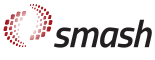 |
Version: SMASH-2.0
|
|
Go to the documentation of this file.
7 #ifndef SRC_INCLUDE_SMASH_SPHEREMODUS_H_
8 #define SRC_INCLUDE_SMASH_SPHEREMODUS_H_
143 #endif // SRC_INCLUDE_SMASH_SPHEREMODUS_H_
const double mus_
Strange chemical potential for thermal initialization; only used if use_thermal_ is true.
double initial_conditions(Particles *particles, const ExperimentParameters ¶meters)
Generates initial state of the particles in the system according to specified parameters: number of p...
const std::map< PdgCode, int > init_multipl_
Particle multiplicities at initialization; required if use_thermal_ is false.
const bool insert_jet_
Whether to insert a single high energy particle at the center of the expanding sphere (0,...
const bool account_for_resonance_widths_
In case of thermal initialization: true – account for resonance spectral functions,...
double radius_
Sphere radius (in fm/c)
const double start_time_
Starting time for the Sphere.
const SphereInitialCondition init_distr_
Initialization scheme for momenta in the sphere; used for expanding metric setup.
Interface to the SMASH configuration files.
friend std::ostream & operator<<(std::ostream &, const SphereModus &)
const double mub_
Baryon chemical potential for thermal initialization; only used if use_thermal_ is true.
double sphere_temperature_
Temperature for momentum distribution (in GeV)
const PdgCode jet_pdg_
Pdg of the particle to use as a jet; necessary if insert_jet_ is true, unused otherwise.
SphereInitialCondition
Initial condition for a particle in a sphere.
std::map< PdgCode, double > average_multipl_
Average multiplicities in case of thermal initialization.
const double jet_mom_
Initial momentum of the jet particle; only used if insert_jet_ is true.
SphereModus(Configuration modus_config, const ExperimentParameters ¶meters)
Constructor.
Helper structure for Experiment.
const bool use_thermal_
Whether to use a thermal initialization for all particles instead of specific numbers.