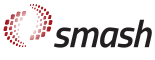 |
Version: SMASH-2.0
|
|
Go to the documentation of this file.
10 #ifndef SRC_INCLUDE_SMASH_CONFIGURATION_H_
11 #define SRC_INCLUDE_SMASH_CONFIGURATION_H_
20 #include <yaml-cpp/yaml.h>
42 static Node
encode(
const T &x) {
return Node{static_cast<std::string>(x)}; }
52 static bool decode(
const Node &node, T &x) {
53 if (!node.IsScalar()) {
56 x = static_cast<T>(node.Scalar());
471 using std::runtime_error::runtime_error;
478 using std::runtime_error::runtime_error;
486 using std::runtime_error::runtime_error;
511 if (!(
n.IsScalar() ||
n.IsSequence() ||
n.IsMap())) {
512 std::stringstream err;
513 err <<
"Configuration value for \"" << key
514 <<
"\" is missing or invalid";
515 throw std::runtime_error(err.str());
551 template <
typename T>
564 template <
typename T>
567 return node_.as<T>();
568 }
catch (YAML::TypedBadConversion<T> &e) {
570 "The value for key \"" + std::string(
key_) +
571 "\" cannot be converted to the requested type.");
580 template <
typename T>
581 operator std::vector<T>()
const {
583 return node_.as<std::vector<T>>();
584 }
catch (YAML::TypedBadConversion<T> &e) {
586 "One of the values in the sequence for key \"" + std::string(
key_) +
587 "\" failed to convert to the requested type. E.g. [1 2] is a "
588 "sequence of one string \"1 2\" and [1, 2] is a sequence of two "
589 "integers. Often there is just a comma missing in the config "
591 }
catch (YAML::TypedBadConversion<std::vector<T>> &e) {
593 "The value for key \"" + std::string(
key_) +
594 "\" cannot be converted to the requested type. A sequence was "
595 "expected but apparently not found.");
606 template <
typename T,
size_t N>
607 operator std::array<T, N>()
const {
608 const std::vector<T> vec =
operator std::vector<T>();
609 const size_t n_read = vec.size();
613 std::string(
key_) +
"\". Expected " +
617 std::to_string(n_read) +
".");
619 std::array<T, N> arr;
620 std::copy_n(vec.begin(), N, arr.begin());
632 const std::vector<std::string> v =
operator std::vector<std::string>();
634 for (
const auto &x : v) {
638 }
else if (x ==
"Elastic") {
640 }
else if (x ==
"NN_to_NR") {
642 }
else if (x ==
"NN_to_DR") {
644 }
else if (x ==
"KN_to_KN") {
646 }
else if (x ==
"KN_to_KDelta") {
648 }
else if (x ==
"Strangeness_exchange") {
650 }
else if (x ==
"NNbar") {
652 }
else if (x ==
"PiDeuteron_to_NN") {
654 }
else if (x ==
"PiDeuteron_to_pidprime") {
656 }
else if (x ==
"NDeuteron_to_Ndprime") {
660 "The value for key \"" + std::string(
key_) +
661 "\" should be \"All\", \"Elastic\", \"NN_to_NR\", \"NN_to_DR\","
662 "\"KN_to_KN\", \"KN_to_KDelta\", \"PiDeuteron_to_NN\", "
663 "\"PiDeuteron_to_pidprime\", \"NDeuteron_to_Ndprime\", "
664 "\"Strangeness_exchange\" or "
665 "\"NNbar\", or any combination of these.");
679 const std::vector<std::string> v =
operator std::vector<std::string>();
681 for (
const auto &x : v) {
685 }
else if (x ==
"Meson_3to1") {
687 }
else if (x ==
"Deuteron_3to2") {
691 "The value for key \"" + std::string(
key_) +
692 "\" should be \"All\", \"Meson_3to1\" or "
693 "\"Deuteron_3to2\", or any combination of these.");
706 operator std::set<ThermodynamicQuantity>()
const {
707 const std::vector<std::string> v =
operator std::vector<std::string>();
708 std::set<ThermodynamicQuantity> s;
709 for (
const auto &x : v) {
710 if (x ==
"rho_eckart") {
712 }
else if (x ==
"tmn") {
714 }
else if (x ==
"tmn_landau") {
716 }
else if (x ==
"landau_velocity") {
718 }
else if (x ==
"j_QBS") {
722 "The value for key \"" + std::string(
key_) +
723 "\" should be \"rho_eckart\", \"tmn\""
724 ", \"tmn_landau\", \"landau_velocity\" or \"j_QBS\".");
738 const std::string s =
