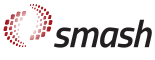 |
Version: SMASH-2.0
|
|
Go to the documentation of this file.
21 :
Action(in_plist, time), total_probability_(0.) {}
33 double xsec_scaling = 1.0;
35 xsec_scaling *= in_part.xsec_scaling_factor();
41 double xsec_scaling = 1.0;
43 xsec_scaling *= in_part.xsec_scaling_factor();
49 double dt,
const double gcell_vol,
80 int sym_factor_in = 1;
91 *type_eta_prime, dt, gcell_vol,
111 if (type_deuteron && type_anti_deuteron) {
117 (pdg_a ==
pdg::p && pdg_b ==
pdg::n && pdg_c.is_pion()) ||
118 (pdg_a ==
pdg::n && pdg_b ==
pdg::p && pdg_c.is_pion())) {
120 ParticleList::iterator it = std::find_if(
125 const double spin_degn =
130 type_pi, *type_deuteron,
141 (pdg_a == -
pdg::p && pdg_b == -
pdg::n && pdg_c.is_pion()) ||
142 (pdg_a == -
pdg::n && pdg_b == -
pdg::p && pdg_c.is_pion())) {
144 ParticleList::iterator it = std::find_if(
149 const double spin_degn =
154 type_pi, *type_anti_deuteron,
156 gcell_vol, spin_degn),
165 (pdg_a ==
pdg::p && pdg_b ==
pdg::n && pdg_c.is_nucleon()) ||
166 (pdg_a ==
pdg::n && pdg_b ==
pdg::p && pdg_c.is_nucleon())) {
167 int symmetry_factor = 1;
169 ParticleList::iterator it =
172 return x.pdgcode().antiparticle_sign() == -1;
179 if (pdg_a == pdg_b) {
188 const double spin_degn =
193 type_N, *type_deuteron,
195 symmetry_factor * spin_degn),
204 (pdg_a == -
pdg::p && pdg_b == -
pdg::n && pdg_c.is_nucleon()) ||
205 (pdg_a == -
pdg::n && pdg_b == -
pdg::p && pdg_c.is_nucleon())) {
206 int symmetry_factor = 1;
208 ParticleList::iterator it =
211 return x.pdgcode().antiparticle_sign() == 1;
218 if (pdg_a == pdg_b) {
227 const double spin_degn =
232 type_N, *type_anti_deuteron,
234 gcell_vol, symmetry_factor * spin_degn),
266 "ScatterActionMulti::generate_final_state: Invalid process type " +
267 std::to_string(static_cast<int>(
process_type_)) +
" was requested.");
275 new_particle.boost_momentum(
278 new_particle.set_4position(middle_point);
287 const double lower_bound = (m1 + m2) * (m1 + m2);
288 const double upper_bound = (sqrts - m3) * (sqrts - m3);
289 const auto result =
integrate(lower_bound, upper_bound, [&](
double m12_sqr) {
290 const double m12 = std::sqrt(m12_sqr);
291 const double e2_star = (m12_sqr - m1 * m1 + m2 * m2) / (2 * m12);
292 const double e3_star = (sqrts * sqrts - m12_sqr - m3 * m3) / (2 * m12);
293 const double m23_sqr_min =
294 (e2_star + e3_star) * (e2_star + e3_star) -
295 std::pow(std::sqrt(e2_star * e2_star - m2 * m2) +
296 std::sqrt(e3_star * e3_star - m3 * m3),
298 const double m23_sqr_max =
299 (e2_star + e3_star) * (e2_star + e3_star) -
300 std::pow(std::sqrt(e2_star * e2_star - m2 * m2) -
301 std::sqrt(e3_star * e3_star - m3 * m3),
303 return m23_sqr_max - m23_sqr_min;
310 const ParticleType& type_out,
double dt,
const double gcell_vol,
311 const int degen_factor)
const {
315 const double sqrts =
sqrt_s();
322 const double ph_sp_3 =
323 1. / (8 * M_PI * M_PI * M_PI) * 1. / (16 * sqrts * sqrts) * I_3;
327 return dt / (gcell_vol * gcell_vol) * M_PI / (4. * e1 * e2 * e3) *
328 gamma_decay / ph_sp_3 * spec_f_val * std::pow(
hbarc, 5.0) *
334 const double gcell_vol,
const double degen_factor)
const {
338 const double m4 = type_out1.
mass();
339 const double m5 = type_out2.
mass();
341 const double sqrts =
sqrt_s();
344 const double lamb =
lambda_tilde(sqrts * sqrts, m4 * m4, m5 * m5);
347 const double ph_sp_3 =
348 1. / (8 * M_PI * M_PI * M_PI) * 1. / (16 * sqrts * sqrts) * I_3;
350 return dt / (gcell_vol * gcell_vol) * 1. / (4. * e1 * e2 * e3) * lamb /
351 (ph_sp_3 * 8 * M_PI * sqrts * sqrts) * xs * std::pow(
hbarc, 5.0) *
359 "Incorrect number of particles in final state: ";
390 (pdg_a != pdg_b && pdg_b != pdg_c && pdg_c != pdg_a);
416 out <<
" (not performed)";
ParticleList particle_list() const
static double lambda_tilde(double a, double b, double c)
Little helper function that calculates the lambda function (sometimes written with a tilde to better ...
