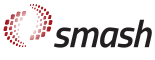 |
Version: SMASH-2.0
|
|
Go to the documentation of this file.
14 #include "Pythia8/Pythia.h"
31 bool isotropic,
double string_formation_time,
33 :
Action({in_part_a, in_part_b}, time),
34 total_cross_section_(0.),
35 isotropic_(isotropic),
36 string_formation_time_(string_formation_time) {
37 box_length_ = box_length;
93 "ScatterAction::generate_final_state: Invalid process type " +
94 std::to_string(static_cast<int>(
process_type_)) +
" was requested. " +
101 new_particle.boost_momentum(
105 new_particle.set_4position(middle_point);
111 double elastic_parameter,
bool two_to_one,
ReactionsBitSet included_2to2,
113 bool strings_switch,
bool use_AQM,
bool strings_with_probability,
114 NNbarTreatment nnbar_treatment,
double scale_xs,
double additional_el_xs) {
118 elastic_parameter, two_to_one, included_2to2, included_multi, low_snn_cut,
119 strings_switch, use_AQM, strings_with_probability, nnbar_treatment,
131 if (!strings_with_probability &&
156 return (1. / std::sqrt(1.0 -
beta_cm().sqr()));
177 const double lamb =
lambda_tilde(m_s, m1 * m1, m2 * m2);
196 " position difference [fm]: ", pos_diff,
197 ", momentum difference [GeV]: ", mom_diff);
199 const double dp2 = mom_diff.
sqr();
200 const double dr2 = pos_diff.
sqr();
205 const double dpdr = pos_diff * mom_diff;
215 const double result = dr2 - dpdr * dpdr / dp2;
216 return result > 0.0 ? result : 0.0;
225 const double mom_diff_sqr =
227 const double x_sqr = delta_x.
sqr();
235 const double p_a_dot_x = p_a.
momentum().
Dot(delta_x);
236 const double p_b_dot_x = p_b.
momentum().
Dot(delta_x);
241 (p_a_sqr * std::pow(p_b_dot_x, 2) + p_b_sqr * std::pow(p_a_dot_x, 2) -
242 2 * p_a_dot_p_b * p_a_dot_x * p_b_dot_x) /
243 (std::pow(p_a_dot_p_b, 2) - p_a_sqr * p_b_sqr);
244 return b_sqr > 0.0 ? b_sqr : 0.0;
263 return 5.5 * p8 / (7.7 + p8);
265 return std::min(9.0, 5.334 + 0.67 * (plab - 2.));
282 }
else if (plab < 0.6) {
283 return 16.53 * (plab - 0.225);
284 }
else if (plab < 1.6) {
285 return -1.63 * plab + 7.16;
292 double kinetic_energy_cm) {
315 const double mass_a = masses.first;
316 const double mass_b = masses.second;
318 const std::array<double, 2> t_range = get_t_range<double>(
319 kinetic_energy_cm, mass_in_a, mass_in_b, mass_a, mass_b);
325 double mandelstam_s_new = 0.;
341 double bb, a, plab =
plab_from_s(mandelstam_s_new);
348 a = (plab < 0.8) ? 1. : 0.64 / (plab * plab);
358 t = t_range[0] + t_range[1] - t;
362 1. - 2. * (t - t_range[0]) / (t_range[1] - t_range[0]));
375 t = t_range[0] + t_range[1] - t;
378 1. - 2. * (t - t_range[0]) / (t_range[1] - t_range[0]));
382 const std::array<double, 4>
p{1.46434, 5.80311, -6.89358, 1.94302};
383 const double a =
p[0] + mass_a * (
p[1] + mass_a * (
p[2] + mass_a *
p[3]));
387 double t = t_range[0];
392 t = t_range[0] + t_range[1] - t;
395 1. - 2. * (t - t_range[0]) / (t_range[1] - t_range[0]));
408 const double p_f =
pCM(kinetic_energy_cm, mass_a, mass_b);
411 " radial momentum: ", p_f);
450 "resonance_formation: "
451 "Incorrect number of particles in final state: ";
477 bool success =
false;
479 const int ntry_max = 10000;
480 while (!success && ntry < ntry_max) {
507 logg[
LPythia].error(
"Unknown string process required.");
511 if (ntry == ntry_max) {
530 out_mom += data.momentum();
533 logg[
LPythia].debug(
"Outgoing momenta string:", out_mom);
541 out <<
" (not performed)";
double transverse_distance_sqr() const
Calculate the transverse distance of the two incoming particles in their local rest frame.
