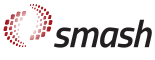 |
Version: SMASH-2.0
|
|
Go to the documentation of this file.
28 const ParticleList &in,
const double time,
const int n_frac_photons,
29 const double hadronic_cross_section_input)
31 reac_(photon_reaction_type(in)),
32 number_of_fractional_photons_(n_frac_photons),
33 hadron_out_t_(outgoing_hadron_type(in)),
34 hadron_out_mass_(sample_out_hadron_mass(hadron_out_t_)),
35 hadronic_cross_section_(hadronic_cross_section_input) {}
38 const ParticleList &in) {
93 for (
const auto &output : outputs) {
94 if (output->is_photon_output()) {
96 output->at_interaction(*
this, 0.0);
119 return rho_p_particle_ptr;
122 return rho_m_particle_ptr;
125 return rho_z_particle_ptr;
130 return pi_p_particle_ptr;
134 return pi_m_particle_ptr;
139 return pi_z_particle_ptr;
149 const ParticleList &in) {
155 const ParticleList &in) {
179 if (hadron->is_stable() && s_sqrt < hadron->mass()) {
183 }
else if (!hadron->is_stable() &&
184 s_sqrt < (hadron->min_mass_spectral() +
really_small)) {
195 <<
"Problem in ScatterActionPhoton::generate_final_state().\n";
196 throw std::runtime_error(
"");
222 const double sqrts =
sqrt_s();
223 std::array<double, 2> mandelstam_t =
get_t_range(sqrts, m1, m2, m_out, 0.0);
224 const double t1 = mandelstam_t[1];
225 const double t2 = mandelstam_t[0];
227 const double pcm_out =
pCM(sqrts, m_out, 0.0);
231 double costheta = (t -
pow_int(m2, 2) +
234 (pcm_in * (s -
pow_int(m_out, 2)) / sqrts);
238 if (costheta > 1 || costheta < -1) {
240 <<
"Cos(theta)of photon scattering out of physical bounds in "
241 "the following scattering: "
255 new_particle.set_4position(middle_point);
256 new_particle.boost_momentum(
271 weight_ = diff_xs * (t2 - t1) /
290 double reaction_cross_section) {
291 CollisionBranchPtr dummy_process = make_unique<CollisionBranch>(
299 double mass = out_t->
mass();
300 const double cms_energy =
sqrt_s();
301 if (cms_energy <= out_t->min_mass_kinematic()) {
303 "Problem in ScatterActionPhoton::sample_hadron_mass");
334 throw std::runtime_error(
335 "Invalid ReactionType in ScatterActionPhoton::rho_mass()");
340 CollisionBranchList process_list;
345 process_list.push_back(make_unique<CollisionBranch>(
352 CollisionBranchList process_list;
360 double xsection = 0.0;
389 throw std::runtime_error(
"");
403 throw std::runtime_error(
"");
415 if (xsection == 0.0) {
427 }
else if (xsection < 0) {
435 " mass rho particle: ", m_rho,
", sqrt_s: ", std::sqrt(s));
465 pow_int(FF.first, 4) * xs.first +
pow_int(FF.second, 4) * xs.second;
485 const double xs_ff =
pow_int(FF, 4) * xs;
492 const double xs_ff =
pow_int(FF, 4) * xs;
498 throw std::runtime_error(
"");
507 double diff_xsection = 0.0;
561 return diff_xsection;
566 const double E_photon) {
590 const double xs_ff =
pow_int(FF.first, 4) * diff_xs.first +
591 pow_int(FF.second, 4) * diff_xs.second;
596 return pow_int(FF, 4) * diff_xs;
600 return pow_int(FF, 4) * diff_xs;
611 const double xs_ff =
pow_int(FF, 4) * xs;
618 const double xs_ff =
pow_int(FF, 4) * xs;
624 throw std::runtime_error(
"");
630 const double Lambda = 1.0;
633 const double t_ff = 34.5096 * std::pow(E_photon, 0.737) -
634 67.557 * std::pow(E_photon, 0.7584) +
635 32.858 * std::pow(E_photon, 0.7806);
636 const double ff = 2 * Lambda2 / (2 * Lambda2 - t_ff);
642 const double Lambda = 1.0;
645 const double t_ff = -61.595 * std::pow(E_photon, 0.9979) +
646 28.592 * std::pow(E_photon, 1.1579) +
647 37.738 * std::pow(E_photon, 0.9317) -
648 5.282 * std::pow(E_photon, 1.3686);
649 const double ff = 2 * Lambda2 / (2 * Lambda2 - t_ff);
655 const double E_photon) {
664 return std::pair<double, double>(xs_pion, xs_omega);
668 const double t,
const double m_rho) {
670 const double diff_xs_omega =
673 return std::pair<double, double>(diff_xs_pion, diff_xs_omega);
ParticleList incoming_particles_
List with data of incoming particles.
