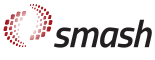 |
Version: SMASH-2.0
|
|
Go to the documentation of this file.
10 #ifndef SRC_INCLUDE_SMASH_LATTICE_H_
11 #define SRC_INCLUDE_SMASH_LATTICE_H_
25 static constexpr
int LLattice = LogArea::Lattice::id;
67 const std::array<int, 3>&
n,
68 const std::array<double, 3>& orig,
bool per,
78 "Rectangular lattice created: sizes[fm] = (",
lattice_sizes_[0],
",",
80 ",",
n_cells_[1],
",",
n_cells_[2],
"), origin = (",
origin_[0],
",",
85 throw std::invalid_argument(
86 "Lattice sizes should be positive, "
87 "lattice dimensions should be > 0.");
170 using iterator =
typename std::vector<T>::iterator;
196 T&
node(
int ix,
int iy,
int iz) {
227 value =
node(ix, iy, iz);
242 template <
typename F>
244 const std::array<int, 3>& upper_bounds, F&& func) {
246 "Iterating sublattice with lower bound index (", lower_bounds[0],
",",
247 lower_bounds[1],
",", lower_bounds[2],
"), upper bound index (",
248 upper_bounds[0],
",", upper_bounds[1],
",", upper_bounds[2],
")");
251 for (
int iz = lower_bounds[2]; iz < upper_bounds[2]; iz++) {
253 for (
int iy = lower_bounds[1]; iy < upper_bounds[1]; iy++) {
256 for (
int ix = lower_bounds[0]; ix < upper_bounds[0]; ix++) {
263 for (
int iz = lower_bounds[2]; iz < upper_bounds[2]; iz++) {
264 const int z_offset = iz *
n_cells_[1];
265 for (
int iy = lower_bounds[1]; iy < upper_bounds[1]; iy++) {
266 const int y_offset =
n_cells_[0] * (iy + z_offset);
267 for (
int ix = lower_bounds[0]; ix < upper_bounds[0]; ix++) {
268 func(
lattice_[ix + y_offset], ix, iy, iz);
287 template <
typename F>
290 std::array<int, 3> l_bounds, u_bounds;
295 for (
int i = 0; i < 3; i++) {
303 for (
int i = 0; i < 3; i++) {
304 if (l_bounds[i] < 0) {
310 if (l_bounds[i] >
n_cells_[i] || u_bounds[i] < 0) {
326 template <
typename L>
328 return n_cells_[0] == lat->dimensions()[0] &&
329 n_cells_[1] == lat->dimensions()[1] &&
330 n_cells_[2] == lat->dimensions()[2] &&
373 return (i + (
n << 8)) %
n;
379 #endif // SRC_INCLUDE_SMASH_LATTICE_H_
LatticeUpdate
Enumerator option for lattice updates.
RectangularLattice(const std::array< double, 3 > &l, const std::array< int, 3 > &n, const std::array< double, 3 > &orig, bool per, const LatticeUpdate upd)
Rectangular lattice constructor.
bool out_of_bounds(int ix, int iy, int iz) const
Checks if 3D index is out of lattice bounds.
typename std::vector< smash::EnergyMomentumTensor >::const_iterator const_iterator
Const interator of lattice.
void iterate_in_radius(const ThreeVector &point, const double r_cut, F &&func)
Iterates only nodes, whose cell centers lie not further than r_cut in x, y, z directions from the giv...
const std::array< int, 3 > n_cells_
Number of cells in x,y,z directions.
bool identical_to_lattice(const L *lat) const
Checks if lattices of possibly different types have identical structure.
int positive_modulo(int i, int n) const
Returns division modulo, which is always between 0 and n-1 in is not suitable, because it returns res...
const std::array< double, 3 > lattice_sizes_
Lattice sizes in x, y, z directions.
std::array< einhard::Logger<>, std::tuple_size< LogArea::AreaTuple >::value > logg
An array that stores all pre-configured Logger objects.
const_iterator end() const
constexpr double really_small
Numerical error tolerance.
const T & operator[](std::size_t i) const
ThreeVector cell_center(int ix, int iy, int iz) const
Find the coordinates of a given cell.
A container class to hold all the arrays on the lattice and access them.
const std::array< double, 3 > cell_sizes_
Cell sizes in x, y, z directions.
const LatticeUpdate when_update_
When the lattice should be recalculated.
ThreeVector cell_center(int index) const
Find the coordinate of cell center given the 1d index of the cell.
std::vector< T > lattice_
The lattice itself, array containing physical quantities.
const_iterator begin() const
RectangularLattice(RectangularLattice< T > const &rl)
Copy-constructor.
T & node(int ix, int iy, int iz)
Take the value of a cell given its 3-D indices.
const std::array< double, 3 > & lattice_sizes() const
bool value_at(const ThreeVector &r, T &value)
Interpolates lattice quantity to coordinate r.
const std::array< double, 3 > & cell_sizes() const
T & operator[](std::size_t i)
const std::array< int, 3 > & dimensions() const
LatticeUpdate when_update() const
const bool periodic_
Whether the lattice is periodic.
typename std::vector< smash::EnergyMomentumTensor >::iterator iterator
Iterator of lattice.
static constexpr int LLattice
const std::array< double, 3 > origin_
Coordinates of the left down nearer corner.
const std::array< double, 3 > & origin() const
void reset()
Sets all values on lattice to zeros.
void iterate_sublattice(const std::array< int, 3 > &lower_bounds, const std::array< int, 3 > &upper_bounds, F &&func)
A sub-lattice iterator, which iterates in a 3D-structured manner and calls a function on every cell.