ParticleList particle_list() const
double Dot(const FourVector &a) const
calculate the scalar product with another four-vector
static double lambda_tilde(double a, double b, double c)
Little helper function that calculates the lambda function (sometimes written with a tilde to better ...
ParticleList incoming_particles_
List with data of incoming particles.
hard string process involving 2->2 QCD process by PYTHIA.
FourVector total_momentum() const
Sum of 4-momenta of incoming particles.
const double time_of_execution_
Time at which the action is supposed to be performed (absolute time in the lab frame in fm/c).
ParticleList get_final_state()
a function to get the final state particle list which is called after the collision
double plab_from_s(double mandelstam_s, double mass)
Convert Mandelstam-s to p_lab in a fixed-target collision.
bool is_Deltastar() const
const FourVector & momentum() const
Get the particle's 4-momentum.
void resonance_formation()
Perform a 2->1 resonance-formation process.
void init(const ParticleList &incoming, double tcoll)
initialization feed intial particles, time of collision and gamma factor of the center of mass.
void assign_formation_time_to_outgoing_particles()
Assign the formation time to the outgoing particles.
double get_partial_weight() const override
Get the partial cross section of the chosen channel.
T expo(T A, T x1, T x2)
Draws a random number x from an exponential distribution exp(A*x), where A is assumed to be positive,...
void sample_2body_phasespace()
Sample the full 2-body phase-space (masses, momenta, angles) in the center-of-mass frame for the fina...
CollisionBranchList generate_collision_list(double elastic_parameter, bool two_to_one_switch, ReactionsBitSet included_2to2, MultiParticleReactionsBitSet included_multi, double low_snn_cut, bool strings_switch, bool use_AQM, bool strings_with_probability, NNbarTreatment nnbar_treatment, StringProcess *string_process, double scale_xs, double additional_el_xs) const
Generate a list of all possible collisions between the incoming particles with the given c....
void boost(const ThreeVector &v)
Apply a full Lorentz boost of momentum and position.
bool next_NDiffHard()
Hard Non-diffractive process is based on PYTHIA 8 with partonic showers and interactions.
double diffractive. Two strings are formed, one from A and one from B.
Use string fragmentation.
non-diffractive. Two strings are formed both have ends in A and B.
double cm_momentum() const
Get the momentum of the center of mass of the incoming particles in the calculation frame.
bool is_string_soft_process(ProcessType p)
Check if a given process type is a soft string excitation.
void format_debug_output(std::ostream &out) const override
int antiparticle_sign() const
double cov_transverse_distance_sqr() const
Calculate the transverse distance of the two incoming particles in their local rest frame written in ...
double sqr() const
calculate the square of the vector (which is a scalar)
void distribute_isotropically()
Populate the object with a new direction.
CollisionBranchList collision_channels_
List of possible collisions.
PdgCode pdgcode() const
Get the pdgcode of the particle.
void elastic_scattering()
Perform an elastic two-body scattering, i.e. just exchange momentum.
constexpr double nucleon_mass
Nucleon mass in GeV.
Guard type that safely disables floating point traps for the scope in which it is placed.
2->2 inelastic scattering
ThreeVector beta_cm() const
Get the velocity of the center of mass of the scattering/incoming particles in the calculation frame.
single diffractive AB->XB.
std::bitset< 10 > ReactionsBitSet
Container for the 2 to 2 reactions in the code.
void set_4momentum(const FourVector &momentum_vector)
Set the particle's 4-momentum directly.
std::array< einhard::Logger<>, std::tuple_size< LogArea::AreaTuple >::value > logg
An array that stores all pre-configured Logger objects.
StringProcess * string_process_
Pointer to interface class for strings.
T pCM(const T sqrts, const T mass_a, const T mass_b) noexcept
constexpr double really_small
Numerical error tolerance.
std::bitset< 2 > MultiParticleReactionsBitSet
Container for the 2 to 2 reactions in the code.
ScatterAction(const ParticleData &in_part1, const ParticleData &in_part2, double time, bool isotropic=false, double string_formation_time=1.0, double box_length=-1.0)
Construct a ScatterAction object.
void add_all_scatterings(double elastic_parameter, bool two_to_one, ReactionsBitSet included_2to2, MultiParticleReactionsBitSet included_multi, double low_snn_cut, bool strings_switch, bool use_AQM, bool strings_with_probability, NNbarTreatment nnbar_treatment, double scale_xs, double additional_el_xs)
Add all possible scattering subprocesses for this action object.
static double Cugnon_bpp(double plab)
Computes the B coefficients from the Cugnon parametrization of the angular distribution in elastic pp...
void string_excitation()
Todo(ryu): document better - it is not really UrQMD-based, isn't it? Perform the UrQMD-based string e...
double cm_momentum_squared() const
Get the squared momentum of the center of mass of the incoming particles in the calculation frame.
double get_total_weight() const override
Get the total cross section of scattering particles.
