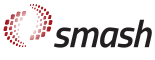 |
Version: SMASH-2.0
|
|
Go to the documentation of this file.
14 #include <initializer_list>
29 double xs_high_energy(
double mandelstam_s,
bool is_opposite_charge,
double ma,
30 double mb,
double P,
double R1,
double R2) {
31 const double M = 2.1206;
32 const double H = 0.272;
33 const double eta1 = 0.4473;
34 const double eta2 = 0.5486;
35 const double s_sab = mandelstam_s / (ma + mb + M) / (ma + mb + M);
37 H * std::log(s_sab) * std::log(s_sab) + P + R1 * std::pow(s_sab, -eta1);
38 xs = is_opposite_charge ? xs + R2 * std::pow(s_sab, -eta2)
39 : xs - R2 * std::pow(s_sab, -eta2);
44 return xs_high_energy(mandelstam_s,
false, 0.939, 0.939, 34.41, 13.07, 7.394);
48 return xs_high_energy(mandelstam_s,
true, 0.939, 0.939, 34.41, 13.07, 7.394);
52 return xs_high_energy(mandelstam_s,
false, 0.939, 0.939, 34.41, 12.52, 6.66);
56 return xs_high_energy(mandelstam_s,
true, 0.939, 0.939, 34.41, 12.52, 6.66);
60 return xs_high_energy(mandelstam_s,
false, 0.939, 0.138, 18.75, 9.56, 1.767);
64 return xs_high_energy(mandelstam_s,
true, 0.939, 0.138, 18.75, 9.56, 1.767);
68 const double xs_ref = 120.;
70 const double constant_a = 0.05;
71 const double constant_b = 0.6;
72 const double factor = constant_a * constant_a * s_ref /
73 ((mandelstam_s - s_ref) * (mandelstam_s - s_ref) +
74 constant_a * constant_a * s_ref) +
76 return xs_ref * (s_ref / mandelstam_s) * factor;
81 const double sqrts = std::sqrt(mandelstam_s);
85 double xs = xs_0 * std::pow(std::log(sqrts / e_0), lambda_pow);
111 std::vector<double> dedup_x;
112 std::vector<double> dedup_y;
113 std::tie(dedup_x, dedup_y) =
dedup_avg(x, y);
114 dedup_y =
smooth(dedup_x, dedup_y, 0.1, 5);
116 make_unique<InterpolateDataLinear<double>>(dedup_x, dedup_y);
123 const double p_lab = (m1 > m2) ?
plab_from_s(mandelstam_s, m2, m1)
125 const auto logp = std::log(p_lab);
126 return 11.4 * std::pow(p_lab, -0.4) + 0.079 * logp * logp;
130 const double p_lab = (m1 > m2) ?
plab_from_s(mandelstam_s, m2, m1)
142 if (mandelstam_s < 2.25) {
144 }
else if (mandelstam_s > 4.84) {
145 const auto logp = std::log(p_lab);
146 sigma = 11.4 * std::pow(p_lab, -0.4) + 0.079 * logp * logp;
159 make_unique<InterpolateDataSpline>(x, y);
161 sigma -= (*piplusp_elastic_res_interpolation)(mandelstam_s);
177 std::vector<double> dedup_x;
178 std::vector<double> dedup_y;
179 std::tie(dedup_x, dedup_y) =
dedup_avg(x, y);
180 dedup_y =
smooth(dedup_x, dedup_y, 0.2, 5);
182 make_unique<InterpolateDataLinear<double>>(dedup_x, dedup_y);
197 std::vector<double> dedup_x;
198 std::vector<double> dedup_y;
199 std::tie(dedup_x, dedup_y) =
dedup_avg(x, y);
200 dedup_y =
smooth(dedup_x, dedup_y, 0.2, 6);
202 make_unique<InterpolateDataLinear<double>>(dedup_x, dedup_y);
