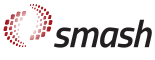 |
Version: SMASH-2.0
|
|
#include <lattice.h>
template<typename T>
class smash::RectangularLattice< T >
A container class to hold all the arrays on the lattice and access them.
- Template Parameters
-
T | The type of the contained values. |
Definition at line 47 of file lattice.h.
|
| RectangularLattice (const std::array< double, 3 > &l, const std::array< int, 3 > &n, const std::array< double, 3 > &orig, bool per, const LatticeUpdate upd) |
| Rectangular lattice constructor. More...
|
|
| RectangularLattice (RectangularLattice< T > const &rl) |
| Copy-constructor. More...
|
|
void | reset () |
| Sets all values on lattice to zeros. More...
|
|
bool | out_of_bounds (int ix, int iy, int iz) const |
| Checks if 3D index is out of lattice bounds. More...
|
|
ThreeVector | cell_center (int ix, int iy, int iz) const |
| Find the coordinates of a given cell. More...
|
|
ThreeVector | cell_center (int index) const |
| Find the coordinate of cell center given the 1d index of the cell. More...
|
|
const std::array< double, 3 > & | lattice_sizes () const |
|
const std::array< int, 3 > & | dimensions () const |
|
const std::array< double, 3 > & | cell_sizes () const |
|
const std::array< double, 3 > & | origin () const |
|
bool | periodic () const |
|
LatticeUpdate | when_update () const |
|
iterator | begin () |
|
const_iterator | begin () const |
|
iterator | end () |
|
const_iterator | end () const |
|
T & | operator[] (std::size_t i) |
|
const T & | operator[] (std::size_t i) const |
|
std::size_t | size () const |
|
T & | node (int ix, int iy, int iz) |
| Take the value of a cell given its 3-D indices. More...
|
|
bool | value_at (const ThreeVector &r, T &value) |
| Interpolates lattice quantity to coordinate r. More...
|
|
template<typename F > |
void | iterate_sublattice (const std::array< int, 3 > &lower_bounds, const std::array< int, 3 > &upper_bounds, F &&func) |
| A sub-lattice iterator, which iterates in a 3D-structured manner and calls a function on every cell. More...
|
|
template<typename F > |
void | iterate_in_radius (const ThreeVector &point, const double r_cut, F &&func) |
| Iterates only nodes, whose cell centers lie not further than r_cut in x, y, z directions from the given point and applies a function to each node. More...
|
|
template<typename L > |
bool | identical_to_lattice (const L *lat) const |
| Checks if lattices of possibly different types have identical structure. More...
|
|
|
std::vector< T > | lattice_ |
| The lattice itself, array containing physical quantities. More...
|
|
const std::array< double, 3 > | lattice_sizes_ |
| Lattice sizes in x, y, z directions. More...
|
|
const std::array< int, 3 > | n_cells_ |
| Number of cells in x,y,z directions. More...
|
|
const std::array< double, 3 > | cell_sizes_ |
| Cell sizes in x, y, z directions. More...
|
|
const std::array< double, 3 > | origin_ |
| Coordinates of the left down nearer corner. More...
|
|
const bool | periodic_ |
| Whether the lattice is periodic. More...
|
|
const LatticeUpdate | when_update_ |
| When the lattice should be recalculated. More...
|
|
|
int | positive_modulo (int i, int n) const |
| Returns division modulo, which is always between 0 and n-1 in is not suitable, because it returns results from -(n-1) to n-1. More...
|
|
◆ iterator
Iterator of lattice.
Definition at line 170 of file lattice.h.
◆ const_iterator
Const interator of lattice.
Definition at line 172 of file lattice.h.
◆ RectangularLattice() [1/2]
Rectangular lattice constructor.
- Parameters
-
[in] | l | 3-dimensional array (lx,ly,lz) indicates the size of the lattice in x, y, z directions respectively [fm]. |
[in] | n | 3-dimensional array (nx,ny,nz) indicates the number of cells of the lattice in x, y, z directions respectively. Each cell in the lattice is labeled by three integers i, j, k where \(i\in[0, nx-1]\), \(j\in[0, ny-1]\), \(k\in[0, nz-1]\). The sizes of each cell are given by lx/nx, ly/ny, lz/nz in x,y,z directions respectively. |
[in] | orig | A 3-dimensional array indicating the coordinates of the origin [fm]. |
[in] | per | Boolean indicating whether a periodic boundary condition is applied. |
[in] | upd | Enum indicating how frequently the lattice is updated. |
Definition at line 66 of file lattice.h.
78 "Rectangular lattice created: sizes[fm] = (",
lattice_sizes_[0],
",",
80 ",",
n_cells_[1],
",",
n_cells_[2],
"), origin = (",
origin_[0],
",",
85 throw std::invalid_argument(
86 "Lattice sizes should be positive, "
87 "lattice dimensions should be > 0.");
◆ RectangularLattice() [2/2]
Copy-constructor.
