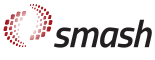 |
Version: SMASH-2.0
|
|
Go to the documentation of this file.
10 #ifndef SRC_INCLUDE_SMASH_CROSSSECTIONS_H_
11 #define SRC_INCLUDE_SMASH_CROSSSECTIONS_H_
25 namespace transit_high_energy {
75 CrossSections(
const ParticleList& incoming_particles,
const double sqrt_s,
76 const std::pair<FourVector, FourVector> potentials);
105 double elastic_parameter,
bool two_to_one_switch,
108 bool strings_switch,
bool use_AQM,
bool strings_with_probability,
110 double scale_xs,
double additional_el_xs)
const;
116 static double sum_xs_of(
const CollisionBranchList& list) {
118 for (
auto& proc : list) {
119 xs_sum += proc->weight();
142 CollisionBranchPtr
elastic(
double elast_par,
bool use_AQM,
double add_el_xs,
143 double scale_xs)
const;
160 CollisionBranchList
two_to_one(
const bool prevent_dprime_form)
const;
174 double cm_momentum_sqr)
const;
249 const double scale_xs)
const;
330 bool use_transition_probability,
bool use_AQM,
331 bool treat_nnbar_with_strings)
const;
339 const double region_upper)
const;
359 double nn_el()
const;
383 double nk_el()
const;
393 CollisionBranchList
npi_yk()
const;
484 static double xs_dn_dprimen(
const double sqrts,
const double cm_mom,
536 template <
class IntegrationMethod>
538 const ParticleTypePtrList& type_res_1,
539 const ParticleTypePtrList& type_res_2,
540 const IntegrationMethod integrator)
const;
575 template <
typename F>
576 void add_channel(CollisionBranchList& process_list, F&& get_xsection,
579 const double sqrt_s_min =
582 double scale_B = 0.0;
583 double scale_I3 = 0.0;
584 bool is_below_threshold;
588 incoming_momentum +=
p.momentum();
599 is_below_threshold = (incoming_momentum +
potentials_.first * scale_B +
601 .abs() <= sqrt_s_min;
603 is_below_threshold = (sqrts <= sqrt_s_min);
605 if (is_below_threshold) {
608 const auto xsection = get_xsection();
610 process_list.push_back(make_unique<CollisionBranch>(
618 #endif // SRC_INCLUDE_SMASH_CROSSSECTIONS_H_
CollisionBranchList bar_bar_to_nuc_nuc(const bool is_anti_particles) const
Calculate cross sections for resonance absorption (i.e.
double min_mass_spectral() const
The minimum mass of the resonance, where the spectral function is non-zero.
static double xs_dpi_dprimepi(const double sqrts, const double cm_mom, ParticleTypePtr produced_nucleus, const ParticleType &type_pi)
Parametrized cross section for πd→ πd' (mockup for πd→ πnp), πd̅→ πd̅' and reverse,...
const ParticleList incoming_particles_
List with data of scattering particles.
CollisionBranchList generate_collision_list(double elastic_parameter, bool two_to_one_switch, ReactionsBitSet included_2to2, MultiParticleReactionsBitSet included_multi, double low_snn_cut, bool strings_switch, bool use_AQM, bool strings_with_probability, NNbarTreatment nnbar_treatment, StringProcess *string_process, double scale_xs, double additional_el_xs) const
Generate a list of all possible collisions between the incoming particles with the given c....
static std::pair< double, int > force_scale(const ParticleType &data)
Evaluates the scaling factor of the forces acting on the particles.
CollisionBranchList two_to_three() const
Find all 2->3 processes for the given scattering.
const std::array< double, 2 > sqrts_range_Npi
transition range in N-pi collisions
double formation(const ParticleType &type_resonance, double cm_momentum_sqr) const
Return the 2-to-1 resonance production cross section for a given resonance.
double npi_el() const
Determine the elastic cross section for a nucleon-pion (Npi) collision.
static double sum_xs_of(const CollisionBranchList &list)
Helper function: Sum all cross sections of the given process list.
2->2 inelastic scattering
std::bitset< 10 > ReactionsBitSet
Container for the 2 to 2 reactions in the code.
double elastic_parametrization(bool use_AQM) const
Choose the appropriate parametrizations for given incoming particles and return the (parametrized) el...
T pCM(const T sqrts, const T mass_a, const T mass_b) noexcept
constexpr double really_small
Numerical error tolerance.
static double nn_to_resonance_matrix_element(double sqrts, const ParticleType &type_a, const ParticleType &type_b, const int twoI)
Scattering matrix amplitude squared (divided by 16π) for resonance production processes like NN → NR ...
std::bitset< 2 > MultiParticleReactionsBitSet
Container for the 2 to 2 reactions in the code.
String excitation processes used in SMASH.
const double pipi_offset
Constant offset as to where to turn on the strings and elastic processes for pi pi reactions (this is...
CollisionBranchList two_to_one(const bool prevent_dprime_form) const
Find all resonances that can be produced in a 2->1 collision of the two input particles and the produ...