211 const auto logp = std::log(p_lab);
212 if (mandelstam_s < 1.69) {
214 }
else if (mandelstam_s > 4.84) {
215 sigma = 1.76 + 11.2 * std::pow(p_lab, -0.64) + 0.043 * logp * logp;
224 if (mandelstam_s > 3.24 && mandelstam_s < 3.8809) {
225 sigma *= (0.12 * std::cos(2 * M_PI * (std::sqrt(mandelstam_s) - 1.8) /
236 std::vector<double> dedup_x;
237 std::vector<double> dedup_y;
238 std::tie(dedup_x, dedup_y) =
dedup_avg(x, y);
240 make_unique<InterpolateDataSpline>(dedup_x, dedup_y);
242 sigma -= (*piminusp_elastic_res_interpolation)(mandelstam_s);
258 std::vector<double> dedup_x;
259 std::vector<double> dedup_y;
260 std::tie(dedup_x, dedup_y) =
dedup_avg(x, y);
261 dedup_y =
smooth(dedup_x, dedup_y, 0.2, 6);
263 make_unique<InterpolateDataLinear<double>>(dedup_x, dedup_y);
278 std::vector<double> dedup_x;
279 std::vector<double> dedup_y;
280 std::tie(dedup_x, dedup_y) =
dedup_avg(x, y);
281 dedup_y =
smooth(dedup_x, dedup_y, 0.2, 6);
283 make_unique<InterpolateDataLinear<double>>(dedup_x, dedup_y);
298 std::vector<double> dedup_x;
299 std::vector<double> dedup_y;
300 std::tie(dedup_x, dedup_y) =
dedup_avg(x, y);
301 dedup_y =
smooth(dedup_x, dedup_y, 0.2, 6);
303 make_unique<InterpolateDataLinear<double>>(dedup_x, dedup_y);
305 const double sqrts = std::sqrt(mandelstam_s);
315 }
else if (p_lab < 0.8) {
316 return 23.5 + 1000 *
pow_int(p_lab - 0.7, 4);
317 }
else if (p_lab < 2.0) {
318 return 1250 / (p_lab + 50) - 4 * (p_lab - 1.3) * (p_lab - 1.3);
319 }
else if (p_lab < 2.776) {
320 return 77 / (p_lab + 1.5);
322 const auto logp = std::log(p_lab);
323 return 11.9 + 26.9 * std::pow(p_lab, -1.21) + 0.169 * logp * logp -
329 const double p_lab = (m1 > m2) ?
plab_from_s(mandelstam_s, m2, m1)
331 const auto logp = std::log(p_lab);
332 return 11.9 + 26.9 * std::pow(p_lab, -1.21) + 0.169 * logp * logp -
339 return 34 * std::pow(p_lab / 0.4, -2.104);
340 }
else if (p_lab < 0.8) {
341 return 23.5 + 1000 *
pow_int(p_lab - 0.7, 4);
342 }
else if (p_lab < 1.5) {
343 return 23.5 + 24.6 / (1 + std::exp(-(p_lab - 1.2) / 0.1));
344 }
else if (p_lab < 5.0) {
345 return 41 + 60 * (p_lab - 0.9) * std::exp(-1.2 * p_lab);
347 const auto logp = std::log(p_lab);
348 return 48.0 + 0.522 * logp * logp - 4.51 * logp;
358 }
else if (p_lab < 0.8) {
359 return 33 + 196 * std::pow(std::abs(p_lab - 0.95), 2.5);
360 }
else if (p_lab < 2.0) {
361 return 31 / std::sqrt(p_lab);
362 }
else if (p_lab < 2.776) {
363 return 77 / (p_lab + 1.5);
365 const auto logp = std::log(p_lab);
366 return 11.9 + 26.9 * std::pow(p_lab, -1.21) + 0.169 * logp * logp -
373 const auto logp = std::log(p_lab);
375 return 6.3555 * std::pow(p_lab, -3.2481) * std::exp(-0.377 * logp * logp);
376 }
else if (p_lab < 1.0) {
377 return 33 + 196 * std::pow(std::abs(p_lab - 0.95), 2.5);
378 }