Definition at line 92 of file lattice.h.
◆ reset()
Sets all values on lattice to zeros.
Definition at line 102 of file lattice.h.
◆ out_of_bounds()
Checks if 3D index is out of lattice bounds.
- Parameters
-
[in] | ix | The index of the cell in x direction. |
[in] | iy | The index of the cell in y direction. |
[in] | iz | The index of the cell in z direction. |
- Returns
- Whether the cell is out of the lattice.
Definition at line 112 of file lattice.h.
◆ cell_center() [1/2]
Find the coordinates of a given cell.
- Parameters
-
[in] | ix | The index of the cell in x direction. |
[in] | iy | The index of the cell in y direction. |
[in] | iz | The index of the cell in z direction. |
- Returns
- Coordinates of the center of the given cell [fm].
Definition at line 129 of file lattice.h.
◆ cell_center() [2/2]
Find the coordinate of cell center given the 1d index of the cell.
- Parameters
-
[in] | index | 1-dimensional index of the given cell. It can be related to a 3-dimensional one by index = ix + nx (iy + iz * ny). |
- Returns
- Coordinates of the center of the given cell [fm].
Definition at line 143 of file lattice.h.
◆ lattice_sizes()
- Returns
- Lengths of the lattice in x, y, z directions.
Definition at line 152 of file lattice.h.
◆ dimensions()
- Returns
- Number of cells in x, y, z directions.
Definition at line 155 of file lattice.h.
◆ cell_sizes()
- Returns
- Lengths of one cell in x, y, z directions.
Definition at line 158 of file lattice.h.
◆ origin()
- Returns
- Lattice origin: left, down, near corner coordinates.
Definition at line 161 of file lattice.h.
◆ periodic()
- Returns
- If lattice is periodic or not.
Definition at line 164 of file lattice.h.
◆ when_update()
- Returns
- The enum, which tells at which time lattice needs to be updated.
Definition at line 167 of file lattice.h.
◆ begin() [1/2]
- Returns
- First element of lattice.
Definition at line 174 of file lattice.h.
◆ begin() [2/2]
- Returns
- First element of lattice (const).
Definition at line 176 of file lattice.h.
◆ end() [1/2]
- Returns
- Last element of lattice.
Definition at line 178 of file lattice.h.
◆ end() [2/2]
- Returns
- Last element of lattice (const).
Definition at line 180 of file lattice.h.
◆ operator[]() [1/2]
- Returns
- ith element of lattice.
Definition at line 182 of file lattice.h.
◆ operator[]() [2/2]
- Returns
- ith element of lattice (const).
Definition at line 184 of file lattice.h.
◆ size()
- Returns
- Size of lattice.
Definition at line 186 of file lattice.h.
◆ node()
Take the value of a cell given its 3-D indices.
- Parameters
-
[in] | ix | The index of the cell in x direction. |
[in] | iy | The index of the cell in y direction. |
[in] | iz | The index of the cell in z direction. |
- Returns
- Physical quantity evaluated at the cell center.
Definition at line 196 of file lattice.h.
◆ value_at()
Interpolates lattice quantity to coordinate r.
Result is stored in the value variable. Returns true if coordinate r is on the lattice, false if out of the lattice. In the latter case, the value is set to the default value (usually 0).
- Parameters
-
[in] | r | Position where the physical quantity would be evaluated. |
[out] | value | Physical quantity evaluated at the nearest cell to the given position. |
- Returns
- Boolean indicates whether the position r is located inside the lattice.
- Todo:
- (oliiny): maybe 1-order interpolation instead of 0-order?
Definition at line 219 of file lattice.h.
227 value =
node(ix, iy, iz);
◆ iterate_sublattice()
template<typename T>
template<typename F >
void smash::RectangularLattice< T >::iterate_sublattice |
( |
const std::array< int, 3 > & |
lower_bounds, |
|
|
const std::array< int, 3 > & |
upper_bounds, |
|
|
F && |
func |
|
) |
| |
|
inline |
A sub-lattice iterator, which iterates in a 3D-structured manner and calls a function on every cell.
- Template Parameters
-
F | Type of the function. Arguments are the current node and the 3 integer indices of the cell. |
- Parameters
-
[in] | lower_bounds | Starting numbers for iterating ix, iy, iz. |
[in] | upper_bounds | Ending numbers for iterating ix, iy, iz. |
[in] | func | Function acting on the cells (such as taking value). |
Definition at line 243 of file lattice.h.
