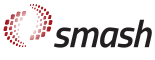 |
Version: SMASH-2.0
|
|
Go to the documentation of this file.
20 static constexpr
int LOutput = LogArea::Output::id;
228 filename_(path / (name +
".root")),
229 write_collisions_(name ==
"Collisions" || name ==
"Dileptons" ||
231 write_particles_(name ==
"Particles"),
232 write_initial_conditions_(name ==
"SMASH_IC"),
233 particles_only_final_(out_par.part_only_final),
234 autosave_frequency_(1000),
235 part_extended_(out_par.part_extended),
236 coll_extended_(out_par.coll_extended),
237 ic_extended_(out_par.ic_extended) {
279 "form_time[npart]/D");
282 "proc_id_origin[npart]/I");
284 "proc_type_origin[npart]/I");
286 "time_last_coll[npart]/D");
288 "pdg_mother1[npart]/I");
290 "pdg_mother2[npart]/I");
320 "form_time[npart]/D");
323 "proc_id_origin[npart]/I");
325 "proc_type_origin[npart]/I");
327 "time_last_coll[npart]/D");
329 "pdg_mother1[npart]/I");
331 "pdg_mother2[npart]/I");
348 const int event_number,
const EventInfo &event) {
353 test_p_ =
event.test_particles;
357 E_tot_ =
event.total_energy;
370 const std::unique_ptr<Clock> &,
374 test_p_ =
event.test_particles;
378 E_tot_ =
event.total_energy;
390 test_p_ =
event.test_particles;
394 E_tot_ =
event.total_energy;
417 if (particles.
size() != 0) {
419 "End time might be too small for initial conditions output. "
420 "Hypersurface has not yet been crossed by ",
421 particles.
size(),
" particle(s).");
439 template <
typename T>
445 bool exceeded_buffer_message =
true;
447 for (
const auto &
p : particles) {
450 if (exceeded_buffer_message) {
452 <<
"\nThe number of particles N = " << particles.size()
455 << std::ceil(particles.size() /
457 <<
" separate ROOT Tree entries will be created at this output."
458 <<
"\nMaximum buffer size (max_buffer_size_) can be changed in "
459 <<
"rootoutput.h\n\n";
460 exceeded_buffer_message =
false;
469 p0_[i] =
p.momentum().x0();
470 px_[i] =
p.momentum().x1();
471 py_[i] =
p.momentum().x2();
472 pz_[i] =
p.momentum().x3();
474 t_[i] =
p.position().x0();
475 x_[i] =
p.position().x1();
476 y_[i] =
p.position().x2();
477 z_[i] =
p.position().x3();
480 const auto h =
p.get_history();
502 const ParticleList &outgoing,
504 const double partial_weight) {
506 nin_ = incoming.size();
507 nout_ = outgoing.size();
519 for (
const ParticleList &plist : {incoming, outgoing}) {
520 for (
const auto &
p : plist) {
524 p0_[i] =
p.momentum().x0();
525 px_[i] =
p.momentum().x1();
526 py_[i] =
p.momentum().x2();
527 pz_[i] =
p.momentum().x3();
529 t_[i] =
p.position().x0();
530 x_[i] =
p.position().x1();
531 y_[i] =
p.position().x2();
532 z_[i] =
p.position().x3();
535 const auto h =
p.get_history();
virtual ProcessType get_type() const
Get the process type.
double wgt_
Property that is written to ROOT output.
bf::path filename_unfinished_
Filename of output as long as simulation is still running.
std::vector< int > charge_
Property that is written to ROOT output.
double E_tot_
Property that is written to ROOT output.
std::vector< int > pdg_mother1_
Property that is written to ROOT output.
const bool coll_extended_
Whether extended collisions output is on.
static constexpr int LOutput
A class to pre-calculate and store parameters relevant for density calculation.
std::vector< double > y_
Property that is written to ROOT output.
std::vector< double > z_
Property that is written to ROOT output.
std::vector< int > proc_id_origin_
Property that is written to ROOT output.
std::vector< int > pdg_mother2_
Property that is written to ROOT output.
std::vector< double > xsec_factor_
Property that is written to ROOT output.
std::vector< double > p0_
Property that is written to ROOT output.
void at_eventend(const Particles &particles, const int event_number, const EventInfo &event) override
update event number and impact parameter, and writes intermediate particles to a tree.
int ev_
Property that is written to ROOT output.
virtual double get_partial_weight() const =0
Return the specific weight for the chosen outgoing channel, which is mainly used for the partial weig...
std::vector< double > py_
Property that is written to ROOT output.