else if (p_lab < 2.0) {
379 return 24.2 + 8.9 * p_lab;
380 }
else if (p_lab < 5.0) {
383 return 48.0 + 0.522 * logp * logp - 4.51 * logp;
391 }
else if (p_lab < 5.0) {
392 return 31.6 + 18.3 / p_lab - 1.1 / (p_lab * p_lab) - 3.8 * p_lab;
394 const auto logp = std::log(p_lab);
395 return 10.2 + 52.7 * std::pow(p_lab, -1.16) + 0.125 * logp * logp -
403 return 271.6 * std::exp(-1.1 * p_lab * p_lab);
404 }
else if (p_lab < 5.0) {
405 return 75.0 + 43.1 / p_lab + 2.6 / (p_lab * p_lab) - 3.9 * p_lab;
407 const auto logp = std::log(p_lab);
408 return 38.4 + 77.6 * std::pow(p_lab, -0.64) + 0.26 * logp * logp -
414 const double tmp = std::sqrt(mandelstam_s) - 2.172;
415 return 4.0 + 0.27 / (tmp * tmp + 0.065 * 0.065);
419 const double s = mandelstam_s;
425 constexpr
double a0 = 10.508;
426 constexpr
double a1 = -3.716;
427 constexpr
double a2 = 1.845;
428 constexpr
double a3 = -0.764;
429 constexpr
double a4 = 0.508;
432 const double p_lab2 = p_lab * p_lab;
434 return (a0 + a1 * p_lab + a2 * p_lab2) / (1 + a3 * p_lab + a4 * p_lab2);
454 std::vector<double> dedup_x;
455 std::vector<double> dedup_y;
456 std::tie(dedup_x, dedup_y) =
dedup_avg(x, y);
457 dedup_y =
smooth(dedup_x, dedup_y, 0.1, 5);
459 make_unique<InterpolateDataLinear<double>>(dedup_x, dedup_y);
468 if (std::sqrt(mandelstam_s) < 1.68) {
474 constexpr
double a0 = 186.03567644;
475 constexpr
double a1 = 0.22002795;
476 constexpr
double a2 = 0.64907116;
478 const double p_i = p_lab;
479 const double p_f = p_lab;
481 const double ratio = a1 * a1 / (a1 * a1 + p_f * p_f);
482 sigma = a0 * p_f / (p_i * mandelstam_s) * std::pow(ratio, a2);
494 make_unique<InterpolateDataSpline>(x, y);
496 const auto old_sigma = sigma;
497 sigma -= (*kminusp_elastic_res_interpolation)(p_lab);
499 std::cout <<
"NEGATIVE SIGMA: sigma=" << sigma
500 <<
", sqrt(s)=" << std::sqrt(mandelstam_s)
501 <<
", sig_el_exp=" << old_sigma
502 <<
", sig_el_res=" << (*kminusp_elastic_res_interpolation)(p_lab)
535 std::vector<double> dedup_x;
536 std::vector<double> dedup_y;
537 std::tie(dedup_x, dedup_y) =
dedup_avg(x, y);
538 dedup_y =
smooth(dedup_x, dedup_y, 0.1, 5);
540 make_unique<InterpolateDataLinear<double>>(dedup_x, dedup_y);
551 std::vector<double> dedup_x;
552 std::vector<double> dedup_y;
553 std::tie(dedup_x, dedup_y) =
dedup_avg(x, y);
554 dedup_y =
smooth(dedup_x, dedup_y, 0.05, 5);
556 make_unique<InterpolateDataLinear<double>>(dedup_x, dedup_y);
572 static void initialize(std::unordered_map<std::pair<uint64_t, uint64_t>,
double,
587 double weight_numerator,
double weight_other) {
588 assert(weight_numerator + weight_other != 0);
591 pack(c.pdgcode().code(), d.pdgcode().code()));
592 const double ratio = weight_numerator / (weight_numerator + weight_other);
603 type_p, type_K_p, type_K_z, type_Delta_pp);
