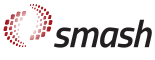 |
Version: SMASH-2.0
|
|
Go to the documentation of this file.
11 #ifndef SRC_INCLUDE_SMASH_HEPMCOUTPUT_H_
12 #define SRC_INCLUDE_SMASH_HEPMCOUTPUT_H_
21 #include <boost/filesystem.hpp>
23 #include "HepMC3/GenEvent.h"
24 #include "HepMC3/GenParticle.h"
25 #include "HepMC3/GenVertex.h"
26 #include "HepMC3/WriterAscii.h"
133 #endif // SRC_INCLUDE_SMASH_HEPMCOUTPUT_H_
const int proj_N_
Total number of nucleons in projectile, needed for converting nuclei to single particles.
const int total_N_
Total number of nucleons in projectile and target, needed for converting nuclei to single particles.
bf::path filename_unfinished_
Filename of output as long as simulation is still running.
HepMcOutput(const bf::path &path, std::string name, const OutputParameters &out_par, const int total_N, const int proj_N)
Create HepMC particle output.
void at_eventstart(const Particles &particles, const int event_number, const EventInfo &) override
Add the initial particles information of an event to the central vertex.
SMASH output to HepMC file.
Structure to contain custom data for output.
std::unique_ptr< HepMC3::WriterAscii > output_file_
Pointer to Ascii HepMC3 output file.
static const int status_code_for_beam_particles
HepMC convention: status code for target and projecticle particles.
~HepMcOutput()
Destructor renames file.
Helper structure for Experiment to hold output options and parameters.
std::unique_ptr< HepMC3::GenEvent > current_event_
HepMC3::GenEvent pointer for current event.
Abstraction of generic output.
static const int status_code_for_final_particles
HepMC convention: status code for final particles.
HepMC3::GenVertexPtr vertex_
HepMC3::GenVertex pointer for central vertex in event.
int construct_nuclear_pdg_code(int na, int nz) const
Construct nulcear pdg code for porjectile and target, see PDG chapter "Monte Carlo particle numbering...
void at_eventend(const Particles &particles, const int32_t event_number, const EventInfo &event) override
Add the final particles information of an event to the central vertex.
const bf::path filename_
Filename of output.