RootOutput(const bf::path &path, const std::string &name, const OutputParameters &out_par)
Construct ROOT output.
const bf::path filename_
Filename of output.
double E_fields_tot_
Property that is written to ROOT output.
bool write_initial_conditions_
Option to write particles tree for initial conditions.
std::vector< double > pz_
Property that is written to ROOT output.
std::vector< double > formation_time_
Property that is written to ROOT output.
int test_p_
Property that is written to ROOT output.
Structure to contain custom data for output.
std::array< einhard::Logger<>, std::tuple_size< LogArea::AreaTuple >::value > logg
An array that stores all pre-configured Logger objects.
const ParticleList & outgoing_particles() const
Get the list of particles that resulted from the action.
void init_trees()
Basic initialization routine, creating the TTree objects for particles and collisions.
double current_t_
Property that is written to ROOT output.
void at_interaction(const Action &action, const double density) override
Writes collisions to a tree defined by treename.
int output_counter_
Number of output in a given event.
int autosave_frequency_
Root file cannot be read if it was not properly closed and finalized.
bool empty_event_
Property that is written to ROOT output.
Print only final-state particles, and those only if the event is not empty.
Helper structure for Experiment to hold output options and parameters.
int nout_
Property that is written to ROOT output.
bool write_particles_
Option to write particles tree.
std::vector< int > coll_per_part_
Property that is written to ROOT output.
Abstraction of generic output.
void at_eventstart(const Particles &particles, const int event_number, const EventInfo &event) override
update event number and writes intermediate particles to a tree.
std::vector< int > pdgcode_
Property that is written to ROOT output.
virtual double get_total_weight() const =0
Return the total weight value, which is mainly used for the weight output entry.
int npart_
Property that is written to ROOT output.
static constexpr int LHyperSurfaceCrossing
double modus_l_
Property that is written to ROOT output.
TTree * collisions_tree_
TTree for collision output.
double impact_b_
Property that is written to ROOT output.
bool write_collisions_
Option to write collisions tree.
int current_event_
Number of current event.
std::vector< double > px_
Property that is written to ROOT output.
std::vector< double > t_
Property that is written to ROOT output.
std::vector< double > time_last_coll_
Property that is written to ROOT output.
std::unique_ptr< TFile > root_out_file_
Pointer to root output file.
bool empty_event
True if no collisions happened.
TTree * particles_tree_
TTree for particles output.
void collisions_to_tree(const ParticleList &incoming, const ParticleList &outgoing, const double weight, const double partial_weight)
Writes collisions to a tree defined by treename.
const bool part_extended_
Whether extended particle output is on.
int nin_
Property that is written to ROOT output.
void at_intermediate_time(const Particles &particles, const std::unique_ptr< Clock > &clock, const DensityParameters &dens_param, const EventInfo &event) override
Writes intermediate particles to a tree defined by treename, if it is allowed (i.e....
double par_wgt_
Property that is written to ROOT output.
static const int max_buffer_size_
Maximal buffer size.
Hypersurface crossing Particles are removed from the evolution and printed to a separate output to se...
void particles_to_tree(T &particles)
Writes particles to a tree defined by treename.
OutputOnlyFinal particles_only_final_
Print only final particles in the event, no intermediate output.
const ParticleList & incoming_particles() const
Get the list of particles that go into the action.
double E_kinetic_tot_
Property that is written to ROOT output.
int tcounter_
Property that is written to ROOT output.
const bool ic_extended_
Whether extended ic output is on.
std::vector< double > x_
Property that is written to ROOT output.
std::vector< int > proc_type_origin_
Property that is written to ROOT output.