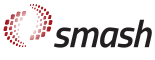 |
Version: SMASH-2.0
|
|
Go to the documentation of this file.
30 static constexpr
int LCollider = LogArea::Collider::id;
270 if (modus_cfg.
has_value({
"Calculation_Frame"})) {
271 frame_ = modus_cfg.
take({
"Calculation_Frame"});
275 if (modus_cfg.
has_value({
"Collisions_Within_Nucleus"})) {
282 bool same_file =
false;
287 }
else if (proj_cfg.
has_value({
"Custom"})) {
290 make_unique<CustomNucleus>(proj_cfg, params.
testparticles, same_file);
295 throw ColliderEmpty(
"Input Error: Projectile nucleus is empty.");
301 }
else if (targ_cfg.
has_value({
"Custom"})) {
303 make_unique<CustomNucleus>(targ_cfg, params.
testparticles, same_file);
308 throw ColliderEmpty(
"Input Error: Target nucleus is empty.");
312 if (modus_cfg.
has_value({
"Fermi_Motion"})) {
321 int energy_input = 0;
323 const double mass_target =
target_->mass();
325 const double mass_a =
327 const double mass_b =
target_->mass() /
target_->number_of_particles();
334 "Input Error: sqrt(s_NN) is not larger than masses:\n" +
335 std::to_string(
sqrt_s_NN_) +
" GeV <= " + std::to_string(mass_a) +
336 " GeV + " + std::to_string(mass_b) +
" GeV.");
340 mass_projec * mass_target / (mass_a * mass_b) +
341 mass_projec * mass_projec + mass_target * mass_target;
347 const double e_kin = modus_cfg.
take({
"E_Kin"});
351 "E_Kin must be nonnegative.");
355 mass_projec, mass_target);
361 const double p_lab = modus_cfg.
take({
"P_Lab"});
365 "P_Lab must be nonnegative.");
369 mass_projec, mass_target);
373 if (energy_input == 0) {
374 throw std::domain_error(
375 "Input Error: Non-existent collision energy. "
376 "Please provide one of Sqrtsnn/E_Kin/P_Lab.");
378 if (energy_input > 1) {
379 throw std::domain_error(
380 "Input Error: Redundant collision energy. "
381 "Please provide only one of Sqrtsnn/E_Kin/P_Lab.");
386 if (modus_cfg.
has_value({
"Impact",
"Value"})) {
392 if (modus_cfg.
has_value({
"Impact",
"Sample"})) {
395 if (!(modus_cfg.
has_value({
"Impact",
"Values"}) ||
396 modus_cfg.
has_value({
"Impact",
"Yields"}))) {
397 throw std::domain_error(
398 "Input Error: Need impact parameter spectrum for custom "
400 "Please provide Values and Yields.");
402 const std::vector<double> impacts =
403 modus_cfg.
take({
"Impact",
"Values"});
404 const std::vector<double> yields = modus_cfg.
take({
"Impact",
"Yields"});
405 if (impacts.size() != yields.size()) {
406 throw std::domain_error(
407 "Input Error: Need as many impact parameter values as yields. "
408 "Please make sure that Values and Yields have the same length.");
413 const auto imp_minmax =
414 std::minmax_element(impacts.begin(), impacts.end());
417 yield_max_ = *std::max_element(yields.begin(), yields.end());
420 if (modus_cfg.
has_value({
"Impact",
"Range"})) {
421 const std::array<double, 2> range = modus_cfg.
take({
"Impact",
"Range"});
425 if (modus_cfg.
has_value({
"Impact",
"Max"})) {
433 modus_cfg.
take({
"Impact",
"Random_Reaction_Plane"},
false);
435 if (modus_cfg.
has_value({
"Initial_Distance"})) {
444 return out <<
"-- Collider Modus:\n"
445 <<
"sqrt(S) (nucleus-nucleus) = "
454 Configuration &nucleus_cfg,
int ntest,
const std::string &nucleus_type) {
455 bool auto_deform = nucleus_cfg.
take({
"Deformed",
"Automatic"});
456 bool is_beta2 = nucleus_cfg.
has_value({
"Deformed",
"Beta_2"}) ?
true :
false;
457 bool is_beta4 = nucleus_cfg.
has_value({
"Deformed",
"Beta_4"}) ?
true :
false;
458 std::unique_ptr<DeformedNucleus> nucleus;
460 if ((auto_deform && (!is_beta2 && !is_beta4)) ||
461 (!auto_deform && (is_beta2 && is_beta4))) {
462 nucleus = make_unique<DeformedNucleus>(nucleus_cfg, ntest, auto_deform);
465 throw std::domain_error(
"Deformation of " + nucleus_type +
466 " nucleus not configured "
467 "properly, please check whether all necessary "
468 "parameters are set.");
489 if (v_a >= 1.0 || v_b >= 1.0) {
490 throw std::domain_error(
491 "Found velocity equal to or larger than 1 in "
492 "ColliderModus::initial_conditions.\nConsider using "
493 "the center of velocity reference frame.");
509 target_->generate_fermi_momenta();
519 throw std::domain_error(
"Invalid Fermi_Motion input.");
529 const double d_a = std::max(0.,
projectile_->get_diffusiveness());
530 const double d_b = std::max(0.,
target_->get_diffusiveness());
531 const double r_a =
projectile_->get_nuclear_radius();
532 const double r_b =
target_->get_nuclear_radius();
535 const double simulation_time = -dz / std::abs(v_a);
536 const double proj_z = -dz - std::sqrt(1.0 - v_a * v_a) * (r_a + d_a);
537 const double targ_z =
538 +dz * std::abs(v_b / v_a) + std::sqrt(1.0 - v_b * v_b) * (r_b + d_b);
548 target_->copy_particles(particles);
550 return simulation_time;
559 p.set_3position(pos);
560 p.set_3momentum(mom);
577 double probability_random = 1;
578 double probability = 0;
580 while (probability_random > probability) {
582 probability = (*impact_interpolation_)(b) /
yield_max_;
583 assert(probability < 1.);
608 v_a =
pCM / std::sqrt(m_a * m_a +
pCM *
pCM);
609 v_b = -
pCM / std::sqrt(m_b * m_b +
pCM *
pCM);
615 throw std::domain_error(
616 "Invalid reference frame in "
617 "ColliderModus::get_velocities.");
619 return std::make_pair(v_a, v_b);
623 const std::string &file_name) {
625 if (file_directory.back() ==
'/') {
626 return file_directory + file_name;
628 return file_directory +
'/' + file_name;
637 if (!targ_config.
