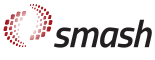 |
Version: SMASH-2.0
|
|
Go to the documentation of this file.
7 #ifndef SRC_INCLUDE_SMASH_GRANDCAN_THERMALIZER_H_
8 #define SRC_INCLUDE_SMASH_GRANDCAN_THERMALIZER_H_
96 double nb()
const {
return nb_; }
98 double ns()
const {
return ns_; }
102 double e()
const {
return e_; }
104 double p()
const {
return p_; }
108 double T()
const {
return T_; }
246 const std::array<int, 3> n_cells,
247 const std::array<double, 3> origin,
bool periodicity,
248 double e_critical,
double t_start,
double delta_t,
252 const std::array<double, 3> lat_sizes,
253 const std::array<double, 3> origin,
bool periodicity)
255 lat_sizes, conf.take({
"Cell_Number"}), origin, periodicity,
256 conf.
take({
"Critical_Edens"}), conf.take({
"Start_Time"}),
257 conf.take({
"Timestep"}),
259 conf.take({
"Microcanonical"},
false)) {}
266 const double t = clock->current_time();
269 t < t_start_ + n * period_ + clock->timestep_duration());
280 bool ignore_cells_under_threshold =
true);
338 template <
typename F>
344 const double gamma = 1.0 / std::sqrt(1.0 - cell.
v().
sqr());
347 if (condition(i->strangeness(), i->baryon_number(), i->charge())) {
369 template <
typename F>
374 double partial_sum = 0.0;
375 int index_only_thermalized = -1;
376 while (partial_sum < r) {
377 index_only_thermalized++;
378 partial_sum +=
N_in_cells_[index_only_thermalized];
383 const double gamma = 1.0 / std::sqrt(1.0 - cell.
v().
sqr());
384 const double N_in_cell =
N_in_cells_[index_only_thermalized];
390 if (!condition(i->strangeness(), i->baryon_number(), i->charge())) {
403 const double m = type_to_sample->
mass();
410 particle.
set_4momentum(m, phitheta.threevec() * momentum_radial);
456 ParticleTypePtrList res;
459 res.push_back(&ptype);
469 const int B =
eos_typelist_[typelist_index]->baryon_number();
493 std::unique_ptr<RectangularLattice<ThermLatticeNode>>
lat_;
539 #endif // SRC_INCLUDE_SMASH_GRANDCAN_THERMALIZER_H_
Class to handle the equation of state (EoS) of the hadron gas, consisting of all hadrons included in ...
double p() const
Get pressure in the rest frame.
void sample_multinomial(HadronClass particle_class, int N)
The sample_multinomial function samples integer numbers n_i distributed according to the multinomial ...
void add_particle_for_derivatives(const ParticleData &, double, ThreeVector)
dummy function for update_lattice
GrandCanThermalizer(const std::array< double, 3 > lat_sizes, const std::array< int, 3 > n_cells, const std::array< double, 3 > origin, bool periodicity, double e_critical, double t_start, double delta_t, ThermalizationAlgorithm algo, bool BF_microcanonical)
Default constructor for the GranCanThermalizer to allocate the lattice.
double mub_
Net baryon chemical potential.
A class to pre-calculate and store parameters relevant for density calculation.
double ns() const
Get net strangeness density of the cell in the computational frame.
double nq() const
Get net charge density of the cell in the computational frame.
Mesons with strangeness S < 0.
ThermLatticeNode()
Default constructor of thermal quantities on the lattice returning thermodynamic quantities in comput...
const double t_start_
Starting time of the simulation.
FourVector Tmu0_
Four-momentum flow of the cell.
ParticleTypePtrList list_eos_particles() const
Extracts the particles in the hadron gas equation of state from the complete list of particle types i...
ThreeVector v() const
Get 3-velocity of the rest frame.
ParticleList particles_to_remove() const
List of particles to be removed from the simulation.
std::ostream & operator<<(std::ostream &out, const ActionPtr &action)
const size_t N_sorts_
Number of different species to be sampled.
Non-strange mesons (S = 0) with electric cherge Q < 0.
void distribute_isotropically()
Populate the object with a new direction.
const ThermalizationAlgorithm algorithm_
Algorithm to choose for sampling of particles obeying conservation laws.
const double period_
Defines periodicity of the lattice in fm.
static double partial_density(const ParticleType &ptype, double T, double mub, double mus, double muq, bool account_for_resonance_widths=false)
Compute partial density of one hadron sort.
void thermalize(const Particles &particles, double time, int ntest)
Main thermalize function, that chooses the algorithm to follow (BF or mode sampling).
void thermalize_BF_algo(QuantumNumbers &conserved_initial, double time, int ntest)
Samples particles according to the BF algorithm by making use of the.
void print_statistics(const Clock &clock) const
Generates standard output with information about the thermodynamic properties of the lattice,...
void set_4momentum(const FourVector &momentum_vector)
Set the particle's 4-momentum directly.
The ThermLatticeNode class is intended to compute thermodynamical quantities in a cell given a set of...
