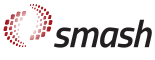 |
Version: SMASH-2.0
|
|
Go to the documentation of this file.
10 #ifndef SRC_INCLUDE_SMASH_MODUSDEFAULT_H_
11 #define SRC_INCLUDE_SMASH_MODUSDEFAULT_H_
60 const OutputsList& = {}) {
73 bool is_box()
const {
return false; }
119 const Particles& particles,
double min_cell_length,
120 double timestep_duration,
122 return {particles, min_cell_length, timestep_duration, strategy};
131 std::unique_ptr<GrandCanThermalizer> create_grandcan_thermalizer(
172 const std::array<double, 3> l = conf.
take({
"Lattice_Sizes"});
173 const std::array<double, 3> origin = {-0.5 * l[0], -0.5 * l[1],
175 const bool periodicity =
false;
176 return make_unique<GrandCanThermalizer>(conf, l, origin, periodicity);
184 using std::invalid_argument::invalid_argument;
192 using BadInput::BadInput;
198 #endif // SRC_INCLUDE_SMASH_MODUSDEFAULT_H_
double impact_parameter() const
int impose_boundary_conditions(Particles *, const OutputsList &={})
Enforces sensible positions for the particles.
double equilibration_time() const
bool cll_in_nucleus() const
Grid< GridOptions::Normal > create_grid(const Particles &particles, double min_cell_length, double timestep_duration, CellSizeStrategy strategy=CellSizeStrategy::Optimal) const
Creates the Grid with normal boundary conditions.
Look for optimal cell size.
double max_timestep(double) const
int total_N_number() const
double velocity_target() const
CellSizeStrategy
Indentifies the strategy of determining the cell size.
Interface to the SMASH configuration files.
Abstracts a list of cells that partition the particles in the experiment into regions of space that c...
double velocity_projectile() const
Value take(std::initializer_list< const char * > keys)
The default interface for SMASH to read configuration values.
double nuclei_passing_time() const
Get the passing time of the two nuclei in a collision.
int proj_N_number() const
FermiMotion
Option to use Fermi Motion.
FermiMotion fermi_motion() const