has_value({
"Custom"})) {
640 std::string projectile_file_directory =
641 proj_config.
read({
"Custom",
"File_Directory"});
642 std::string target_file_directory =
643 targ_config.
read({
"Custom",
"File_Directory"});
644 std::string projectile_file_name = proj_config.
read({
"Custom",
"File_Name"});
645 std::string target_file_name = targ_config.
read({
"Custom",
"File_Name"});
647 std::string proj_path =
649 std::string targ_path =
651 if (proj_path == targ_path) {
std::unique_ptr< InterpolateDataLinear< double > > impact_interpolation_
Pointer to the impact parameter interpolation.
double initial_z_displacement_
Initial z-displacement of nuclei.
double imp_min_
Minimum value of impact parameter.
std::string custom_file_path(const std::string &file_directory, const std::string &file_name)
Creates full path string consisting of file_directory and file_name Needed to initialize a customnucl...
Sample from areal / quadratic distribution.
bool cll_in_nucleus_
An option to accept first collisions within the same nucleus.
FermiMotion fermi_motion_
An option to include Fermi motion ("off", "on", "frozen")
bool random_reaction_plane_
Whether the reaction plane should be randomized.
Value read(std::initializer_list< const char * > keys) const
Additional interface for SMASH to read configuration values without removing them.
Sampling sampling_
Method used for sampling of impact parameter.
double sqrt_s_NN_
Center-of-mass energy of a nucleon-nucleon collision.
double s_from_plab(double plab, double m_P, double m_T)
Convert p_lab to Mandelstam-s for a fixed-target setup, with a projectile of mass m_P and momentum pl...
std::unique_ptr< Nucleus > target_
Target.
double fixed_target_projectile_v(double s, double ma, double mb)
bool same_inputfile(Configuration &proj_config, Configuration &targ_config)
Checks if target and projectile are read from the same external file if they are both initialized as ...
std::ostream & operator<<(std::ostream &out, const ActionPtr &action)
void sample_impact()
Sample impact parameter.
double s_from_Ekin(double e_kin, double m_P, double m_T)
Convert E_kin to Mandelstam-s for a fixed-target setup, with a projectile of mass m_P and a kinetic e...
bool has_value(std::initializer_list< const char * > keys) const
Returns whether there is a non-empty value behind the requested keys.
double initial_conditions(Particles *particles, const ExperimentParameters ¶meters)
Generates initial state of the particles in the system.
void rotate_around_z(double theta)
Rotate the vector around the z axis by the given angle theta.
Use fermi motion without potentials.
std::array< einhard::Logger<>, std::tuple_size< LogArea::AreaTuple >::value > logg
An array that stores all pre-configured Logger objects.
Sample from custom, user-defined distribution.
double velocity_projectile_
Beam velocity of the projectile.
T pCM(const T sqrts, const T mass_a, const T mass_b) noexcept
double center_of_velocity_v(double s, double ma, double mb)
Interface to the SMASH configuration files.
double imp_max_
Maximum value of impact parameter.
FormattingHelper< T > format(const T &value, const char *unit, int width=-1, int precision=-1)
Acts as a stream modifier for std::ostream to output an object with an optional suffix string and wit...
double total_s_
Center-of-mass energy squared of the nucleus-nucleus collision.
CalculationFrame frame_
Reference frame for the system, as specified from config.
static constexpr int LCollider
double yield_max_
Maximum value of yield. Needed for custom impact parameter sampling.
double impact_
Impact parameter.
Sample from uniform distribution.
Use fermi motion in combination with potentials.
std::unique_ptr< Nucleus > projectile_
Projectile.
double velocity_target_
Beam velocity of the target.
Value take(std::initializer_list< const char * > keys)
The default interface for SMASH to read configuration values.
Helper structure for Experiment.
static std::unique_ptr< DeformedNucleus > create_deformed_nucleus(Configuration &nucleus_cfg, const int ntest, const std::string &nucleus_type)
Configure Deformed Nucleus.
int testparticles
Number of test particle.
void rotate_reaction_plane(double phi, Particles *particles)
Rotate the reaction plane about the angle phi.
std::pair< double, double > get_velocities(double mandelstam_s, double m_a, double m_b)
Get the frame dependent velocity for each nucleus, using the current reference frame.
ColliderModus(Configuration modus_config, const ExperimentParameters ¶meters)
Constructor.
T pCM_from_s(const T s, const T mass_a, const T mass_b) noexcept