605 type_p, type_K_p, type_K_p, type_Delta_p);
607 add_to_ratios(type_p, type_K_p, type_K_z, type_Delta_pp, weight1, weight2);
608 add_to_ratios(type_p, type_K_p, type_K_p, type_Delta_p, weight2, weight1);
612 type_n, type_K_p, type_K_z, type_Delta_p);
614 type_n, type_K_p, type_K_p, type_Delta_z);
616 add_to_ratios(type_n, type_K_p, type_K_z, type_Delta_p, weight1, weight2);
617 add_to_ratios(type_n, type_K_p, type_K_p, type_Delta_z, weight2, weight1);
623 type_p, type_K_z, type_K_z, type_Delta_p);
625 type_p, type_K_z, type_K_p, type_Delta_z);
627 add_to_ratios(type_p, type_K_z, type_K_z, type_Delta_p, weight1, weight2);
628 add_to_ratios(type_p, type_K_z, type_K_p, type_Delta_z, weight2, weight1);
632 type_n, type_K_z, type_K_z, type_Delta_z);
634 type_n, type_K_z, type_K_p, type_Delta_m);
636 add_to_ratios(type_n, type_K_z, type_K_z, type_Delta_z, weight1, weight2);
637 add_to_ratios(type_n, type_K_z, type_K_p, type_Delta_m, weight2, weight1);
649 for (
const auto&
p : {&a, &b, &c, &d}) {
650 if (
p->is_nucleon()) {
652 flip =
p->antiparticle_sign();
654 assert(
p->antiparticle_sign() == flip);
658 const auto key = std::make_pair(
670 constexpr
double a0 = 100;
671 constexpr
double a1 = 0.15;
672 constexpr
unsigned a2 = 2;
675 const double p_i = p_lab;
676 const double p_f = p_lab;
678 return a0 * p_f / (p_i * mandelstam_s) *
679 pow_int(a1 * a1 / (a1 * a1 + p_f * p_f), a2);
710 assert(p_lambda != 0);
711 assert(sqrts_sqrts0 >= 0);
712 return 37.15 / 2 * p_N / p_lambda * std::pow(sqrts_sqrts0, -0.16);
720 assert(sqrts_sqrts0 >= 0);
721 return 24.3781 * std::pow(sqrts_sqrts0, -0.479);
729 assert(sqrts_sqrts0 >= 0);
730 if (sqrts_sqrts0 < 0.03336) {
731 return 6.475 * std::pow(sqrts_sqrts0, -0.4167);
733 return 14.5054 * std::pow(sqrts_sqrts0, -0.1795);
742 assert(sqrts_sqrts0 >= 0);
743 if (sqrts_sqrts0 < 0.09047) {
744 return 5.625 * std::pow(sqrts_sqrts0, -0.318);
746 return 4.174 * std::pow(sqrts_sqrts0, -0.4421);
763 return 14.194 * std::pow(sqrts_sqrts0, -0.442);
double ppbar_high_energy(double mandelstam_s)
ppbar total cross section at high energies
double xs_high_energy(double mandelstam_s, bool is_opposite_charge, double ma, double mb, double P, double R1, double R2)
total hadronic cross sections at high energies parametrized in the 2016 PDG book(http://pdg....
double k0p_elastic_background(double mandelstam_s)
K0 p elastic background cross section parametrization Source: Buss:2011mx , B.3.9.
std::pair< std::vector< T >, std::vector< T > > dedup_avg(const std::vector< T > &x, const std::vector< T > &y)
Remove duplicates from data (x, y) by averaging y.
double kplusn_inelastic_background(double mandelstam_s)
K+ n inelastic background cross section parametrization Source: Buss:2011mx , B.3....
