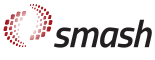 |
Version: SMASH-2.0
|
|
Go to the documentation of this file.
28 static constexpr
int LBox = LogArea::Box::id;
32 out <<
"-- Box Modus:\nSize of the box: (" << m.
length_ <<
" fm)³\n";
34 out <<
"Thermal multiplicities "
36 <<
" GeV, muS = " << m.
mus_ <<
" GeV, muQ = " << m.
muq_ <<
" GeV)\n";
40 out << ptype->
name() <<
" initial multiplicity " <<
p.second <<
'\n';
45 out <<
"All initial momenta = 3T = " << 3 * m.
temperature_ <<
" GeV\n";
48 out <<
"Boltzmann momentum distribution with T = " << m.
temperature_
52 out <<
"Fermi/Bose momentum distribution with T = " << m.
temperature_
58 out <<
"Adding a " << ptype->
name() <<
" as a jet in the middle "
59 <<
"of the box with " << m.
jet_mom_ <<
" GeV initial momentum.\n";
221 : initial_condition_(modus_config.take({
"Box",
"Initial_Condition"})),
222 length_(modus_config.take({
"Box",
"Length"})),
224 modus_config.take({
"Box",
"Equilibration_Time"}, -1.)),
225 temperature_(modus_config.take({
"Box",
"Temperature"})),
226 start_time_(modus_config.take({
"Box",
"Start_Time"}, 0.)),
228 modus_config.take({
"Box",
"Use_Thermal_Multiplicities"},
false)),
229 mub_(modus_config.take({
"Box",
"Baryon_Chemical_Potential"}, 0.)),
230 mus_(modus_config.take({
"Box",
"Strange_Chemical_Potential"}, 0.)),
231 muq_(modus_config.take({
"Box",
"Charge_Chemical_Potential"}, 0.)),
232 account_for_resonance_widths_(
233 modus_config.take({
"Box",
"Account_Resonance_Widths"},
true)),
234 init_multipl_(use_thermal_
235 ? std::map<PdgCode, int>()
236 : modus_config.take({
"Box",
"Init_Multiplicities"})
237 .convert_for(init_multipl_)),
238 insert_jet_(modus_config.has_value({
"Box",
"Jet",
"Jet_PDG"})),
239 jet_pdg_(insert_jet_ ? modus_config.take({
"Box",
"Jet",
"Jet_PDG"})
240 .convert_for(jet_pdg_)
242 jet_mom_(modus_config.take({
"Box",
"Jet",
"Jet_Momentum"}, 20.)) {
243 if (parameters.res_lifetime_factor < 0.) {
244 throw std::invalid_argument(
245 "Resonance lifetime modifier cannot be negative!");
248 if (std::abs(length_ - parameters.box_length) >
really_small) {
249 throw std::runtime_error(
"Box length inconsistency");
255 double momentum_radial = 0.0, mass = 0.0;
266 const double lifetime_factor =
275 double nb_init = 0.0, ns_init = 0.0, nq_init = 0.0;
278 particles->
create(thermal_mult_int, mult.first);
279 nb_init += mult.second * mult.first.baryon_number();
280 ns_init += mult.second * mult.first.strangeness();
281 nq_init += mult.second * mult.first.charge();
282 logg[
LBox].debug(mult.first,
" initial multiplicity ", thermal_mult_int);
284 logg[
LBox].info(
"Initial hadron gas baryon density ", nb_init);
285 logg[
LBox].info(
"Initial hadron gas strange density ", ns_init);
286 logg[
LBox].info(
"Initial hadron gas charge density ", nq_init);
290 logg[
LBox].debug(
"Particle ",
p.first,
" initial multiplicity ",
294 std::unique_ptr<QuantumSampling> quantum_sampling;
296 quantum_sampling = make_unique<QuantumSampling>(
init_multipl_, V, T);
302 momentum_radial = 3.0 * T;
303 mass = data.pole_mass();
319 mass = data.type().mass();
320 momentum_radial = quantum_sampling->sample(data.pdgcode());
324 logg[
LBox].debug(data.type().name(),
"(id ", data.id(),
325 ") radial momentum ", momentum_radial,
", direction",
327 data.set_4momentum(mass, phitheta.
