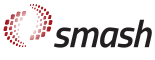 |
Version: SMASH-2.0
|
|
Go to the documentation of this file.
7 #ifndef SRC_INCLUDE_SMASH_PARTICLETYPE_H_
8 #define SRC_INCLUDE_SMASH_PARTICLETYPE_H_
54 throw std::runtime_error(
"unreachable");
181 return (I == 0) ? 0 : static_cast<double>(
isospin3()) / I;
329 const ParticleTypePtrList dlist)
const;
401 const double cms_energy,
int L = 0)
const;
414 const double cms_energy,
432 static const ParticleTypeList &
list_all();
492 using std::runtime_error::runtime_error;
603 using std::runtime_error::runtime_error;
674 return std::addressof(
lookup());
706 operator bool()
const {
return index_ != 0xffff; }
753 #endif // SRC_INCLUDE_SMASH_PARTICLETYPE_H_
void operator*=(Parity &x, Parity y)
static bool exists(PdgCode pdgcode)
static ParticleTypePtrList & list_nucleons()
PdgCode get_antiparticle() const
Construct the antiparticle to a given PDG code.
double spectral_function_no_norm(double m) const
Full spectral function without normalization factor.
static ParticleTypePtrList & list_anti_nucleons()
const ParticleType * operator->() const
void dump_width_and_spectral_function() const
Prints out width and spectral function versus mass to the standard output.
double width_
width of the particle
double width_at_pole() const
double min_mass_spectral() const
The minimum mass of the resonance, where the spectral function is non-zero.
DecayType is the abstract base class for all decay types.
bool wanted_decaymode(const DecayType &t, WhichDecaymodes wh) const
Helper Function that containes the if-statement logic that decides if a decay mode is either a hadron...
bool is_Deltastar() const
IsoParticleType * iso_multiplet() const
bool operator!=(const ParticleType &rhs) const
int baryon_number() const
ParticleType & operator=(const ParticleType &)=delete
assignment is not allowed, see copy constructor above
static ParticleTypePtrList & list_light_nuclei()
int32_t charge_
Charge of the particle; filled automatically from pdgcode_.
unsigned int spin() const
unsigned int spin_degeneracy() const
friend std::ostream & operator<<(std::ostream &out, const ParticleType &type)
EnergyMomentumTensor operator-(EnergyMomentumTensor a, const EnergyMomentumTensor &b)
Direct subtraction operator.
int antiparticle_sign() const
double spectral_function(double m) const
Full spectral function of the resonance (relativistic Breit-Wigner distribution with mass-dependent ...
int isospin_
Isospin of the particle; filled automatically from pdgcode_.
double get_partial_in_width(const double m, const ParticleData &p_a, const ParticleData &p_b) const
Get the mass-dependent partial in-width of a resonance with mass m, decaying into two given daughter ...
int antiparticle_sign() const
static void create_type_list(const std::string &particles)
Initialize the global ParticleType list (list_all) from the given input data.
ParticleTypePtr()=default
Default construction initializes with an invalid index.
std::string name_
name of the particle
unsigned int spin() const
ParticleTypePtr operator&() const
Returns an object that acts like a pointer, except that it requires only 2 bytes and inhibits pointer...
double min_mass_kinematic_
minimum kinematically allowed mass of the particle Mutable, because it is initialized at first call o...
static ParticleTypePtrList & list_anti_Deltas()
static ParticleTypePtrList & list_Deltas()
double total_width(const double m) const
Get the mass-dependent total width of a particle with mass m.
static const ParticleType & find(PdgCode pdgcode)
Returns the ParticleType object for the given pdgcode.
int32_t get_decimal() const
bool has_antiparticle() const
const DecayModes & decay_modes() const
unsigned int spin_degeneracy() const
double norm_factor_
This normalization factor ensures that the spectral function is normalized to unity,...
IsoParticleType * iso_multiplet_
Container for the isospin multiplet information.
DecayBranchList get_partial_widths(const FourVector p, const ThreeVector x, WhichDecaymodes wh) const
Get all the mass-dependent partial decay widths of a particle with mass m.
const ParticleType & lookup() const
Helper function that does the ParticleType lookup from the stored index.
int isospin() const
Returns twice the isospin vector length .
int I3_
Isospin projection of the particle; filled automatically from pdgcode_.
Ignore dilepton decay modes widths.
std::pair< double, double > sample_resonance_masses(const ParticleType &t2, const double cms_energy, int L=0) const
Resonance mass sampling for 2-particle final state with two resonances.
double sample_resonance_mass(const double mass_stable, const double cms_energy, int L=0) const
Resonance mass sampling for 2-particle final state with one resonance (type given by 'this') and one ...
WhichDecaymodes
Decide which decay mode widths are returned in get partical widths.
double isospin3_rel() const
ParticleTypePtr(std::uint16_t i)
Constructs a pointer to the ParticleType object at offset i.
double spectral_function_simple(double m) const
This one is the most simple form of the spectral function, using a Cauchy distribution (non-relativis...
const std::string & name() const
bool operator<(const ParticleTypePtr &rhs) const
"Less than" operator
PdgCode pdgcode_
PDG Code of the particle.
static const ParticleTypePtr try_find(PdgCode pdgcode)
Returns the ParticleTypePtr for the given pdgcode.
ParticleType(std::string n, double m, double w, Parity p, PdgCode id)
Creates a fully initialized ParticleType object.
Parity parity_
Parity of the particle.
static void check_consistency()
bool operator<(const ParticleType &rhs) const
"Less than" operator for sorting the ParticleType list (by PDG code)
ParticleTypePtr get_antiparticle() const
std::uint16_t index_
Stores the index of the references ParticleType object in the global vector.
double max_factor2_
Maximum factor for double-res mass sampling, cf. sample_resonance_masses.
const ParticleType & operator*() const
double get_partial_width(const double m, const ParticleTypePtrList dlist) const
Get the mass-dependent partial width of a resonance with mass m, decaying into two given daughter par...
bool is_Nstar1535() const
double partial_width(const double m, const DecayBranch *mode) const
Get the mass-dependent partial decay width of a particle with mass m in a particular decay mode.
bool operator!=(const ParticleTypePtr &rhs) const
double min_mass_spectral_
minimum mass, where the spectral function is non-zero Mutable, because it is initialized at first cal...
double spectral_function_const_width(double m) const
double max_factor1_
Maximum factor for single-res mass sampling, cf. sample_resonance_mass.
Parity
Represent the parity of a particle type.
bool has_antiparticle() const
static ParticleTypePtrList & list_baryon_resonances()
double min_mass_kinematic() const
The minimum mass of the resonance that is kinematically allowed.
double mass_
pole mass of the particle
bool operator==(const ParticleType &rhs) const
EnergyMomentumTensor operator*(EnergyMomentumTensor a, const double b)
Direct multiplication operator.
Only return dilepton decays widths.
bool is_Nstar1535() const
static constexpr double width_cutoff
Decay width cutoff for considering a particle as stable.
bool operator==(const ParticleTypePtr &rhs) const
static const ParticleTypeList & list_all()
int32_t charge() const
The charge of the particle.
int baryon_number() const