const std::initializer_list< double > KMINUSP_RES_SIG
Elastic K̅⁻ N⁺ cross section contributions from decays.
double kplusn_k0p(double mandelstam_s)
K+ n charge exchange cross section parametrization.
double sigma0sigma0_ximinusp(double sqrts_sqrts0)
Sigma0 Sigma0 <-> Xi- p cross section parametrization Two hyperon exchange, based on effective model ...
double plab_from_s(double mandelstam_s, double mass)
Convert Mandelstam-s to p_lab in a fixed-target collision.
double deuteron_nucleon_elastic(double mandelstam_s)
Deuteron nucleon elastic cross-section [mb] parametrized by Oh:2009gx .
static std::unique_ptr< InterpolateDataLinear< double > > piminusp_sigma0k0_interpolation
An interpolation that gets lazily filled using the PIMINUSP_SIGMA0K0_RES data.
double sigma0sigma0_xi0n(double sqrts_sqrts0)
Sigma0 Sigma0 <-> Xi0 n cross section parametrization Two hyperon exchange, based on effective model ...
double ppbar_elastic(double mandelstam_s)
ppbar elastic cross section parametrization Source: Bass:1998ca
std::vector< T > smooth(const std::vector< T > &x, const std::vector< T > &y, T span=2./3, size_t iter=3, T delta=0)
Apply the LOWESS smoother (see the reference below) to the given data (x, y).
double kminusn_piminuslambda(double sqrts)
K- n <-> pi- Lambda cross section parametrization Follow from the parametrization with the same stran...
double kminusp_piplussigmaminus(double sqrts)
K- p <-> pi+ Sigma- cross section parametrization Taken from UrQMD (Graef:2014mra ).
const std::initializer_list< double > PIMINUSP_SIGMA0K0_RES_SQRTS
pi- p to Sigma0 K0 cross section: square root s
const std::initializer_list< double > PIMINUSP_ELASTIC_SIG
PDG data on pi- p elastic cross section: cross section.
static std::unique_ptr< InterpolateDataLinear< double > > piplusp_elastic_interpolation
An interpolation that gets lazily filled using the PIPLUSP_ELASTIC_SIG data.
double kbar0p_elastic_background(double mandelstam_s)
Kbar0 p elastic background cross section parametrization Source: Buss:2011mx , B.3....
double kminusp_pi0sigma0(double sqrts)
K- p <-> pi0 Sigma0 cross section parametrization Fit to Landolt-Börnstein instead of UrQMD values.
double piplusp_high_energy(double mandelstam_s)
pi+p total cross section at high energies
const std::initializer_list< double > PIMINUSP_SIGMAMINUSKPLUS_P_LAB
PDG data on pi- p to Sigma- K+ cross section: momentum in lab frame.
static std::unique_ptr< InterpolateDataLinear< double > > piminusp_elastic_interpolation
An interpolation that gets lazily filled using the PIMINUSP_ELASTIC data.
static double piplusp_elastic_pdg(double mandelstam_s)
double pipi_string_hard(double mandelstam_s)
pion-pion hard scattering cross section (with partonic scattering)
double pp_elastic(double mandelstam_s)
pp elastic cross section parametrization Source: Weil:2013mya , eq.
static std::unique_ptr< InterpolateDataLinear< double > > piminusp_lambdak0_interpolation
An interpolation that gets lazily filled using the PIMINUSP_LAMBDAK0 data.
double k0n_elastic_background(double mandelstam_s)
K0 n elastic background cross section parametrization Source: Buss:2011mx , B.3.9.
const std::initializer_list< double > PIMINUSP_LAMBDAK0_P_LAB
PDG data on pi- p to Lambda K0 cross section: momentum in lab frame.
double lambdalambda_xi0n(double sqrts_sqrts0, double p_N, double p_lambda)
Lambda Lambda <-> Xi0 n cross section parametrization Two hyperon exchange, based on effective model ...