threevec() * momentum_radial);
328 momentum_total += data.momentum();
331 ThreeVector pos{uniform_length(), uniform_length(), uniform_length()};
339 data.set_4momentum(data.momentum().abs(),
340 data.momentum().threevec() -
341 momentum_total.
threevec() / particles->size());
346 auto &jet_particle = particles->create(
jet_pdg_);
356 momentum_total += data.momentum();
361 logg[
LBox].debug() <<
"Initial total 4-momentum [GeV]: " << momentum_total;
366 const OutputsList &output_list) {
375 data.set_4position(position);
378 make_unique<WallcrossingAction>(incoming_particle, data);
379 for (
const auto &output : output_list) {
380 if (!output->is_dilepton_output() && !output->is_photon_output()) {
381 output->at_interaction(*action, 0.);
386 logg[
LBox].debug(
"Moved ", wraps,
" particles back into the box.");
const bool account_for_resonance_widths_
In case of thermal initialization: true – account for resonance spectral functions,...
const double muq_
Charge chemical potential for thermal initialization; only used if use_thermal_ is true.
const double mub_
Baryon chemical potential for thermal initialization; only used if use_thermal_ is true.
const std::map< PdgCode, int > init_multipl_
Particle multiplicities at initialization; required if use_thermal_ is false.
const double length_
Length of the cube's edge in fm/c.
const bool use_thermal_
Whether to use a thermal initialization for all particles instead of specific numbers.
const PdgCode jet_pdg_
Pdg of the particle to use as a jet; necessary if insert_jet_ is true, unused otherwise.
const double temperature_
Temperature of the Box in GeV.
static bool enforce_periodic_boundaries(Iterator begin, const Iterator &end, typename std::iterator_traits< Iterator >::value_type length)
Enforces periodic boundaries on the given collection of values.
const bool insert_jet_
Whether to insert a single high energy particle at the center of the box (0,0,0).
std::ostream & operator<<(std::ostream &out, const ActionPtr &action)
void distribute_isotropically()
Populate the object with a new direction.
const BoxInitialCondition initial_condition_
Initial momenta distribution: thermal or peaked momenta.
static double partial_density(const ParticleType &ptype, double T, double mub, double mus, double muq, bool account_for_resonance_widths=false)
Compute partial density of one hadron sort.
double res_lifetime_factor
Multiplicative factor to be applied to resonance lifetimes; in the case of thermal multiplicities thi...
std::array< einhard::Logger<>, std::tuple_size< LogArea::AreaTuple >::value > logg
An array that stores all pre-configured Logger objects.
constexpr double really_small
Numerical error tolerance.
static const ParticleType & find(PdgCode pdgcode)
Returns the ParticleType object for the given pdgcode.
const double mus_
Strange chemical potential for thermal initialization; only used if use_thermal_ is true.
Interface to the SMASH configuration files.
void create(size_t n, PdgCode pdg)
Add n particles of the same type (pdg) to the list.
static double sample_mass_thermal(const ParticleType &ptype, double beta)
Sample resonance mass in a thermal medium.
std::map< PdgCode, double > average_multipl_
Average multiplicities in case of thermal initialization.
const std::string & name() const
int poisson(const T &lam)
Returns a Poisson distributed random number.
uniform_dist< T > make_uniform_distribution(T min, T max)
static constexpr int LBox
ThreeVector threevec() const
double initial_conditions(Particles *particles, const ExperimentParameters ¶meters)
Generates initial state of the particles in the system according to specified parameters: number of p...
BoxModus(Configuration modus_config, const ExperimentParameters ¶meters)
Constructor.
static bool is_eos_particle(const ParticleType &ptype)
Check if a particle belongs to the EoS.
Helper structure for Experiment.
Angles provides a common interface for generating directions: i.e., two angles that should be interpr...
int impose_boundary_conditions(Particles *particles, const OutputsList &output_list={})
Enforces that all particles are inside the box at the beginning of an event.
double sample_momenta_from_thermal(const double temperature, const double mass)
Samples a momentum from the Maxwell-Boltzmann (thermal) distribution in a faster way,...
int testparticles
Number of test particle.
ThreeVector threevec() const
const double start_time_
Initial time of the box.
static const ParticleTypeList & list_all()
const double jet_mom_
Initial momentum of the jet particle; only used if insert_jet_ is true.