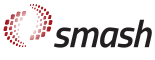 |
Version: SMASH-2.0
|
|
Go to the documentation of this file.
22 #include <boost/filesystem.hpp>
23 #include <boost/filesystem/fstream.hpp>
34 static constexpr
int LList = LogArea::List::id;
117 : shift_id_(modus_config.take({
"List",
"Shift_Id"})) {
118 std::string fd = modus_config.take({
"List",
"File_Directory"});
121 std::string fp = modus_config.take({
"List",
"File_Prefix"});
130 out <<
"-- List Modus\nInput directory for external particle lists:\n"
138 double earliest_formation_time = DBL_MAX;
139 double formation_time_difference = 0.0;
140 double reference_formation_time = 0.0;
141 bool first_particle =
true;
142 for (
const auto &particle : particles) {
143 const double t = particle.position().x0();
144 if (t < earliest_formation_time) {
145 earliest_formation_time = t;
147 if (first_particle) {
148 reference_formation_time = t;
149 first_particle =
false;
151 formation_time_difference += std::abs(t - reference_formation_time);
156 bool anti_streaming_needed = (formation_time_difference >
really_small);
158 if (anti_streaming_needed) {
159 for (
auto &particle : particles) {
161 const double t = particle.position().x0();
164 particle.position().threevec() - delta_t * particle.velocity();
166 particle.set_formation_time(t);
167 particle.set_cross_section_scaling_factor(0.0);
173 double t,
double x,
double y,
double z,
174 double mass,
double E,
double px,
double py,
176 constexpr
int max_warns_precision = 10, max_warn_mass_consistency = 10;
185 <<
" [GeV] is inconsistent with SMASH value = "
187 <<
". Forcing E = sqrt(p^2 + m^2)"
188 <<
", where m is SMASH mass.";
192 "Further warnings about SMASH mass versus input mass"
193 " inconsistencies will be suppressed.");
203 <<
"Provided 4-momentum " << particle.
momentum() <<
" and "
204 <<
" mass " << mass <<
" do not satisfy E^2 - p^2 = m^2."
205 <<
" This may originate from the lack of numerical"
206 <<
" precision in the input. Setting E to sqrt(p^2 + m^2).";
210 "Further warnings about E != sqrt(p^2 + m^2) will"
221 logg[
LList].warn() <<
"SMASH does not recognize pdg code " << pdgcode
222 <<
" loaded from file. This particle will be ignored.\n";
232 std::istringstream lineinput(line.text);
233 double t, x, y, z, mass, E, px, py, pz;
235 std::string pdg_string;
236 lineinput >> t >> x >> y >> z >> mass >> E >> px >> py >> pz >>
237 pdg_string >>
id >> charge;
238 if (lineinput.fail()) {
241 "Failed to convert the input string to the "
242 "expected data types.",
246 logg[
LList].debug(
"Particle ", pdgcode,
" (x,y,z)= (", x,
", ", y,
", ", z,
250 if (pdgcode.