operator std::string();
739 if (s ==
"center of velocity") {
742 if (s ==
"center of mass") {
745 if (s ==
"fixed target") {
749 "The value for key \"" + std::string(
key_) +
750 "\" should be \"center of velocity\" or \"center of mass\" "
751 "or \"fixed target\".");
762 const std::string s =
operator std::string();
773 "The value for key \"" + std::string(
key_) +
774 "\" should be \"off\" or \"on\" or \"frozen\".");
785 const std::string s =
operator std::string();
792 if (s ==
"baryonic isospin") {
798 if (s ==
"total isospin") {
806 "\" should be \"hadron\" or \"baryon\" "
807 "or \"baryonic isospin\" or \"pion\" "
819 const std::string s =
operator std::string();
820 if (s ==
"NoExpansion") {
823 if (s ==
"MasslessFRW") {
826 if (s ==
"MassiveFRW") {
829 if (s ==
"Exponential") {
833 "The value for key \"" + std::string(
key_) +
834 "\" should be \"NoExpansion\", \"MasslessFRW\"," +
835 "\"MassiveFRW\" or \"Exponential\".");
846 const std::string s =
operator std::string();
855 "\" should be \"None\" or \"Fixed\".");
866 const std::string s =
operator std::string();
867 if (s ==
"thermal momenta") {
870 if (s ==
"thermal momenta quantum") {
873 if (s ==
"peaked momenta") {
877 "The value for key \"" + std::string(
key_) +
878 "\" should be \"thermal momenta\", \"thermal momenta quantum\", " +
879 "or \"peaked momenta\".");
890 const std::string s =
operator std::string();
891 if (s ==
"thermal momenta") {
894 if (s ==
"thermal momenta quantum") {
906 if (s ==
"IC_Massive") {
910 "The value for key \"" + std::string(
key_) +
911 "\" should be \"thermal momenta\", \"thermal momenta quantum\", " +
912 "\"IC_ES\", \"IC_1M\", \"IC_2M\" or" +
"\"IC_Massive\".");
923 const std::string s =
operator std::string();
924 if (s ==
"no annihilation") {
927 if (s ==
"resonances") {
930 if (s ==
"strings") {
934 "The value for key \"" + std::string(
key_) +
"\" should be " +
935 "\"no annihilation\", \"detailed balance\", or \"strings\".");
946 const std::string s =
operator std::string();
947 if (s ==
"quadratic") {
953 if (s ==
"uniform") {
957 "The value for key \"" + std::string(
key_) +
958 "\" should be \"quadratic\", \"uniform\" or \"custom\".");
969 const std::string s =
operator std::string();
970 if (s ==
"mode sampling") {
973 if (s ==
"biased BF") {
976 if (s ==
"unbiased BF") {
980 "The value for key \"" + std::string(
key_) +
981 "\" should be \"mode sampling\", \"biased BF\" or \"unbiased BF\".");
992 const std::string s =
operator std::string();
993 if (s ==
"Geometric") {
996 if (s ==
"Stochastic") {
999 if (s ==
"Covariant") {
1003 "The value for key \"" + std::string(
key_) +
"\" should be " +
1004 "\"Geometric\", \"Stochastic\" " +
"or \"Covariant\".");
1015 const std::string s =
operator std::string();
1022 if (s ==
"IfNotEmpty") {
1026 std::string(
key_) +
"\" should be " +
1027 "\"Yes\", \"No\" or \"IfNotEmpty\".");
1045 explicit Configuration(
const bf::path &path,
const bf::path &filename);
1105 Value
take(std::initializer_list<const char *> keys);
1108 template <
typename T>
1109 T
take(std::initializer_list<const char *> keys, T default_value) {
1113 return default_value;
1130 Value
read(std::initializer_list<const char *> keys)
const;
1133 template <
typename T>
1134 T
read(std::initializer_list<const char *> keys, T default_value) {
1138 return default_value;
1162 template <
typename T>
1174 template <
typename T>
1185 std::initializer_list<const char *> keys)
const;
1190 bool has_value(std::initializer_list<const char *> keys)
const;
1220 #endif // SRC_INCLUDE_SMASH_CONFIGURATION_H_
static Node encode(const T &x)
Serialization: Converts x (of any type) to a YAML::Node.
