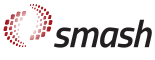 |
Version: SMASH-2.0
|
|
Go to the documentation of this file.
10 #ifndef SRC_INCLUDE_SMASH_POTENTIALS_H_
11 #define SRC_INCLUDE_SMASH_POTENTIALS_H_
57 double skyrme_pot(
const double baryon_density)
const;
73 const double baryon_density)
const;
81 double symmetry_S(
const double baryon_density)
const;
187 virtual std::tuple<ThreeVector, ThreeVector, ThreeVector, ThreeVector>
265 double dVsym_drhoI3(
const double rhoB,
const double rhoI3)
const;
285 double dVsym_drhoB(
const double rhoB,
const double rhoI3)
const;
290 #endif // SRC_INCLUDE_SMASH_POTENTIALS_H_
double symmetry_S_Pot_
Parameter S_Pot in the symmetry potential in MeV.
A class to pre-calculate and store parameters relevant for density calculation.
double skyrme_tau() const
double potential(const ThreeVector &r, const ParticleList &plist, const ParticleType &acts_on) const
Evaluates potential at point r.
double symmetry_pot(const double baryon_isospin_density, const double baryon_density) const
Evaluates symmetry potential given baryon isospin density.
static std::pair< double, int > force_scale(const ParticleType &data)
Evaluates the scaling factor of the forces acting on the particles.
bool symmetry_is_rhoB_dependent_
Whether the baryon density dependence of the symmetry potential is included.
Potentials(Configuration conf, const DensityParameters ¶meters)
Potentials constructor.
virtual std::tuple< ThreeVector, ThreeVector, ThreeVector, ThreeVector > all_forces(const ThreeVector &r, const ParticleList &plist) const
Evaluates the electrical and magnetic components of the forces at point r.
virtual bool use_symmetry() const
virtual bool use_skyrme() const
Interface to the SMASH configuration files.
double skyrme_b_
Parameters of skyrme potentials: the coefficient in front of in GeV.
double dVsym_drhoB(const double rhoB, const double rhoI3) const
Calculate the derivative of the symmetry potential with respect to the net baryon density in GeV * fm...
virtual ~Potentials()
Standard destructor.
std::pair< ThreeVector, ThreeVector > symmetry_force(const double rhoI3, const ThreeVector grad_rhoI3, const ThreeVector djI3_dt, const ThreeVector rot_jI3, const double rhoB, const ThreeVector grad_rhoB, const ThreeVector djB_dt, const ThreeVector rot_jB) const
Evaluates the electrical and magnetic components of the symmetry force.
A class that stores parameters of potentials, calculates potentials and their gradients.
double skyrme_a_
Parameter of skyrme potentials: the coefficient in front of in GeV.
double symmetry_S(const double baryon_density) const
Calculate the factor in the symmetry potential.
bool use_symmetry_
Symmetry potential on/off.
const DensityParameters param_
Struct that contains the gaussian smearing width , the distance cutoff and the testparticle number n...
double skyrme_tau_
Parameters of skyrme potentials: the power index.
std::pair< ThreeVector, ThreeVector > skyrme_force(const double density, const ThreeVector grad_rho, const ThreeVector dj_dt, const ThreeVector rot_j) const
Evaluates the electrical and magnetic components of the skyrme force.
double dVsym_drhoI3(const double rhoB, const double rhoI3) const
Calculate the derivative of the symmetry potential with respect to the isospin density in GeV * fm^3.
double skyrme_pot(const double baryon_density) const
Evaluates skyrme potential given a baryon density.
bool use_skyrme_
Skyrme potential on/off.
double symmetry_gamma_
Power in formula for :