charge() != charge) {
251 logg[
LList].error() <<
"Charge of pdg = " << pdgcode <<
" != " << charge;
252 throw std::invalid_argument(
"Inconsistent input (charge).");
254 try_create_particle(*particles, pdgcode, t, x, y, z, mass, E, px, py, pz);
256 if (particles->
size() > 0) {
267 std::stringstream fname;
272 const bf::path fpath = default_path / fname.str();
274 logg[
LList].debug() << fpath.filename().native() <<
'\n';
276 if (!bf::exists(fpath)) {
277 logg[
LList].fatal() << fpath.filename().native() <<
" does not exist! \n"
278 <<
"\n Usage of smash with external particle lists:\n"
279 <<
"1. Put the external particle lists in file \n"
280 <<
"File_Directory/File_Prefix{id} where {id} "
281 <<
"traversal [Shift_Id, Nevent-1]\n"
282 <<
"2. Particles info: t x y z mass p0 px py pz"
283 <<
" pdg ID charge\n"
284 <<
"in units of: fm fm fm fm GeV GeV GeV GeV GeV"
286 throw std::runtime_error(
"External particle list does not exist!");
294 bf::ifstream ifs{fpath};
308 std::string event_string;
309 const std::string needle =
"end";
311 while (getline(ifs, line)) {
312 if (line.find(needle) == std::string::npos) {
313 event_string += line +
"\n";
319 if (!ifs.eof() && (ifs.fail() || ifs.bad())) {
320 logg[
LList].fatal() <<
"Error while reading " << fpath.filename().native();
321 throw std::runtime_error(
"Error while reading external particle list");
331 std::streampos last_position) {
332 bf::ifstream ifs{filepath};
337 if (last_position == -1) {
340 ifs.seekg(last_position);
343 int skipped_lines = 0;
344 const int max_comment_lines = 4;
345 while (std::getline(ifs, line) && line[0] !=
'#' &&
346 skipped_lines++ < max_comment_lines) {
354 logg[
LList].fatal() <<
"Error while reading "
355 << filepath.filename().native();
356 throw std::runtime_error(
"Error while reading external particle list");
build_vector_< Line > line_parser(const std::string &input)
Helper function for parsing particles.txt and decaymodes.txt.
const FourVector & momentum() const
Get the particle's 4-momentum.
std::string build_error_string(std::string message, const Line &line)
Builds a meaningful error message.
double pole_mass() const
Get the particle's pole mass ("on-shell").
std::string particle_list_file_directory_
File directory of the particle list.
double initial_conditions(Particles *particles, const ExperimentParameters ¶meters)
Generates initial state of the particles in the system according to a list.
int n_warns_mass_consistency_
Counter for energy-momentum conservation warnings to avoid spamming.
Line consists of a line number and the contents of that line.
std::ostream & operator<<(std::ostream &out, const ActionPtr &action)
double sqr() const
calculate the square of the vector (which is a scalar)
int file_id_
file_id_ is the id of the current file
void set_4momentum(const FourVector &momentum_vector)
Set the particle's 4-momentum directly.
std::array< einhard::Logger<>, std::tuple_size< LogArea::AreaTuple >::value > logg
An array that stores all pre-configured Logger objects.
void backpropagate_to_same_time(Particles &particles)
Judge whether formation times are the same for all the particles; Don't do anti-freestreaming if all ...
constexpr double really_small
Numerical error tolerance.
Interface to the SMASH configuration files.
void create(size_t n, PdgCode pdg)
Add n particles of the same type (pdg) to the list.
double start_time_
Starting time for the List; changed to the earliest formation time.
void set_formation_time(const double &form_time)
Set the absolute formation time.
int charge() const
The charge of the particle.
const std::string & name() const
static constexpr int LList
void set_cross_section_scaling_factor(const double &xsec_scal)
Set the particle's initial cross_section_scaling_factor.
void try_create_particle(Particles &particles, PdgCode pdgcode, double t, double x, double y, double z, double mass, double E, double px, double py, double pz)
Tries to add a new particle to particles and performs consistency checks: (i) The PDG code is legal a...
bool file_has_events_(bf::path filepath, std::streampos last_position)
Check if the file given by filepath has events left after streampos last_position.
int n_warns_precision_
Counter for mass-check warnings to avoid spamming.
std::streampos last_read_position_
last read position in current file
std::string next_event_()
Read the next event.
void set_4position(const FourVector &pos)
Set the particle's 4-position directly.
Helper structure for Experiment.
std::string particle_list_file_prefix_
File prefix of the particle list.
int event_id_
event_id_ = the unique id of the current event
const ParticleType & type() const
Get the type of the particle.
const int shift_id_
shift_id is the start number of file_id_
ListModus()
Construct an empty list. Useful for convenient JetScape connection.
bf::path file_path_(const int file_id)
Return the absolute file path based on given integer.