Sample from areal / quadratic distribution.
std::vector< std::string > list_upmost_nodes()
Lists all YAML::Nodes from the configuration setup.
TimeStepMode
The time step mode.
const YAML::Node node_
a YAML leaf node
OutputOnlyFinal
Whether and when only final state particles should be printed.
Convert from YAML::Node to SMASH-readable (C++) format and vice versa.
YAML::Node root_node_
the general_config.yaml contents - fully parsed
Value read(std::initializer_list< const char * > keys) const
Additional interface for SMASH to read configuration values without removing them.
Use string fragmentation.
std::string to_string() const
Returns a YAML string of the current tree.
Value(const YAML::Node &n, const char *key)
Constructs the Value wrapper from a YAML::Node.
std::string unused_values_report() const
Returns a string listing the key/value pairs that have not been taken yet.
bool has_value(std::initializer_list< const char * > keys) const
Returns whether there is a non-empty value behind the requested keys.
BoxInitialCondition
Initial condition for a particle in a box.
T read(std::initializer_list< const char * > keys, T default_value)
Use fermi motion without potentials.
std::bitset< 10 > ReactionsBitSet
Container for the 2 to 2 reactions in the code.
Configuration(const YAML::Node &node)
Creates a subobject that has its root node at the given node.
Sample from custom, user-defined distribution.
Interface to the SMASH configuration files.
std::bitset< 2 > MultiParticleReactionsBitSet
Container for the 2 to 2 reactions in the code.
ThermalizationAlgorithm
Defines the algorithm used for the forced thermalization.
Print only final-state particles, and those only if the event is not empty.
Configuration(const bf::path &path)
Reads config.yaml from the specified path.
Sampling
Possible methods of impact parameter sampling.
Configuration(const char *yaml)
CollisionCriterion
Criteria used to check collisions.
Return type of Configuration::take that automatically determines the target type.
Print only final-state particles.
SphereInitialCondition
Initial condition for a particle in a sphere.
Use intermediate Resonances.
CalculationFrame
The calculation frame.
DensityType
Allows to choose which kind of density to calculate.
Sample from uniform distribution.
const char *const key_
The key to be interpreted.
void merge_yaml(const std::string &yaml)
Merge the configuration in yaml into the existing tree.
Use fermi motion in combination with potentials.
T convert_for(const T &) const
Convert the value to the type of the supplied argument.
elastic scattering: particles remain the same, only momenta change
Value take(std::initializer_list< const char * > keys)
The default interface for SMASH to read configuration values.
bool has_value_including_empty(std::initializer_list< const char * > keys) const
Returns if there is a (maybe empty) value behind the requested keys.
Configuration & operator=(T &&value)
Assignment overwrites the value of the current YAML node.
NNbarTreatment
Treatment of N Nbar Annihilation.
Value & operator=(const Value &)=delete
If you want to copy this you're doing it wrong.
Configuration & operator=(const Configuration &)=default
If you want to copy this you're doing it wrong.
(Default) geometric criterion.
ExpansionMode
Defines properties of expansion for the metric (e.g.
void remove_all_but(const std::string &key)
Removes all entries in the map except for key.
Configuration operator[](T &&key)
Access to the YAML::Node behind the requested keys.
static bool decode(const Node &node, T &x)
Deserialization: Converts a YAML::Node to any SMASH-readable data type and returns whether or not thi...
FermiMotion
Option to use Fermi Motion.
T take(std::initializer_list< const char * > keys, T default_value)