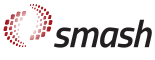 |
Version: SMASH-2.0
|
|
Go to the documentation of this file.
14 #include <boost/filesystem.hpp>
18 #include "smash/config.h"
135 const std::string &mode,
136 const std::string &name,
137 bool extended_format)
138 :
OutputInterface(name), file_{path, mode}, extended_(extended_format) {
139 std::fwrite(
"SMSH", 4, 1, file_.get());
140 write(format_version_);
141 std::uint16_t format_variant = static_cast<uint16_t>(extended_);
142 write(format_variant);
143 write(VERSION_MAJOR);
148 std::fwrite(&c,
sizeof(
char), 1,
file_.
get());
152 const auto size = boost::numeric_cast<uint32_t>(s.size());
153 std::fwrite(&size,
sizeof(std::uint32_t), 1,
file_.
get());
154 std::fwrite(s.c_str(), s.size(), 1,
file_.
get());
158 std::fwrite(&x,
sizeof(x), 1,
file_.
get());
166 for (
const auto &
p : particles) {
172 for (
const auto &
p : particles) {
179 double mass =
p.effective_mass();
180 std::fwrite(&mass,
sizeof(mass), 1,
file_.
get());
182 write(
p.pdgcode().get_decimal());
186 const auto history =
p.get_history();
187 write(history.collisions_per_particle);
188 write(
p.formation_time());
189 write(
p.xsec_scaling_factor());
190 write(history.id_process);
191 write(static_cast<int32_t>(history.process_type));
192 write(history.time_last_collision);
193 write(history.p1.get_decimal());
194 write(history.p2.get_decimal());
202 path / ((name ==
"Collisions" ?
"collisions_binary" : name) +
".bin"),
203 "wb", name, out_par.get_coll_extended(name)),
204 print_start_end_(out_par.coll_printstartend) {}
208 const char pchar =
'p';
210 std::fwrite(&pchar,
sizeof(
char), 1,
file_.
get());
217 const int32_t event_number,
219 const char pchar =
'p';
221 std::fwrite(&pchar,
sizeof(
char), 1,
file_.
get());
227 const char fchar =
'f';
228 std::fwrite(&fchar,
sizeof(
char), 1,
file_.
get());
231 const char empty =
event.empty_event;
239 const double density) {
240 const char ichar =
'i';
241 std::fwrite(&ichar,
sizeof(
char), 1,
file_.
get());
244 std::fwrite(&density,
sizeof(
double), 1,
file_.
get());
246 std::fwrite(&weight,
sizeof(
double), 1,
file_.
get());
248 std::fwrite(&partial_weight,
sizeof(
double), 1,
file_.
get());
249 const auto type = static_cast<uint32_t>(action.
get_type());
250 std::fwrite(&type,
sizeof(uint32_t), 1,
file_.
get());
259 out_par.part_extended),
260 only_final_(out_par.part_only_final) {}
264 const char pchar =
'p';
266 std::fwrite(&pchar,
sizeof(
char), 1,
file_.
get());
273 const int event_number,
275 const char pchar =
'p';
277 std::fwrite(&pchar,
sizeof(
char), 1,
file_.
get());
283 const char fchar =
'f';
284 std::fwrite(&fchar,
sizeof(
char), 1,
file_.
get());
287 const char empty =
event.empty_event;
295 const std::unique_ptr<Clock> &,
298 const char pchar =
'p';
300 std::fwrite(&pchar,
sizeof(
char), 1,
file_.
get());
308 :
BinaryOutputBase(path /
"SMASH_IC.bin",
"wb", name, out_par.ic_extended) {
315 const int event_number,
318 const char fchar =
'f';
319 std::fwrite(&fchar,
sizeof(
char), 1,
file_.
get());
322 const char empty =
event.empty_event;
330 if (particles.
size() != 0) {
332 "End time might be too small for initial conditions output. "
333 "Hypersurface has not yet been crossed by ",
334 particles.
size(),
" particle(s).");
341 const char pchar =
'p';
342 std::fwrite(&pchar,
sizeof(
char), 1,
file_.
get());
virtual ProcessType get_type() const
Get the process type.
bool extended_
Option for extended output.
A class to pre-calculate and store parameters relevant for density calculation.
OutputOnlyFinal only_final_
Whether final- or initial-state particles should be written.
void at_eventstart(const Particles &particles, const int event_number, const EventInfo &event) override
Writes the initial particle information of an event to the binary output.
void at_eventend(const Particles &particles, const int event_number, const EventInfo &event) override
Writes the final particle information of an event to the binary output.
void at_intermediate_time(const Particles &particles, const std::unique_ptr< Clock > &clock, const DensityParameters &dens_param, const EventInfo &event) override
Writes particles at each time interval; fixed by option OUTPUT_INTERVAL.
void at_eventstart(const Particles &, const int, const EventInfo &) override
Writes the initial particle information of an event to the binary output.
virtual double get_partial_weight() const =0
Return the specific weight for the chosen outgoing channel, which is mainly used for the partial weig...
void at_eventend(const Particles &particles, const int32_t event_number, const EventInfo &event) override
Writes the final particle information list of an event to the binary output.
BinaryOutputBase(const bf::path &path, const std::string &mode, const std::string &name, bool extended_format)
Create binary output base.
Structure to contain custom data for output.
std::array< einhard::Logger<>, std::tuple_size< LogArea::AreaTuple >::value > logg
An array that stores all pre-configured Logger objects.
const ParticleList & outgoing_particles() const
Get the list of particles that resulted from the action.
void at_eventstart(const Particles &particles, const int event_number, const EventInfo &event) override
Writes the initial particle information list of an event to the binary output.
FILE * get()
Get the underlying FILE* pointer.
Print only final-state particles, and those only if the event is not empty.
Helper structure for Experiment to hold output options and parameters.
RenamingFilePtr file_
Binary particles output file path.
void write_particledata(const ParticleData &p)
Write particle data to binary output.
Abstraction of generic output.
void write(const char c)
Write byte to binary output.
virtual double get_total_weight() const =0
Return the total weight value, which is mainly used for the weight output entry.
void at_interaction(const Action &action, const double) override
Writes particles that are removed when crossing the hypersurface to the output.
void at_interaction(const Action &action, const double density) override
Writes an interaction block, including information about the incoming and outgoing particles,...
void at_eventend(const Particles &particles, const int event_number, const EventInfo &event) override
Writes the final particle information of an event to the binary output.
static constexpr int HyperSurfaceCrossing
bool print_start_end_
Write initial and final particles additonally to collisions?
bool empty_event
True if no collisions happened.
Hypersurface crossing Particles are removed from the evolution and printed to a separate output to se...
double impact_parameter
Impact parameter for collider modus, otherwise dummy.
const ParticleList & incoming_particles() const
Get the list of particles that go into the action.
BinaryOutputInitialConditions(const bf::path &path, std::string name, const OutputParameters &out_par)
Create binary initial conditions particle output.
BinaryOutputParticles(const bf::path &path, std::string name, const OutputParameters &out_par)
Create binary particle output.
BinaryOutputCollisions(const bf::path &path, std::string name, const OutputParameters &out_par)
Create binary particle output.