double xs_ppbar_annihilation(double mandelstam_s)
parametrized cross-section for proton-antiproton annihilation used in the UrQMD model
double lambdalambda_ximinusp(double sqrts_sqrts0, double p_N, double p_lambda)
Lambda Lambda <-> Xi- p cross section parametrization Two hyperon exchange, based on effective model ...
const std::initializer_list< double > KMINUSP_RES_SQRTS
Center-of-mass energy list for K̅⁻ N⁺
constexpr double nucleon_mass
Nucleon mass in GeV.
static std::unique_ptr< InterpolateDataLinear< double > > kplusp_total_interpolation
An interpolation that gets lazily filled using the KPLUSP_TOT data.
double isospin_clebsch_gordan_sqr_2to2(const ParticleType &p_a, const ParticleType &p_b, const ParticleType &p_c, const ParticleType &p_d, const int I=-1)
Calculate the squared isospin Clebsch-Gordan coefficient for a 2-to-2 reaction A + B -> C + D.
double lambdasigma0_ximinusp(double sqrts_sqrts0)
Lambda Sigma0 <-> Xi- p cross section parametrization Two hyperon exchange, based on effective model ...
double ppbar_total(double mandelstam_s)
ppbar total cross section parametrization Source: Bass:1998ca
const std::initializer_list< double > PIMINUSP_SIGMA0K0_RES_SIG
pi- p to Sigma0 K0 cross section: cross section
double kminusp_elastic_background(double mandelstam_s)
K- p elastic background cross section parametrization Source: Buss:2011mx , B.3.9.
static double kminusp_elastic_pdg(double mandelstam_s)
constexpr double really_small
Numerical error tolerance.
static const ParticleType & find(PdgCode pdgcode)
Returns the ParticleType object for the given pdgcode.
double NN_string_hard(double mandelstam_s)
nucleon-nucleon hard scattering cross section (with partonic scattering)
const std::initializer_list< double > KPLUSP_TOT_SIG
PDG data on K+ p total cross section: cross section.
double kplusn_elastic_background(double mandelstam_s)
K+ n elastic background cross section parametrization sigma(K+n->K+n) = sigma(K+n->K0p) = 0....
const std::initializer_list< double > PIMINUSP_SIGMAMINUSKPLUS_SIG
PDG data on pi- p to Sigma- K+ cross section: cross section.
double piminusp_sigmaminuskplus_pdg(double mandelstam_s)
pi- p -> Sigma- K+ cross section parametrization, PDG data.
constexpr T square(const T base)
Efficient template for calculating the square.
double piplusp_elastic_AQM(double mandelstam_s, double m1, double m2)
An overload of piplusp_elastic_high_energy in which the very low part is replaced by a flat 5 mb cros...
double kminusn_elastic_background(double mandelstam_s)
K- n elastic background cross section parametrization Source: Buss:2011mx , B.3.9.
double pp_high_energy(double mandelstam_s)
pp total cross section at high energies
const std::initializer_list< double > PIPLUSP_SIGMAPLUSKPLUS_P_LAB
PDG data on pi+ p to Sigma+ K+ cross section: momentum in lab frame.
double np_high_energy(double mandelstam_s)
np total cross section at high energies
const std::initializer_list< double > KPLUSP_TOT_PLAB
PDG data on K+ p total cross section: momentum in lab frame.