Soft String NNbar annihilation process can fail by lack of energy.
bool next_SDiff(bool is_AB_to_AX)
Single-diffractive process is based on single pomeron exchange described in Ingelman:1984ns .
bool next_DDiff()
Double-diffractive process ( A + B -> X + X ) is similar to the single-diffractive process,...
double string_probability(bool strings_switch, bool use_transition_probability, bool use_AQM, bool treat_nnbar_with_strings) const
const FourVector & position() const
Get the particle's position in Minkowski space.
ProcessType process_type_
type of process
double total_cross_section_
Total hadronic cross section.
static constexpr int LPythia
ProcessType get_type() const override
static constexpr int LScatterAction
The cross section class assembels everything that is needed to calculate the cross section and return...
virtual double cross_section() const
Get the total cross section of the scattering particles.
double high_energy() const
Determine the parametrized total cross section at high energies for the given collision,...
std::pair< FourVector, FourVector > get_potential_at_interaction_point() const
Get the skyrme and asymmetry potential at the interaction point.
double gamma_cm() const
Get the gamma factor corresponding to a boost to the center of mass frame of the colliding particles.
FourVector total_momentum_of_outgoing_particles() const
Calculate the total kinetic momentum of the outgoing particles.
ThreeVector velocity() const
Get the velocity (3-vector divided by zero component).
void sample_angles(std::pair< double, double > masses, double kinetic_energy_cm) override
Sample final-state angles in a 2->2 collision (possibly anisotropic).
bool isotropic_
Do this collision isotropically?
ParticleList outgoing_particles_
Initially this stores only the PDG codes of final-state particles.
virtual void sample_3body_phasespace()
Sample the full 3-body phase-space (masses, momenta, angles) in the center-of-mass frame for the fina...
ThreeVector threevec() const
(41-45) soft string excitations.
void rotate_z_axis_to(ThreeVector &r)
Rotate the z-axis onto the vector r.
double sqrt_s() const
Determine the total energy in the center-of-mass frame [GeV].
constexpr T pow_int(const T base, unsigned const exponent)
Efficient template for calculating integer powers using squaring.
a special case of baryon-antibaryon annihilation.
T power(T n, T xMin, T xMax)
Draws a random number according to a power-law distribution ~ x^n.
void add_collision(CollisionBranchPtr p)
Add a new collision channel.
elastic scattering: particles remain the same, only momenta change
void inelastic_scattering()
Perform an inelastic two-body scattering, i.e. new particles are formed.
FourVector get_interaction_point() const
Get the interaction point.
bool next_BBbarAnn()
Baryon-antibaryon annihilation process Based on what UrQMD Bass:1998ca , Bleicher:1999xi does,...
Angles provides a common interface for generating directions: i.e., two angles that should be interpr...
NNbarTreatment
Treatment of N Nbar Annihilation.
double partial_cross_section_
Partial cross-section to the chosen outgoing channel.
CollisionBranchList string_excitation(double total_string_xs, StringProcess *string_process, bool use_AQM) const
Determine the cross section for string excitations, which is given by the difference between the para...
const ParticleList & incoming_particles() const
Get the list of particles that go into the action.
void generate_final_state() override
Generate the final-state of the scattering process.
resonance formation (2->1)
const ParticleType & type() const
Get the type of the particle.
double relative_velocity() const
Get the relative velocity of the two incoming particles.
void two_to_three_scattering()
Perform a two-to-three-body scattering.
static double Cugnon_bnp(double plab)
Computes the B coefficients from the Cugnon parametrization of the angular distribution in elastic np...
ThreeVector threevec() const
void add_collisions(CollisionBranchList pv)
Add several new collision channels at once.
bool next_NDiffSoft()
Soft Non-diffractive process is modelled in accordance with dual-topological approach Capella:1978ig ...
int32_t charge() const
The charge of the particle.
double mandelstam_s() const
Determine the Mandelstam s variable,.
T pCM_sqr(const T sqrts, const T mass_a, const T mass_b) noexcept
T pCM_from_s(const T s, const T mass_a, const T mass_b) noexcept