std::pair< double, double > total_cross_section_pair()
For processes which can happen via (pi, a1, rho) and omega exchange, return the total cross section f...
const ReactionType reac_
Photonic process as determined from incoming particles.
const int number_of_fractional_photons_
Number of photons created for each hadronic scattering, needed for correct weighting.
static bool is_kinematically_possible(const double s_sqrt, const ParticleList &in)
Check if CM-energy is sufficient to produce hadron in final state.
static double xs_pi0_rho0_pi0(const double s, const double m_rho)
Total cross sections for given photon process:
static double xs_pi_rho_pi0(const double s, const double m_rho)
Total cross sections for given photon process:
const double hadron_out_mass_
Mass of outgoing hadron.
double total_cross_section_w_ff(const double E_photon)
Compute the total cross corrected for form factors.
Class to calculate the cross-section of a meson-meson to meson-photon process.
CollisionBranchList create_collision_branch()
Creates a CollisionBranchList containing the photon processes.
constexpr double omega_mass
omega mass in GeV.
static double xs_pi_pi_rho0(const double s, const double m_rho)
Total cross sections for given photon process:
virtual void check_conservation(const uint32_t id_process) const
Check various conservation laws.
double cm_momentum() const
Get the momentum of the center of mass of the incoming particles in the calculation frame.
void perform_photons(const OutputsList &outputs)
Create the photon final state and write to output.
static double xs_diff_pi0_rho_pi_rho_mediated(const double s, const double t, const double m_rho)
Differential cross section for given photon process.
static double xs_pi_pi0_rho(const double s, const double m_rho)
Total cross sections for given photon process:
static double xs_pi_rho0_pi(const double s, const double m_rho)
Total cross sections for given photon process:
2->2 inelastic scattering
double rho_mass() const
Find the mass of the participating rho-particle.
ReactionType
Enum for encoding the photon process.
std::array< einhard::Logger<>, std::tuple_size< LogArea::AreaTuple >::value > logg
An array that stores all pre-configured Logger objects.
constexpr int photon
Photon.
T pCM(const T sqrts, const T mass_a, const T mass_b) noexcept
static double xs_pi0_rho_pi(const double s, const double m_rho)
Total cross sections for given photon process:
constexpr double really_small
Numerical error tolerance.
static const ParticleType & find(PdgCode pdgcode)
Returns the ParticleType object for the given pdgcode.
static double xs_diff_pi_pi_rho0(const double s, const double t, const double m_rho)
Differential cross section for given photon process.
static ReactionType photon_reaction_type(const ParticleList &in)
Determine photon process from incoming particles.
static double xs_diff_pi_rho0_pi(const double s, const double t, const double m_rho)
Differential cross section for given photon process.
std::array< T, 2 > get_t_range(const T sqrts, const T m1, const T m2, const T m3, const T m4)
Get the range of Mandelstam-t values allowed in a particular 2->2 process, see PDG 2014 booklet,...
const ParticleTypePtr hadron_out_t_
ParticleTypePtr to the type of the outgoing hadron.
static double xs_diff_pi0_rho0_pi0(const double s, const double t, const double m_rho)
Differential cross section for given photon process.
static double xs_diff_pi_rho_pi0_omega_mediated(const double s, const double t, const double m_rho)
Differential cross section for given photon process.
double diff_cross_section_w_ff(const double t, const double m_rho, const double E_photon)
Compute the differential cross section corrected for form factors.
double sample_resonance_mass(const double mass_stable, const double cms_energy, int L=0) const
Resonance mass sampling for 2-particle final state with one resonance (type given by 'this') and one ...