246 "Iterating sublattice with lower bound index (", lower_bounds[0],
",",
247 lower_bounds[1],
",", lower_bounds[2],
"), upper bound index (",
248 upper_bounds[0],
",", upper_bounds[1],
",", upper_bounds[2],
")");
251 for (
int iz = lower_bounds[2]; iz < upper_bounds[2]; iz++) {
253 for (
int iy = lower_bounds[1]; iy < upper_bounds[1]; iy++) {
256 for (
int ix = lower_bounds[0]; ix < upper_bounds[0]; ix++) {
263 for (
int iz = lower_bounds[2]; iz < upper_bounds[2]; iz++) {
264 const int z_offset = iz *
n_cells_[1];
265 for (
int iy = lower_bounds[1]; iy < upper_bounds[1]; iy++) {
266 const int y_offset =
n_cells_[0] * (iy + z_offset);
267 for (
int ix = lower_bounds[0]; ix < upper_bounds[0]; ix++) {
268 func(
lattice_[ix + y_offset], ix, iy, iz);
◆ iterate_in_radius()
template<typename T>
template<typename F >
Iterates only nodes, whose cell centers lie not further than r_cut in x, y, z directions from the given point and applies a function to each node.
Useful for adding quantities from one particle to the lattice.
- Template Parameters
-
F | Type of the function. Arguments are the current node and the 3 integer indices of the cell. |
- Parameters
-
[in] | point | Position, usually the position of particle [fm]. |
[in] | r_cut | Maximum distance from the cell center to the given position. [fm] |
[in] | func | Function acting on the cells (such as taking value). |
Definition at line 288 of file lattice.h.
290 std::array<int, 3> l_bounds, u_bounds;
295 for (
int i = 0; i < 3; i++) {
303 for (
int i = 0; i < 3; i++) {
304 if (l_bounds[i] < 0) {
310 if (l_bounds[i] >
n_cells_[i] || u_bounds[i] < 0) {
◆ identical_to_lattice()
template<typename T>
template<typename L >
Checks if lattices of possibly different types have identical structure.
- Template Parameters
-
L | Type of the other lattice. |
- Parameters
-
[in] | lat | The other lattice being compared with the current one |
- Returns
- Whether the two lattices have the same sizes, cell numbers, origins, and boundary conditions.
Definition at line 327 of file lattice.h.
328 return n_cells_[0] == lat->dimensions()[0] &&
329 n_cells_[1] == lat->dimensions()[1] &&
330 n_cells_[2] == lat->dimensions()[2] &&
◆ positive_modulo()
Returns division modulo, which is always between 0 and n-1 in is not suitable, because it returns results from -(n-1) to n-1.
- Parameters
-
[in] | i | Dividend. |
[in] | n | Divisor. |
- Returns
- Positive remainder.
Definition at line 368 of file lattice.h.
373 return (i + (
n << 8)) %
n;
◆ lattice_
The lattice itself, array containing physical quantities.
Definition at line 345 of file lattice.h.
◆ lattice_sizes_
Lattice sizes in x, y, z directions.
Definition at line 347 of file lattice.h.
◆ n_cells_
Number of cells in x,y,z directions.
Definition at line 349 of file lattice.h.
◆ cell_sizes_
Cell sizes in x, y, z directions.
Definition at line 351 of file lattice.h.
◆ origin_
Coordinates of the left down nearer corner.
Definition at line 353 of file lattice.h.
◆ periodic_
Whether the lattice is periodic.
Definition at line 355 of file lattice.h.
◆ when_update_
When the lattice should be recalculated.
Definition at line 357 of file lattice.h.
The documentation for this class was generated from the following file:
bool out_of_bounds(int ix, int iy, int iz) const
Checks if 3D index is out of lattice bounds.
const std::array< int, 3 > n_cells_
Number of cells in x,y,z directions.
int positive_modulo(int i, int n) const
Returns division modulo, which is always between 0 and n-1 in is not suitable, because it returns res...
const std::array< double, 3 > lattice_sizes_
Lattice sizes in x, y, z directions.
std::array< einhard::Logger<>, std::tuple_size< LogArea::AreaTuple >::value > logg
An array that stores all pre-configured Logger objects.
constexpr double really_small
Numerical error tolerance.
ThreeVector cell_center(int ix, int iy, int iz) const
Find the coordinates of a given cell.
const std::array< double, 3 > cell_sizes_
Cell sizes in x, y, z directions.
const LatticeUpdate when_update_
When the lattice should be recalculated.
std::vector< T > lattice_
The lattice itself, array containing physical quantities.
T & node(int ix, int iy, int iz)
Take the value of a cell given its 3-D indices.
const bool periodic_
Whether the lattice is periodic.
static constexpr int LLattice
const std::array< double, 3 > origin_
Coordinates of the left down nearer corner.
void iterate_sublattice(const std::array< int, 3 > &lower_bounds, const std::array< int, 3 > &upper_bounds, F &&func)
A sub-lattice iterator, which iterates in a 3D-structured manner and calls a function on every cell.