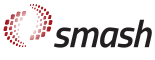 |
Version: SMASH-2.0
|
|
Go to the documentation of this file.
9 #ifndef SRC_INCLUDE_SMASH_DENSITY_H_
10 #define SRC_INCLUDE_SMASH_DENSITY_H_
29 static constexpr
int LDensity = LogArea::Density::id;
78 const double tmp = two_sigma_sqr * M_PI;
79 return tmp * std::sqrt(tmp);
100 const double x = rcut_in_sigma / std::sqrt(2.0);
101 return -2.0 / std::sqrt(M_PI) * x * std::exp(-x * x) + std::erf(x);
119 :
sig_(par.gaussian_sigma),
120 r_cut_(par.gauss_cutoff_in_sigma * par.gaussian_sigma),
121 ntest_(par.testparticles) {
123 const double two_sig_sqr = 2 *
sig_ *
sig_;
126 const double corr_factor =
227 std::tuple<double, FourVector, ThreeVector, ThreeVector, ThreeVector>
230 bool compute_gradient,
bool smearing);
232 std::tuple<double, FourVector, ThreeVector, ThreeVector, ThreeVector>
235 bool compute_gradient,
bool smearing);
280 if (FactorTimesSf > 0.0) {
281 jmu_pos_ += PartFourVelocity * FactorTimesSf;
283 jmu_neg_ += PartFourVelocity * FactorTimesSf;
302 for (
int k = 1; k <= 3; k++) {
303 djmu_dx_[k] += factor * PartFourVelocity * sf_grad[k - 1];
305 factor * PartFourVelocity * sf_grad[k - 1] * part.
velocity()[k - 1];
326 double density(
const double norm_factor = 1.0) {
341 j_rot *= norm_factor;
353 for (
int i = 1; i < 4; i++) {
354 rho_grad[i - 1] =
djmu_dx_[i].x0() * norm_factor;
366 return djmu_dx_[0].threevec() * norm_factor;
401 template <
typename T>
405 const bool compute_gradient =
false) {
407 if (lat ==
nullptr || lat->
when_update() != update) {
412 for (
const auto &part : particles) {
413 const double dens_factor =
density_factor(part.type(), dens_type);
418 const double m =
p.abs();
420 logg[
LDensity].warn(
"Gaussian smearing is undefined for momentum ",
p);
423 const double m_inv = 1.0 / m;
425 const ThreeVector pos = part.position().threevec();
427 pos, par.
r_cut(), [&](T &node,
int ix,
int iy,
int iz) {
432 node.add_particle(part, sf.first * norm_factor * dens_factor);
434 if (compute_gradient) {
435 node.add_particle_for_derivatives(part, dens_factor,
436 sf.second * norm_factor);
444 #endif // SRC_INCLUDE_SMASH_DENSITY_H_
LatticeUpdate
Enumerator option for lattice updates.
double two_sig_sqr_inv_
[fm ]
void set_x2(double y)
set second component
double two_sig_sqr_inv() const
A class to pre-calculate and store parameters relevant for density calculation.
std::array< FourVector, 4 > djmu_dx_
void set_x3(double z)
set third component
double smearing_factor_rcut_correction(const double rcut_in_sigma)
Gaussians used for smearing are cut at radius for calculation speed-up.
#define unlikely(x)
Tell the branch predictor that this expression is likely false.
FourVector jmu_neg_
Four-current density of the negatively charged particle.
void add_particle(const ParticleData &part, double FactorTimesSf)
Adds particle to 4-current: .
void iterate_in_radius(const ThreeVector &point, const double r_cut, F &&func)
Iterates only nodes, whose cell centers lie not further than r_cut in x, y, z directions from the giv...
std::ostream & operator<<(std::ostream &out, const ActionPtr &action)
ThreeVector rot_j(const double norm_factor=1.0)
Compute curl of the current on the local lattice.
double norm_factor_sf_
Normalization for smearing factor.
double r_cut_sqr_
Squared cut-off radius [fm ].
FourVector jmu_net() const
std::pair< double, ThreeVector > unnormalized_smearing_factor(const ThreeVector &r, const FourVector &p, const double m_inv, const DensityParameters &dens_par, const bool compute_gradient=false)
Implements gaussian smearing for any quantity.
std::array< einhard::Logger<>, std::tuple_size< LogArea::AreaTuple >::value > logg
An array that stores all pre-configured Logger objects.
A class for time-efficient (time-memory trade-off) calculation of density on the lattice.
constexpr double really_small
Numerical error tolerance.
const int ntest_
Testparticle number.
double density_factor(const ParticleType &type, DensityType dens_type)
Get the factor that determines how much a particle contributes to the density type that is computed.
ThreeVector cell_center(int ix, int iy, int iz) const
Find the coordinates of a given cell.
double smearing_factor_norm(const double two_sigma_sqr)
Norm of the Gaussian smearing function.
static constexpr int LDensity
void add_particle_for_derivatives(const ParticleData &part, double factor, ThreeVector sf_grad)
Adds particle to the time and spatial derivatives of the 4-current.
void update_lattice(RectangularLattice< T > *lat, const LatticeUpdate update, const DensityType dens_type, const DensityParameters &par, const Particles &particles, const bool compute_gradient=false)
Updates the contents on the lattice.
A container class to hold all the arrays on the lattice and access them.
const double r_cut_
Cut-off radius [fm].
std::tuple< double, FourVector, ThreeVector, ThreeVector, ThreeVector > current_eckart(const ThreeVector &r, const ParticleList &plist, const DensityParameters &par, DensityType dens_type, bool compute_gradient, bool smearing)
Calculates Eckart rest frame density and 4-current of a given density type and optionally the gradien...
void set_x1(double x)
set first component
FourVector jmu_pos_
Four-current density of the positively charged particle.
double gauss_cutoff_in_sigma
Distance at which gaussian is cut, i.e. set to zero, IN SIGMA (not fm)
double abs() const
calculate the lorentz invariant absolute value
DensityOnLattice()
Default constructor.
double density(const double norm_factor=1.0)
Compute the net Eckart density on the local lattice.
DensityType
Allows to choose which kind of density to calculate.
DensityParameters(const ExperimentParameters &par)
Constructor of DensityParameters.
ThreeVector dj_dt(const double norm_factor=1.0)
Compute time derivative of the current density on the local lattice.
double norm_factor_sf() const
ThreeVector grad_rho(const double norm_factor=1.0)
Compute gradient of the density on the local lattice.
Helper structure for Experiment.
LatticeUpdate when_update() const
ThreeVector velocity() const
Get the velocity 3-vector.
void reset()
Sets all values on lattice to zeros.
RectangularLattice< DensityOnLattice > DensityLattice
Conveniency typedef for lattice of density.
const double sig_
Gaussian smearing width [fm].