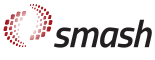 |
Version: SMASH-2.0
|
|
Go to the documentation of this file.
7 #ifndef SRC_INCLUDE_SMASH_NUCLEUS_H_
8 #define SRC_INCLUDE_SMASH_NUCLEUS_H_
51 Nucleus(
const std::map<PdgCode, int> &particle_list,
int nTest);
117 void boost(
double beta_scalar);
140 void shift(
double z_offset,
double x_offset,
double simulation_time);
170 "Number of test particles and test particles"
171 "per particle are incompatible.");
184 size_t proton_counter = 0;
188 if (particle.type().pdgcode() ==
pdg::p) {
197 "Number of test protons and test particles"
198 "per proton are incompatible.");
218 p->set_4position(
p->position() - centerpoint);
233 using std::length_error::length_error;
273 inline std::vector<ParticleData>::iterator
begin() {
277 inline std::vector<ParticleData>::iterator
end() {
return particles_.end(); }
279 inline std::vector<ParticleData>::const_iterator
cbegin()
const {
283 inline std::vector<ParticleData>::const_iterator
cend()
const {
323 return (1.12 * std::pow(A, 1.0 / 3.0) - 0.86 * std::pow(A, -1.0 / 3.0));
345 #endif // SRC_INCLUDE_SMASH_NUCLEUS_H_
void random_euler_angles()
Randomly generate Euler angles.
A nucleus is a collection of particles that are initialized, before the beginning of the simulation a...
double diffusiveness_
Diffusiveness of Woods-Saxon distribution of this nucleus in fm (for diffusiveness_ == 0,...
virtual ~Nucleus()=default
void fill_from_list(const std::map< PdgCode, int > &particle_list, int testparticles)
Adds particles from a map PDG code => Number_of_particles_with_that_PDG_code to the nucleus.
std::vector< ParticleData >::const_iterator cbegin() const
For const iterators over the particle list:
Nucleus()=default
default constructor
virtual void generate_fermi_momenta()
Generates momenta according to Fermi motion for the nucleons.
size_t number_of_protons() const
Number of physical protons in the nucleus:
double get_diffusiveness() const
double default_nuclear_radius()
Default nuclear radius calculated as:
std::vector< ParticleData >::iterator begin()
For iterators over the particle list:
double get_saturation_density() const
double woods_saxon(double x)
Woods-Saxon distribution.
double nuclear_radius_
Nuclear radius of this nucleus.
constexpr double nuclear_density
Ground state density of symmetric nuclear matter [fm^-3].
double euler_theta_
Euler angel theta.
void copy_particles(Particles *particles)
Copies the particles from this nucleus into the particle list.
void align_center()
Shifts the nucleus so that its center is at (0,0,0)
virtual void arrange_nucleons()
Sets the positions of the nucleons inside a nucleus.
size_t number_of_particles() const
Number of physical particles in the nucleus:
virtual void set_parameters_automatic()
Sets the deformation parameters of the Woods-Saxon distribution according to the current mass number.
std::vector< ParticleData > particles_
Particles associated with this nucleus.
Interface to the SMASH configuration files.
size_t testparticles_
Number of testparticles per physical particle.
double euler_psi_
Euler angel psi.
void shift(double z_offset, double x_offset, double simulation_time)
Shifts the nucleus to correct impact parameter and z displacement.
friend std::ostream & operator<<(std::ostream &, const Nucleus &)
void set_nuclear_radius(double rad)
Sets the nuclear radius.
virtual ThreeVector distribute_nucleon()
The distribution of return values from this function is according to a spherically symmetric Woods-Sa...
std::vector< ParticleData >::iterator end()
For iterators over the particle list:
FourVector center() const
Calculate geometrical center of the nucleus.
virtual void rotate()
Rotates the nucleus.
virtual void set_parameters_from_config(Configuration &config)
Sets the parameters of the Woods-Saxon according to manually added values in the configuration file.
double proton_radius_
Single proton radius in fm.
void set_diffusiveness(double diffuse)
Sets the diffusiveness of the nucleus.
size_t size() const
Number of numerical (=test-)particles in the nucleus:
double saturation_density_
Saturation density of this nucleus.
double euler_phi_
Euler angel phi.
void boost(double beta_scalar)
Boosts the nuclei into the computational frame, such that the nucleons have the appropriate momentum ...
double get_nuclear_radius() const
virtual double nucleon_density(double r, double) const
Return the Woods-Saxon probability density for the given position.
std::vector< ParticleData >::const_iterator cend() const
For const iterators over the particle list:
void set_saturation_density(double density)
Sets the saturation density of the nucleus.