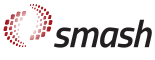 |
Version: SMASH-2.0
|
|
Go to the documentation of this file.
15 #include <boost/filesystem.hpp>
18 #include "smash/config.h"
115 const std::string &name,
118 file_{path /
"thermodynamics.dat",
"w"},
120 std::fprintf(file_.get(),
"# %s thermodynamics output\n", VERSION_MAJOR);
122 if (out_par_.td_smearing) {
123 std::fprintf(file_.get(),
"# @ point (%6.2f, %6.2f, %6.2f) [fm]\n", r.x1(),
126 std::fprintf(file_.get(),
"# averaged over the entire volume\n");
128 std::fprintf(file_.get(),
"# %s\n", to_string(out_par.td_dens_type));
129 std::fprintf(file_.get(),
"# time [fm/c], ");
130 if (out_par_.td_rho_eckart) {
131 std::fprintf(file_.get(),
"%s [fm^-3], ",
134 if (out_par_.td_tmn) {
135 if (out_par_.td_smearing) {
136 std::fprintf(file_.get(),
"%s [GeV/fm^3] 00 01 02 03 11 12 13 22 23 33, ",
139 std::fprintf(file_.get(),
"%s [GeV] 00 01 02 03 11 12 13 22 23 33, ",
143 if (out_par_.td_tmn_landau) {
144 if (out_par_.td_smearing) {
145 std::fprintf(file_.get(),
"%s [GeV/fm^3] 00 01 02 03 11 12 13 22 23 33, ",
148 std::fprintf(file_.get(),
"%s [GeV] 00 01 02 03 11 12 13 22 23 33, ",
152 if (out_par_.td_v_landau) {
153 std::fprintf(file_.get(),
"%s x y z, ",
156 if (out_par_.td_jQBS) {
157 if (out_par_.td_smearing) {
158 std::fprintf(file_.get(),
"j_QBS [(Q,B,S)/fm^3] (0 1 2 3)x3");
160 std::fprintf(file_.get(),
"j_QBS [(Q,B,S)] (0 1 2 3)x3");
163 std::fprintf(file_.get(),
"\n");
169 const int event_number,
171 std::fprintf(
file_.
get(),
"# event %i\n", event_number);
181 const Particles &particles,
const std::unique_ptr<Clock> &clock,
183 std::fprintf(
file_.
get(),
"%6.2f ", clock->current_time());
184 constexpr
bool compute_gradient =
false;
189 std::fprintf(
file_.
get(),
"%7.4f ", rho);
193 for (
const auto &
p : particles) {
194 const double dens_factor =
203 1.0 /
p.momentum().abs(), dens_param, compute_gradient)
210 Tmn.add_particle(
p, dens_factor);
216 for (
int i = 0; i < 10; i++) {
221 for (
int i = 0; i < 10; i++) {
222 std::fprintf(
file_.
get(),
"%7.4f ", Tmn_L[i]);
226 std::fprintf(
file_.
get(),
"%7.4f %7.4f %7.4f ", -u[1] / u[0],
227 -u[2] / u[0], -u[3] / u[0]);
240 std::fprintf(
file_.
get(),
"%15.12f %15.12f %15.12f %15.12f ", jQ[0], jQ[1],
242 std::fprintf(
file_.
get(),
"%15.12f %15.12f %15.12f %15.12f ", jB[0], jB[1],
244 std::fprintf(
file_.
get(),
"%15.12f %15.12f %15.12f %15.12f ", jS[0], jS[1],
251 const char *file_name,
const ParticleList &plist,
255 std::ofstream a_file;
256 a_file.open(file_name, std::ios::out);
257 const bool compute_gradient =
false;
258 const bool smearing =
true;
260 for (
int i = 0; i <= n_points; i++) {
261 r = line_start + (line_end - line_start) * (1.0 * i / n_points);
262 double rho_eck = std::get<0>(
263 current_eckart(r, plist, param, dens_type, compute_gradient, smearing));
264 a_file << r.
x1() <<
" " << r.
x2() <<
" " << r.
x3() <<
" " << rho_eck
void density_along_line(const char *file_name, const ParticleList &plist, const DensityParameters ¶m, DensityType dens_type, const ThreeVector &line_start, const ThreeVector &line_end, int n_points)
Prints density along the specified line.
ThermodynamicOutput(const bf::path &path, const std::string &name, const OutputParameters &out_par)
Construct Output param[in] path Path to output param[in] name Filename param[in] out_par Parameters o...
RenamingFilePtr file_
Pointer to output file.
A class to pre-calculate and store parameters relevant for density calculation.
bool td_jQBS
Print out QBS 4-currents or not?
bool td_v_landau
Print out Landau velocity of type td_dens_type or not?
void at_eventstart(const Particles &particles, const int event_number, const EventInfo &event) override
writes the event header
std::pair< double, ThreeVector > unnormalized_smearing_factor(const ThreeVector &r, const FourVector &p, const double m_inv, const DensityParameters &dens_par, const bool compute_gradient=false)
Implements gaussian smearing for any quantity.
const OutputParameters out_par_
Structure that holds all the information about what to printout.
Structure to contain custom data for output.
constexpr double really_small
Numerical error tolerance.
FILE * get()
Get the underlying FILE* pointer.
double density_factor(const ParticleType &type, DensityType dens_type)
Get the factor that determines how much a particle contributes to the density type that is computed.
ThreeVector td_position
Point, where thermodynamic quantities are calculated.
Helper structure for Experiment to hold output options and parameters.
bool td_rho_eckart
Print out Eckart rest frame density of type td_dens_type or not?
Abstraction of generic output.
void at_eventend(const Particles &particles, const int event_number, const EventInfo &event) override
only flushes the output the file
bool td_tmn
Print out energy-momentum tensor of type td_dens_type or not?
std::tuple< double, FourVector, ThreeVector, ThreeVector, ThreeVector > current_eckart(const ThreeVector &r, const ParticleList &plist, const DensityParameters &par, DensityType dens_type, bool compute_gradient, bool smearing)
Calculates Eckart rest frame density and 4-current of a given density type and optionally the gradien...
DensityType
Allows to choose which kind of density to calculate.
double norm_factor_sf() const
~ThermodynamicOutput()
Default destructor.
DensityType td_dens_type
Type (e.g., baryon/pion/hadron) of thermodynamic quantity.
void at_intermediate_time(const Particles &particles, const std::unique_ptr< Clock > &clock, const DensityParameters &dens_param, const EventInfo &event) override
Writes thermodynamics every fixed time interval.
bool td_smearing
Whether smearing is on or off; WARNING : if smearing is off, then final result is in GeV instead of G...
bool td_tmn_landau
Print out energy-momentum tensor in Landau rest frame (of type td_dens_type) or not?