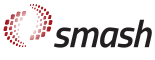 |
Version: SMASH-2.0
|
|
Go to the documentation of this file.
108 :
Nucleus(particle_list, nTest) {}
111 bool auto_deformation)
113 if (auto_deformation) {
119 if (config.
has_value({
"Deformed",
"Orientation"})) {
143 return a_direction.
threevec() * a_radius;
151 const std::map<int, std::string> A_map = {
152 {238,
"Uranium"}, {208,
"Lead"}, {197,
"Gold"}, {63,
"Copper"}};
153 const std::map<std::string, std::string> Z_map = {
154 {
"Uranium",
"92"}, {
"Lead",
"82"}, {
"Gold",
"79"}, {
"Copper",
"29"}};
194 }
else if (Z == 44) {
198 throw std::domain_error(
199 "Number of protons for nuclei with mass number A = 96 does not "
200 "match that of Zirconium or Ruthenium. The deformation parameters "
201 "for additional isobars are currently not implemented."
202 " Please specify at least \"Beta_2\" and \"Beta_4\" "
203 "manually and set \"Automatic: False.\" ");
207 throw std::domain_error(
208 "Mass number not listed for automatically setting deformation "
209 "parameters. Please specify at least \"Beta_2\" and \"Beta_4\" "
210 "manually and set \"Automatic: False.\" ");
213 throw std::domain_error(
"Mass number is listed under " + A_map.at(A) +
219 Z_map.at(A_map.at(A)) +
221 "Please specify at least \"Beta_2\" and \"Beta_4\" "
222 "manually and set \"Automatic: False.\" ");
229 if (config.
has_value({
"Deformed",
"Beta_2"})) {
230 set_beta_2(static_cast<double>(config.
take({
"Deformed",
"Beta_2"})));
232 if (config.
has_value({
"Deformed",
"Beta_4"})) {
233 set_beta_4(static_cast<double>(config.
take({
"Deformed",
"Beta_4"})));
242 if (orientation_config.
has_value({
"Theta"})) {
243 if (orientation_config.
has_value({
"Random_Rotation"}) &&
244 orientation_config.
take({
"Random_Rotation"})) {
245 throw std::domain_error(
246 "Random rotation of nuclei is activated although"
247 " theta is provided. Please specify only either of them. ");
253 if (orientation_config.
has_value({
"Phi"})) {
254 if (orientation_config.
has_value({
"Random_Rotation"}) &&
255 orientation_config.
take({
"Random_Rotation"})) {
256 throw std::domain_error(
257 "Random rotation of nuclei is activated although"
258 " phi is provided. Please specify only either of them. ");
261 static_cast<double>(orientation_config.
take({
"Phi"})));
265 if (orientation_config.
take({
"Random_Rotation"},
false)) {
278 for (
auto &particle : *
this) {
283 ThreeVector three_pos = particle.position().threevec();
286 particle.set_3position(three_pos);
292 return (1. / 4) * std::sqrt(5 / M_PI) * (3. * (cosx * cosx) - 1);
294 return (3. / 16) * std::sqrt(1 / M_PI) *
295 (35. * (cosx * cosx) * (cosx * cosx) - 30. * (cosx * cosx) + 3);
297 throw std::domain_error(
298 "Not a valid angular momentum quantum number in y_l_0.");
void random_euler_angles()
Randomly generate Euler angles.
A nucleus is a collection of particles that are initialized, before the beginning of the simulation a...
size_t number_of_protons() const
Number of physical protons in the nucleus:
double get_diffusiveness() const
void rotate(double phi, double theta, double psi)
Rotate vector by the given Euler angles phi, theta, psi.
double get_saturation_density() const
void distribute_isotropically()
Populate the object with a new direction.
double euler_theta_
Euler angel theta.
bool has_value(std::initializer_list< const char * > keys) const
Returns whether there is a non-empty value behind the requested keys.
size_t number_of_particles() const
Number of physical particles in the nucleus:
Interface to the SMASH configuration files.
double y_l_0(int l, double cosx)
Spherical harmonics Y_2_0 and Y_4_0.
ThreeVector threevec() const
double euler_phi_
Euler angel phi.
Value take(std::initializer_list< const char * > keys)
The default interface for SMASH to read configuration values.
double get_nuclear_radius() const
Angles provides a common interface for generating directions: i.e., two angles that should be interpr...