:\n"
Proxy analysis_handler_proxy()
Return a proxy that temporarily disables FP exceptions.
~RivetOutput()
Destructor.
void setup()
Read configuration of Rivet from SMASH configuration.
static constexpr int LOutput
HepMC3::GenEvent event_
The event.
void at_eventend(const Particles &particles, const int32_t event_number, const EventInfo &event) override
Add the final particles information of an event to the central vertex.
std::string to_string() const
Returns a YAML string of the current tree.
bool has_value(std::initializer_list< const char * > keys) const
Returns whether there is a non-empty value behind the requested keys.
void add_path(const std::string &path)
Add a load path to the Rivet handler.
Structure to contain custom data for output.
std::array< einhard::Logger<>, std::tuple_size< LogArea::AreaTuple >::value > logg
An array that stores all pre-configured Logger objects.
Helper structure for Experiment to hold output options and parameters.
bool need_init_
Whether we need initialisation.
void at_eventend(const Particles &particles, const int32_t event_number, const EventInfo &event) override
Add the final particles information of an event to the central vertex.
void set_log_level(const std::string &name, const std::string &level)
Set log level in Rivet.
void add_analysis(const std::string &name)
Add an analysis or analyses to Rivet.
bf::path filename_
Output file.
RivetOutput(const bf::path &path, std::string name, const bool full_event, const int total_N, const int proj_N, const OutputParameters &out_par)
Create Rivet output.
Base class for output handlers that need the HepMC3 structure.
Configuration rivet_confs_
Configutations for rivet.
Value take(std::initializer_list< const char * > keys)
The default interface for SMASH to read configuration values.
void add_preload(const std::string &file)
Add preload to Rivet handler.
void set_cross_section(double xs, double xserr)
Set X-section.
std::shared_ptr< Rivet::AnalysisHandler > handler_
Rivet analysis handler.
void set_ignore_beams(bool ignore=true)
Do not insist on appropriate beams for analyses.