Interface to the SMASH configuration files.
void set_rest_frame_quantities(double T0, double mub0, double mus0, double muq0, const ThreeVector v0)
Set all the rest frame quantities to some values, this is useful for testing.
GrandCanThermalizer(Configuration &conf, const std::array< double, 3 > lat_sizes, const std::array< double, 3 > origin, bool periodicity)
void compute_rest_frame_quantities(HadronGasEos &eos)
Temperature, chemical potentials and rest frame velocity are calculated given the hadron gas equation...
double e() const
Get energy density in the rest frame.
ThermalizationAlgorithm
Defines the algorithm used for the forced thermalization.
double lat_cell_volume_
Volume of a single lattice cell, necessary to convert thermal densities to actual particle numbers.
double T() const
Get the temperature.
double p_
Pressure in the rest frame.
void set_formation_time(const double &form_time)
Set the absolute formation time.
void boost_momentum(const ThreeVector &v)
Apply a Lorentz-boost to only the momentum.
double nq_
Net charge density of the cell in the computational frame.
A container class to hold all the arrays on the lattice and access them.
const bool BF_enforce_microcanonical_
Enforce energy conservation as part of BF sampling algorithm or not.
double N_total_in_cells_
Total number of particles over all cells in thermalization region.
Neutral non-strange mesons.
HadronClass get_class(size_t typelist_index) const
Defines the class of hadrons by quantum numbers.
ThreeVector uniform_in_cell() const
Mesons with strangeness S > 0.
Non-strange mesons (S = 0) with electric cherge Q > 0.
void update_thermalizer_lattice(const Particles &particles, const DensityParameters &par, bool ignore_cells_under_threshold=true)
Compute all the thermodynamical quantities on the lattice from particles.
ThreeVector v_
Velocity of the rest frame.
void renormalize_momenta(ParticleList &plist, const FourVector required_total_momentum)
Changes energy and momenta of the particles in plist to match the required_total_momentum.
Clock tracks the time in the simulation.
ParticleData sample_in_random_cell_mode_algo(const double time, F &&condition)
Samples one particle and the species, cell, momentum and coordinate are chosen from the corresponding...
void compute_N_in_cells_mode_algo(F &&condition)
Computes average number of particles in each cell for the mode algorithm.
double ns_
Net strangeness density of the cell in the computational frame.
void sample_in_random_cell_BF_algo(ParticleList &plist, const double time, size_t type_index)
The total number of particles of species type_index is defined by mult_int_ array that is returned by...
double mus() const
Get the net strangeness chemical potential.
std::vector< double > N_in_cells_
Number of particles to be sampled in one cell.
ParticleList sampled_list_
Newly generated particles by thermalizer.
std::vector< size_t > cells_to_sample_
Cells above critical energy density.
std::array< double, 7 > mult_classes_
The different hadron species according to the enum defined in.
HadronClass
Specifier to classify the different hadron species according to their quantum numbers.
static bool is_eos_particle(const ParticleType &ptype)
Check if a particle belongs to the EoS.
double nb_
Net baryon density of the cell in the computational frame.
HadronGasEos eos_
Hadron gas equation of state.
std::vector< double > mult_sort_
Real number multiplicity for each particle type.
std::vector< int > mult_int_
Integer multiplicity for each particle type.
double mub() const
Get the net baryon chemical potential.
double e_crit() const
Get the critical energy density.
double nb() const
Get net baryon density of the cell in the computational frame.
FourVector Tmu0() const
Get Four-momentum flow of the cell.
Value take(std::initializer_list< const char * > keys)
The default interface for SMASH to read configuration values.
void set_4position(const FourVector &pos)
Set the particle's 4-position directly.
const ParticleTypePtrList eos_typelist_
List of particle types from which equation of state is computed.
double mus_
Net strangeness chemical potential.
double mult_class(const HadronClass cl) const
void thermalize_mode_algo(QuantumNumbers &conserved_initial, double time)
Samples particles to the according to the mode algorithm.
void add_particle(const ParticleData &p, double factor)
Add particle contribution to Tmu0, nb, ns and nq May look like unused at first glance,...
RectangularLattice< ThermLatticeNode > & lattice() const
Getter function for the lattice.
Angles provides a common interface for generating directions: i.e., two angles that should be interpr...
ParticleList particles_to_insert() const
List of newly created particles to be inserted in the simulation.
The GrandCanThermalizer class implements the following functionality:
double muq_
Net charge chemical potential.
double sample_momenta_from_thermal(const double temperature, const double mass)
Samples a momentum from the Maxwell-Boltzmann (thermal) distribution in a faster way,...
ParticleList to_remove_
Particles to be removed after this thermalization step.
const double e_crit_
Critical energy density above which cells are thermalized.
std::unique_ptr< RectangularLattice< ThermLatticeNode > > lat_
The lattice on which the thermodynamic quantities are calculated.
double muq() const
Get the net charge chemical potential.
static const ParticleTypeList & list_all()
double e_
Energy density in the rest frame.
bool is_time_to_thermalize(std::unique_ptr< Clock > &clock) const
Check that the clock is close to n * period of thermalization, since the thermalization only happens ...