Calculate and store isospin ratios for K N -> K Delta reactions.
const std::initializer_list< double > PIPLUSP_ELASTIC_SIG
PDG data on pi+ p elastic cross section: cross section.
double sigma0sigmaminus_ximinusn(double sqrts_sqrts0)
Sigma0 Sigma- <-> Xi- n cross section parametrization Two hyperon exchange, based on effective model ...
double pp_elastic_high_energy(double mandelstam_s, double m1, double m2)
pp elastic cross section parametrization, with only the high energy part generalized to all energy re...
const std::initializer_list< double > PIMINUSP_RES_SIG
Elastic π⁻N⁺ cross section contributions from decays.
double kminusp_pi0lambda(double sqrts)
K- p <-> pi0 Lambda cross section parametrization Fit to Landolt-Börnstein instead of UrQMD values.
double lambdasigmaplus_xi0p(double sqrts_sqrts0)
Lambda Sigma+ <-> Xi0 p cross section parametrization Two hyperon exchange, based on effective model ...
double piplusp_elastic_high_energy(double mandelstam_s, double m1, double m2)
pi+p elactic cross section parametrization.
double deuteron_pion_elastic(double mandelstam_s)
Deuteron pion elastic cross-section [mb] parametrized to fit pi-d elastic scattering data (the data c...
const std::initializer_list< double > PIMINUSP_RES_SQRTS
Center-of-mass energy.
double np_total(double mandelstam_s)
np total cross section parametrization Sources: low-p: Cugnon:1996kh highest-p: Buss:2011mx
double kplusp_elastic_background(double mandelstam_s)
K+ p elastic background cross section parametrization.
static std::unique_ptr< InterpolateDataSpline > piplusp_elastic_res_interpolation
A null interpolation that gets filled using the PIPLUSP_RES data.
KaonNucleonRatios kaon_nucleon_ratios
static std::unique_ptr< InterpolateDataLinear< double > > piminusp_sigmaminuskplus_interpolation
An interpolation that gets lazily filled using the PIMINUSP_SIGMAMINUSKPLUS data.
const std::initializer_list< double > PIMINUSP_LAMBDAK0_SIG
PDG data on pi- p to Lambda K0 cross section: cross section.
double piminusp_sigma0k0_res(double mandelstam_s)
pi- p -> Sigma0 K0 cross section parametrization, resonance contribution.
double kbar0n_elastic_background(double mandelstam_s)
Kbar0 n elastic background cross section parametrization Source: Buss:2011mx , B.3....
double piminusp_high_energy(double mandelstam_s)
pi-p total cross section at high energies
double np_elastic(double mandelstam_s)
np elastic cross section parametrization Source: Weil:2013mya , eq.
static void initialize(std::unordered_map< std::pair< uint64_t, uint64_t >, double, pair_hash > &ratios)
Calculate and store isospin ratios for K N -> K Delta reactions.
double piminusp_lambdak0_pdg(double mandelstam_s)
pi- p -> Lambda K0 cross section parametrization, PDG data.
static std::unique_ptr< InterpolateDataSpline > kminusp_elastic_res_interpolation
An interpolation that gets lazily filled using the KMINUSP_RES data.
const std::initializer_list< double > PIPLUSP_ELASTIC_P_LAB
PDG data on pi+ p elastic cross section: momentum in lab frame.
const std::initializer_list< double > KMINUSP_ELASTIC_SIG
PDG data on K- p elastic cross section: cross section.
double kminusn_piminussigma0(double sqrts)
K- n <-> pi- Sigma0 cross section parametrization Follow from the parametrization with the same stran...
double pp_total(double mandelstam_s)
pp total cross section parametrization Sources: low-p: Cugnon:1996kh highest-p: Buss:2011mx
const std::initializer_list< double > PIPLUSP_RES_SIG
Elastic π⁺N⁺ cross section contributions from decays.
static double piminusp_elastic_pdg(double mandelstam_s)
double kplusp_inelastic_background(double mandelstam_s)
K+ p inelastic background cross section parametrization Source: Buss:2011mx , B.3....
constexpr int Delta_pp
Δ⁺⁺.
constexpr T pow_int(const T base, unsigned const exponent)
Efficient template for calculating integer powers using squaring.
std::unordered_map< std::pair< uint64_t, uint64_t >, double, pair_hash > ratios_
Internal representation of isospin weights once calculated.