CollisionBranchList nk_xx(ReactionsBitSet included_2to2) const
Find all inelastic 2->2 background processes for Nucleon-Kaon (NK) Scattering.
CollisionBranchList npi_yk() const
Find all processes for Nucleon-Pion to Hyperon-Kaon Scattering.
double string_probability(bool strings_switch, bool use_transition_probability, bool use_AQM, bool treat_nnbar_with_strings) const
const double sqrt_s_
Total energy in the center-of-mass frame.
CollisionBranchList find_nn_xsection_from_type(const ParticleTypePtrList &type_res_1, const ParticleTypePtrList &type_res_2, const IntegrationMethod integrator) const
Utility function to avoid code replication in nn_xx().
double isospin3_rel() const
const double sqrts_add_lower
constant for the lower end of transition region in the case of AQM this is added to the sum of masses
CollisionBranchList bb_xx_except_nn(ReactionsBitSet included_2to2) const
Find all inelastic 2->2 processes for Baryon-Baryon (BB) Scattering except the more specific Nucleon-...
double cm_momentum() const
Determine the momenta of the incoming particles in the center-of-mass system.
The cross section class assembels everything that is needed to calculate the cross section and return...
const double sqrts_range
constant for the range of transition region in the case of AQM this is added to the sum of masses + s...
double high_energy() const
Determine the parametrized total cross section at high energies for the given collision,...
CollisionBranchList deltak_xx(ReactionsBitSet included_2to2) const
Find all inelastic 2->2 processes for Delta-Kaon (DeltaK) Scattering.
CollisionBranchList dn_xx(ReactionsBitSet included_2to2) const
Find all inelastic 2->2 processes involving Nucleon and (anti-) Deuteron (dN), specifically Nd → Nd',...
const bool is_BBbar_pair_
Whether incoming particles are a baryon-antibaryon pair.
CollisionBranchList rare_two_to_two() const
Find all 2->2 processes which are suppressed at high energies when strings are turned on with probabi...
CollisionBranchList two_to_two(ReactionsBitSet included_2to2) const
Find all inelastic 2->2 processes for the given scattering.
CollisionBranchList NNbar_creation() const
Determine the cross section for NNbar creation, which is given by detailed balance from the reverse r...
CollisionBranchList ypi_xx(ReactionsBitSet included_2to2) const
Find all inelastic 2->2 processes for Hyperon-Pion (Ypi) Scattering.
const double KN_offset
Constant offset as to where to shift from 2to2 to string processes (in GeV) in the case of KN reactio...
CollisionBranchPtr NNbar_annihilation(const double current_xs, const double scale_xs) const
Determine the cross section for NNbar annihilation, which is given by the difference between the para...
double nk_el() const
Determine the elastic cross section for a nucleon-kaon (NK) collision.
NNbarTreatment
Treatment of N Nbar Annihilation.
CollisionBranchList dpi_xx(ReactionsBitSet included_2to2) const
Find all inelastic 2->2 processes involving Pion and (anti-) Deuteron (dpi), specifically dπ→ NN,...
double nn_el() const
Determine the (parametrized) elastic cross section for a nucleon-nucleon (NN) collision.
CollisionBranchList string_excitation(double total_string_xs, StringProcess *string_process, bool use_AQM) const
Determine the cross section for string excitations, which is given by the difference between the para...
Potentials * pot_pointer
Pointer to a Potential class.
CrossSections(const ParticleList &incoming_particles, const double sqrt_s, const std::pair< FourVector, FourVector > potentials)
Construct CrossSections instance.
CollisionBranchList nn_xx(ReactionsBitSet included_2to2) const
Find all inelastic 2->2 processes for Nucelon-Nucelon Scattering.
const std::array< double, 2 > sqrts_range_NN
transition range in N-N collisions: Tuned to reproduce experimental exclusive cross section data,...
static double xs_dn_dprimen(const double sqrts, const double cm_mom, ParticleTypePtr produced_nucleus, const ParticleType &type_nucleus, const ParticleType &type_N)
Parametrized cross section for Nd → Nd', N̅d → N̅d', N̅d̅→ N̅d̅', Nd̅→ Nd̅' and reverse (e....
const std::pair< FourVector, FourVector > potentials_
Potentials at the interacting point.
double string_hard_cross_section() const
Determine the (parametrized) hard non-diffractive string cross section for this collision.
CollisionBranchPtr elastic(double elast_par, bool use_AQM, double add_el_xs, double scale_xs) const
Determine the elastic cross section for this collision.
double probability_transit_high(const double region_lower, const double region_upper) const
void add_channel(CollisionBranchList &process_list, F &&get_xsection, double sqrts, const ParticleType &type_a, const ParticleType &type_b) const
Helper function: Add a 2-to-2 channel to a collision branch list given a cross section.
static double two_to_three_xs(const ParticleType &type_in1, const ParticleType &type_in2, double sqrts)
Determine 2->3 cross section for the scattering of the given particle types.