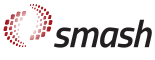 |
Version: SMASH-2.0
|
|
Go to the documentation of this file.
10 #ifndef SRC_INCLUDE_SMASH_ROOTOUTPUT_H_
11 #define SRC_INCLUDE_SMASH_ROOTOUTPUT_H_
17 #include <boost/filesystem.hpp>
88 RootOutput(
const bf::path &path,
const std::string &name,
120 const std::unique_ptr<Clock> &clock,
156 template <
typename T>
166 const ParticleList &outgoing,
const double weight,
167 const double partial_weight);
251 #endif // SRC_INCLUDE_SMASH_ROOTOUTPUT_H_
double wgt_
Property that is written to ROOT output.
bf::path filename_unfinished_
Filename of output as long as simulation is still running.
std::vector< int > charge_
Property that is written to ROOT output.
double E_tot_
Property that is written to ROOT output.
std::vector< int > pdg_mother1_
Property that is written to ROOT output.
const bool coll_extended_
Whether extended collisions output is on.
A class to pre-calculate and store parameters relevant for density calculation.
std::vector< double > y_
Property that is written to ROOT output.
std::vector< double > z_
Property that is written to ROOT output.
OutputOnlyFinal
Whether and when only final state particles should be printed.
std::vector< int > proc_id_origin_
Property that is written to ROOT output.
std::vector< int > pdg_mother2_
Property that is written to ROOT output.
std::vector< double > xsec_factor_
Property that is written to ROOT output.
std::vector< double > p0_
Property that is written to ROOT output.
void at_eventend(const Particles &particles, const int event_number, const EventInfo &event) override
update event number and impact parameter, and writes intermediate particles to a tree.
int ev_
Property that is written to ROOT output.
std::vector< double > py_
Property that is written to ROOT output.
RootOutput(const bf::path &path, const std::string &name, const OutputParameters &out_par)
Construct ROOT output.
const bf::path filename_
Filename of output.
double E_fields_tot_
Property that is written to ROOT output.
bool write_initial_conditions_
Option to write particles tree for initial conditions.
std::vector< double > pz_
Property that is written to ROOT output.
std::vector< double > formation_time_
Property that is written to ROOT output.
int test_p_
Property that is written to ROOT output.
Structure to contain custom data for output.
void init_trees()
Basic initialization routine, creating the TTree objects for particles and collisions.
double current_t_
Property that is written to ROOT output.
void at_interaction(const Action &action, const double density) override
Writes collisions to a tree defined by treename.
int output_counter_
Number of output in a given event.
int autosave_frequency_
Root file cannot be read if it was not properly closed and finalized.
bool empty_event_
Property that is written to ROOT output.
Helper structure for Experiment to hold output options and parameters.
int nout_
Property that is written to ROOT output.
bool write_particles_
Option to write particles tree.
std::vector< int > coll_per_part_
Property that is written to ROOT output.
Abstraction of generic output.
void at_eventstart(const Particles &particles, const int event_number, const EventInfo &event) override
update event number and writes intermediate particles to a tree.
std::vector< int > pdgcode_
Property that is written to ROOT output.
int npart_
Property that is written to ROOT output.
double modus_l_
Property that is written to ROOT output.
TTree * collisions_tree_
TTree for collision output.
double impact_b_
Property that is written to ROOT output.
bool write_collisions_
Option to write collisions tree.
int current_event_
Number of current event.
std::vector< double > px_
Property that is written to ROOT output.
std::vector< double > t_
Property that is written to ROOT output.
std::vector< double > time_last_coll_
Property that is written to ROOT output.
std::unique_ptr< TFile > root_out_file_
Pointer to root output file.
TTree * particles_tree_
TTree for particles output.
void collisions_to_tree(const ParticleList &incoming, const ParticleList &outgoing, const double weight, const double partial_weight)
Writes collisions to a tree defined by treename.
const bool part_extended_
Whether extended particle output is on.
int nin_
Property that is written to ROOT output.
void at_intermediate_time(const Particles &particles, const std::unique_ptr< Clock > &clock, const DensityParameters &dens_param, const EventInfo &event) override
Writes intermediate particles to a tree defined by treename, if it is allowed (i.e....
double par_wgt_
Property that is written to ROOT output.
static const int max_buffer_size_
Maximal buffer size.
void particles_to_tree(T &particles)
Writes particles to a tree defined by treename.
OutputOnlyFinal particles_only_final_
Print only final particles in the event, no intermediate output.
double E_kinetic_tot_
Property that is written to ROOT output.
int tcounter_
Property that is written to ROOT output.
const bool ic_extended_
Whether extended ic output is on.
std::vector< double > x_
Property that is written to ROOT output.
std::vector< int > proc_type_origin_
Property that is written to ROOT output.