double piplusp_elastic(double mandelstam_s)
pi+p elastic cross section parametrization, PDG data.
static std::unique_ptr< InterpolateDataSpline > piminusp_elastic_res_interpolation
An interpolation that gets lazily filled using the PIMINUSP_RES data.
double xs_string_hard(double mandelstam_s, double xs_0, double e_0, double lambda_pow)
Utility function called by specific other parametrizations Parametrized hard scattering cross section...
double get_ratio(const ParticleType &a, const ParticleType &b, const ParticleType &c, const ParticleType &d) const
Return the isospin ratio of the given K N -> K Delta cross section.
const std::initializer_list< double > PIMINUSP_ELASTIC_P_LAB
PDG data on pi- p elastic cross section: momentum in lab frame.
double piplusp_sigmapluskplus_pdg(double mandelstam_s)
pi+ p to Sigma+ K+ cross section parametrization, PDG data.
static std::unique_ptr< InterpolateDataLinear< double > > piplusp_sigmapluskplus_interpolation
An interpolation that gets lazily filled using the PIPLUSP_SIGMAPLUSKPLUS_SIG data.
double piminusp_elastic(double mandelstam_s)
pi-p elastic cross section parametrization Source: GiBUU:parametrizationBarMes_HighEnergy....
double npbar_high_energy(double mandelstam_s)
npbar total cross section at high energies
double sigmaplussigmaminus_xi0n(double sqrts_sqrts0)
Sigma+ Sigma- <-> Xi0 n cross section parametrization Two hyperon exchange, based on effective model ...
static std::unique_ptr< InterpolateDataLinear< double > > kplusn_total_interpolation
An interpolation that gets lazily filled using the KPLUSN_TOT data.
const std::initializer_list< double > KPLUSN_TOT_PLAB
PDG data on K+ n total cross section: momentum in lab frame.
double Npi_string_hard(double mandelstam_s)
nucleon-pion hard scattering cross section (with partonic scattering)
double lambdasigmaminus_ximinusn(double sqrts_sqrts0)
Lambda Sigma- <-> Xi- n cross section parametrization Two hyperon exchange, based on effective model ...
double lambdasigma0_xi0n(double sqrts_sqrts0)
Lambda Sigma0 <-> Xi0 n cross section parametrization Two hyperon exchange, based on effective model ...
double kminusp_piminussigmaplus(double sqrts)
K- p <-> pi- Sigma+ cross section parametrization Taken from UrQMD (Graef:2014mra ).
double kminusp_kbar0n(double mandelstam_s)
K- p <-> Kbar0 n cross section parametrization.
constexpr double kaon_mass
Kaon mass in GeV.
std::int32_t code() const
const std::initializer_list< double > KPLUSN_TOT_SIG
PDG data on K+ n total cross section: cross section.
const std::initializer_list< double > PIPLUSP_SIGMAPLUSKPLUS_SIG
PDG data on pi+ p to Sigma+ K+ section: cross section.
constexpr double pion_mass
Pion mass in GeV.
const std::initializer_list< double > PIPLUSP_RES_SQRTS
Center-of-mass energy.
static std::unique_ptr< InterpolateDataLinear< double > > kminusp_elastic_interpolation
An interpolation that gets lazily filled using the KMINUSP_ELASTIC data.
double sigmaplussigmaminus_ximinusp(double sqrts_sqrts0)
Sigma+ Sigma- <-> Xi- p cross section parametrization Two hyperon exchange, based on effective model ...
double sigmaplussigmaminus_xi0p(double sqrts_sqrts0)
Sigma+ Sigma- <-> Xi0 p cross section parametrization Two hyperon exchange, based on effective model ...
const std::initializer_list< double > KMINUSP_ELASTIC_P_LAB
PDG data on K- p elastic cross section: momentum in lab frame.
constexpr uint64_t pack(int32_t x, int32_t y)
Pack two int32_t into an uint64_t.