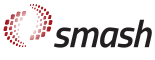 |
Version: SMASH-2.0
|
|
Go to the documentation of this file.
10 #ifndef SRC_INCLUDE_SMASH_HYPERSURFACECROSSINGACTION_H_
11 #define SRC_INCLUDE_SMASH_HYPERSURFACECROSSINGACTION_H_
37 const double time_until)
38 :
Action(in_part, out_part, time_until,
80 const ParticleList &plist,
double dt,
const double,
81 const std::vector<FourVector> &beam_momentum)
const override;
85 const ParticleList &,
const ParticleList &,
double,
86 const std::vector<FourVector> &)
const override {
92 const ParticleList &,
const Particles &,
double,
93 const std::vector<FourVector> &)
const override {
118 const double tau)
const;
131 const double tau)
const;
136 #endif // SRC_INCLUDE_SMASH_HYPERSURFACECROSSINGACTION_H_
ActionList find_actions_in_cell(const ParticleList &plist, double dt, const double, const std::vector< FourVector > &beam_momentum) const override
Find the next hypersurface crossings for each particle that occur within the timestepless propagation...
ParticleList incoming_particles_
List with data of incoming particles.
ActionList find_actions_with_neighbors(const ParticleList &, const ParticleList &, double, const std::vector< FourVector > &) const override
Ignore the neighbor searches for hypersurface crossing.
void generate_final_state() override
Generate the final state of the hypersurface crossing particles.
void check_conservation(const uint32_t id_process) const override
Check various conservation laws.
const double prop_time_
Proper time of the hypersurface in fm.
HypersurfacecrossingAction(const ParticleData &in_part, const ParticleData &out_part, const double time_until)
Construct hypersurfacecrossing action.
bool crosses_hypersurface(ParticleData &pdata_before_propagation, ParticleData &pdata_after_propagation, const double tau) const
Determine whether particle crosses hypersurface within next timestep during propagation.
double get_partial_weight() const override
Return the specific weight for the chosen outgoing channel, which is mainly used for the partial weig...
FourVector coordinates_on_hypersurface(ParticleData &pdata_before_propagation, ParticleData &pdata_after_propagation, const double tau) const
Find the coordinates where particle crosses hypersurface.
ActionList find_final_actions(const Particles &, bool) const override
No final actions for hypersurface crossing.
ActionList find_actions_with_surrounding_particles(const ParticleList &, const Particles &, double, const std::vector< FourVector > &) const override
Ignore the surrounding searches for hypersurface crossing.
Hypersurface crossing Particles are removed from the evolution and printed to a separate output to se...
double get_total_weight() const override
Return the total weight value, which is mainly used for the weight output entry.
void format_debug_output(std::ostream &out) const override
HyperSurfaceCrossActionsFinder(double tau)
Construct hypersurfacecrossing action finder.
ProcessType
Process Types are used to identify the type of the process.