ParticleList incoming_particles_
List with data of incoming particles.
void assign_formation_time_to_outgoing_particles()
Assign the formation time to the outgoing particles.
void sample_2body_phasespace()
Sample the full 2-body phase-space (masses, momenta, angles) in the center-of-mass frame for the fina...
void add_reactions(CollisionBranchList pv)
Add several new reaction channels at once.
static PdgCode from_decimal(const int pdgcode_decimal)
Construct PDG code from decimal number.
unsigned int spin_degeneracy() const
void generate_final_state() override
Generate the final-state of the multi-particle scattering process.
A C++ interface for numerical integration in one dimension with the GSL CQUAD integration functions.
PdgCode pdgcode() const
Get the pdgcode of the particle.
double spectral_function(double m) const
Full spectral function of the resonance (relativistic Breit-Wigner distribution with mass-dependent ...
void format_debug_output(std::ostream &out) const override
constexpr double hbarc
GeV <-> fm conversion factor.
constexpr double gev2_mb
GeV^-2 <-> mb conversion factor.
std::array< einhard::Logger<>, std::tuple_size< LogArea::AreaTuple >::value > logg
An array that stores all pre-configured Logger objects.
void add_possible_reactions(double dt, const double gcell_vol, const MultiParticleReactionsBitSet incl_multi)
Add all possible multi-particle reactions for the given incoming particles.
double probability_three_to_one(const ParticleType &type_out, double dt, const double gcell_vol, const int degen_factor=1) const
Calculate the probability for a 3m-to-1 reaction according to the stochastic collision criterion (e....
std::bitset< 2 > MultiParticleReactionsBitSet
Container for the 2 to 2 reactions in the code.
unsigned int spin_degeneracy() const
double calculate_I3(const double sqrts) const
Calculate the integration necessary for the three-body phase space.
multi particle scattering
static Integrator integrate
void add_reaction(CollisionBranchPtr p)
Add a new reaction channel.
double partial_probability_
Partial probability of the chosen outgoing channel.
constexpr int decimal_antid
Anti-deuteron in decimal digits.
ProcessType process_type_
type of process
ProcessType get_type() const override
double react_degen_factor(const int spin_factor_inc, const int spin_degen_out1, const int spin_degen_out2) const
Determine the spin degeneracy factor ( ) for the 3->2 reaction.
constexpr int decimal_d
Deuteron in decimal digits.
FourVector total_momentum_of_outgoing_particles() const
Calculate the total kinetic momentum of the outgoing particles.
static constexpr int LScatterActionMulti
static const ParticleTypePtr try_find(PdgCode pdgcode)
Returns the ParticleTypePtr for the given pdgcode.
ParticleList outgoing_particles_
Initially this stores only the PDG codes of final-state particles.
ScatterActionMulti(const ParticleList &in_plist, double time)
Construct a ScatterActionMulti object.
double sqrt_s() const
Determine the total energy in the center-of-mass frame [GeV].
double get_partial_width(const double m, const ParticleTypePtrList dlist) const
Get the mass-dependent partial width of a resonance with mass m, decaying into two given daughter par...
bool two_pions_eta(const ParticleData &data_a, const ParticleData &data_b, const ParticleData &data_c) const
Check wether the three incoming particles are π⁺,π⁻,η or π⁰,π⁰,η in any order.
FourVector get_interaction_point() const
Get the interaction point.
double total_probability_
Total probability of reaction.
void three_to_two()
Perform a 3->2 process.
double probability_three_to_two(const ParticleType &type_out1, const ParticleType &type_out2, double dt, const double gcell_vol, const double degen_factor=1.0) const
Calculate the probability for a 3-to-2 reaction according to the stochastic collision criterion (simi...
bool three_different_pions(const ParticleData &data_a, const ParticleData &data_b, const ParticleData &data_c) const
Check wether the three incoming particles are π⁺,π⁻,π⁰ in any order.
double get_total_weight() const override
Get the total probability for the reaction (scaled with the cross section scaling factors of the inco...
double get_partial_weight() const override
Get the partial probability for the chosen channel (scaled with the cross section scaling factors of ...
CollisionBranchList reaction_channels_
List of possible collisions.
void annihilation()
Perform a n->1 annihilation process.
static double two_to_three_xs(const ParticleType &type_in1, const ParticleType &type_in2, double sqrts)
Determine 2->3 cross section for the scattering of the given particle types.