ProcessType process_type_
type of process
double form_factor_pion(const double E_photon) const
Compute the form factor for a process with a pion as the lightest exchange particle.
static ParticleTypePtr outgoing_hadron_type(const ParticleList &in)
Return ParticleTypePtr of hadron in the out channel, given the incoming particles.
std::pair< double, double > form_factor_pair(const double E_photon)
For processes which can happen via (pi, a1, rho) and omega exchange, return the form factor for the (...
void generate_final_state() override
Generate the final-state for the photon scatter process.
static double xs_pi0_rho_pi_rho_mediated(const double s, const double m_rho)
Total cross sections for given photon process:
static constexpr int LScatterAction
static double xs_pi_rho_pi0_omega_mediated(const double s, const double m_rho)
Total cross sections for given photon process:
double weight_
Weight of the produced photon.
double hadronic_cross_section() const
Return the total cross section of the underlying hadronic scattering.
FourVector total_momentum_of_outgoing_particles() const
Calculate the total kinetic momentum of the outgoing particles.
double total_cross_section(MediatorType mediator=default_mediator_) const
Calculate the total cross section of the photon process.
double diff_cross_section(const double t, const double m_rho, MediatorType mediator=default_mediator_) const
Calculate the differential cross section of the photon process.
ParticleList outgoing_particles_
Initially this stores only the PDG codes of final-state particles.
ThreeVector threevec() const
double sqrt_s() const
Determine the total energy in the center-of-mass frame [GeV].
constexpr T pow_int(const T base, unsigned const exponent)
Efficient template for calculating integer powers using squaring.
MediatorType
Compile-time switch for setting the handling of processes which can happen via different mediating pa...
void add_collision(CollisionBranchPtr p)
Add a new collision channel.
static double xs_diff_pi_pi0_rho(const double s, const double t, const double m_rho)
Differential cross section for given photon process.
ScatterActionPhoton(const ParticleList &in, const double time, const int n_frac_photons, const double hadronic_cross_section_input)
Construct a ScatterActionPhoton object.
FourVector get_interaction_point() const
Get the interaction point.
Angles provides a common interface for generating directions: i.e., two angles that should be interpr...
constexpr std::uint32_t ID_PROCESS_PHOTON
Process ID for any photon process.
CollisionBranchList collision_processes_photons_
Holds the photon branch.
double form_factor_omega(const double E_photon) const
Compute the form factor for a process with a omega as the lightest exchange particle.
double sample_out_hadron_mass(const ParticleTypePtr out_type)
Sample the mass of the outgoing hadron.
static double xs_pi_rho_pi0_rho_mediated(const double s, const double m_rho)
Total cross sections for given photon process:
std::int32_t code() const
std::pair< double, double > diff_cross_section_pair(const double t, const double m_rho)
For processes which can happen via (pi, a1, rho) and omega exchange, return the differential cross se...
static double xs_pi0_rho_pi_omega_mediated(const double s, const double m_rho)
Total cross sections for given photon process:
static double xs_diff_pi_rho_pi0_rho_mediated(const double s, const double t, const double m_rho)
Differential cross section for given photon process.
static double xs_diff_pi0_rho_pi_omega_mediated(const double s, const double t, const double m_rho)
Differential cross section for given photon process.
double mandelstam_s() const
Determine the Mandelstam s variable,.
constexpr uint64_t pack(int32_t x, int32_t y)
Pack two int32_t into an uint64_t.
bool collision_branch_created_
Was the collision branch already created?
void add_dummy_hadronic_process(double reaction_cross_section)
Adds one hadronic process with a given cross-section.
static constexpr MediatorType default_mediator_
Value used for default exchange particle. See MediatorType.