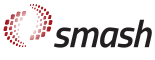 |
Version: SMASH-2.0
|
|
Go to the documentation of this file.
8 #ifndef SRC_INCLUDE_SMASH_ISOPARTICLETYPE_H_
9 #define SRC_INCLUDE_SMASH_ISOPARTICLETYPE_H_
12 #include <unordered_map>
117 static const IsoParticleTypeList &
list_all();
156 using std::runtime_error::runtime_error;
195 const bf::path &tabulations_path);
277 #endif // SRC_INCLUDE_SMASH_ISOPARTICLETYPE_H_
int isospin() const
Returns twice the total isospin of the multiplet.
const IsoParticleType * anti_multiplet() const
Return a multiplet of antiparticles, if it is different from the original multiplet.
double width() const
Returns the (average) multiplet width.
static const IsoParticleType & find(const std::string &name)
Returns the IsoParticleType object for the given name.
unsigned int spin_
twice the spin of the multiplet
std::string name_
name of the multiplet
const std::string & name() const
Returns the name of the multiplet.
double get_integral_rhoR(double sqrts)
Look up the tabulated resonance integral for the XX -> rhoR cross section.
bool has_anti_multiplet() const
Check if there is a multiplet of antiparticles, which is different from the original multiplet.
double mass_
(average) mass of the multiplet
unsigned int spin() const
Returns twice the spin of the multiplet.
ParticleTypePtrList states_
list of states that are contained in the multiplet
Parity parity_
parity of the multiplet
Tabulation * XS_NR_tabulation_
A tabulation for the NN -> NR cross sections, where R is a resonance from this multiplet.
Tabulation * XS_RK_tabulation_
A tabulation of the spectral integral for the NK -> RK cross sections.
bool operator==(const IsoParticleType &rhs) const
Returns whether the two IsoParticleType objects have the same PDG code for their first state; if so,...
static void tabulate_integrals(sha256::Hash hash, const bf::path &tabulations_path)
Tabulate all relevant integrals.
Tabulation * XS_rhoR_tabulation_
A tabulation for the ρρ integrals.
double get_integral_piR(double sqrts)
Look up the tabulated resonance integral for the XX -> piR cross section.
static const ParticleTypePtr find_state(const std::string &name)
Returns the ParticleType object for the given name, by first finding the correct multiplet and then l...
static const std::vector< const IsoParticleType * > list_baryon_resonances()
Returns a list of all IsoParticleTypes that are baryon resonances.
void add_state(const ParticleType &type)
Add a new state to an existing multiplet (and check if isospin symmetry is fulfilled).
static bool exists(const std::string &name)
Returns whether the ParticleType with the given pdgcode exists.
double get_integral_NR(double sqrts)
Look up the tabulated resonance integral for the XX -> NR cross section.
Tabulation * XS_piR_tabulation_
A tabulation of the spectral integral for the dpi -> d'pi cross sections.
A class for storing a one-dimensional lookup table of floating-point values.
double mass() const
Returns the (average) multiplet mass.
std::array< uint8_t, HASH_SIZE > Hash
A SHA256 hash.
Tabulation * XS_DeltaR_tabulation_
A tabulation for the NN -> RΔ cross sections, where R is a resonance from this multiplet.
static const IsoParticleType * try_find(const std::string &name)
Returns the IsoParticleType pointer for the given name.
double get_integral_RK(double sqrts)
Look up the tabulated resonance integral for the XX -> RK cross section.
static IsoParticleType & find_private(const std::string &name)
Private version of the 'find' method that returns a non-const reference.
double get_integral_RR(IsoParticleType *type_res_2, double sqrts)
Look up the tabulated resonance integral for the XX -> RR cross section.
Parity
Represent the parity of a particle type.
IsoParticleType & operator=(const IsoParticleType &)=delete
Assignment is not allowed, see copy constructor above.
double width_
(average) width of the multiplet
IsoParticleType(const std::string &n, double m, double w, unsigned int s, Parity p)
Creates a fully initialized IsoParticleType object.
ParticleTypePtrList get_states() const
Returns list of states that form part of the multiplet.
static const IsoParticleTypeList & list_all()
Returns a list of all IsoParticleTypes.
static void create_multiplet(const ParticleType &type)
Add a new multiplet to the global list of IsoParticleTypes, which contains type.