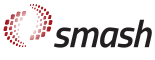 |
Version: SMASH-2.0
|
|
Go to the documentation of this file.
10 #ifndef SRC_INCLUDE_SMASH_OUTPUTINTERFACE_H_
11 #define SRC_INCLUDE_SMASH_OUTPUTINTERFACE_H_
117 const std::unique_ptr<Clock> &clock,
197 throw std::invalid_argument(
"Unknown thermodynamic quantity.");
212 return "net_baryonI3";
216 return "tot_isospin3";
220 return "strangeness";
224 throw std::invalid_argument(
"Unknown density type.");
240 #endif // SRC_INCLUDE_SMASH_OUTPUTINTERFACE_H_
OutputInterface(std::string name)
Construct output interface.
A class to pre-calculate and store parameters relevant for density calculation.
bool is_IC_output() const
Get, whether this is the IC output?
const char * to_string(const ThermodynamicQuantity tq)
Convert thermodynamic quantities to strings.
virtual ~OutputInterface()=default
int test_particles
Testparticle number, see Testparticles in General.
Structure to contain custom data for output.
bool is_photon_output() const
Get, whether this is the photon output?
double current_time
Time in fm/c.
const bool is_photon_output_
Is this the photon output?
virtual void at_eventend(const Particles &particles, const int event_number, const EventInfo &info)=0
Output launched at event end.
virtual void at_eventstart(const Particles &particles, const int event_number, const EventInfo &info)=0
Output launched at event start after initialization, when particles are generated but not yet propaga...
virtual void at_interaction(const Action &action, const double density)
Called whenever an action modified one or more particles.
double total_kinetic_energy
Sum of kinetic energies of all particles.
virtual void at_intermediate_time(const Particles &particles, const std::unique_ptr< Clock > &clock, const DensityParameters &dens_param, const EventInfo &info)
Output launched after every N'th timestep.
A container class to hold all the arrays on the lattice and access them.
Abstraction of generic output.
const bool is_dilepton_output_
Is this the dilepton output?
double total_mean_field_energy
Total energy in the mean field.
bool is_dilepton_output() const
Get, whether this is the dilepton output?
#define SMASH_UNUSED(x)
Mark as unused, silencing compiler warnings.
double total_energy
Kinetic + mean field energy.
DensityType
Allows to choose which kind of density to calculate.
bool empty_event
True if no collisions happened.
ThermodynamicQuantity
Represents thermodynamic quantities that can be printed out.
double modus_length
Box length in case of box simulation, otherwise dummy.
double impact_parameter
Impact parameter for collider modus, otherwise dummy.
The GrandCanThermalizer class implements the following functionality:
const bool is_IC_output_
Is this the IC output?
const char * to_string(const DensityType dens_type)
Convert density types to strings.
virtual void thermodynamics_output(const ThermodynamicQuantity tq, const DensityType dt, RectangularLattice< DensityOnLattice > &lattice)
Output to write thermodynamics from the lattice.
virtual void thermodynamics_output(const GrandCanThermalizer &gct)
Output to write energy-momentum tensor and related quantities from the thermalizer class.
virtual void thermodynamics_output(const ThermodynamicQuantity tq, const DensityType dt, RectangularLattice< EnergyMomentumTensor > &lattice)
Output to write energy-momentum tensor and related quantities from the lattice.