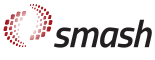 |
Version: SMASH-2.0
|
|
Go to the documentation of this file.
10 #ifndef SRC_TESTS_SETUP_H_
11 #define SRC_TESTS_SETUP_H_
13 #include "../include/smash/cxx14compat.h"
14 #include "../include/smash/decaymodes.h"
15 #include "../include/smash/experiment.h"
16 #include "../include/smash/outputinterface.h"
17 #include "../include/smash/particledata.h"
18 #include "../include/smash/particles.h"
19 #include "../include/smash/particletype.h"
20 #include "../include/smash/random.h"
22 #include <boost/filesystem.hpp>
38 #include <particles.txt.h>
50 #include <decaymodes.txt.h>
68 "# NAME MASS[GEV] WIDTH[GEV] PARITY PDG\n"
97 p.set_4position(position);
106 p.set_4momentum(momentum);
116 p.set_4position(position);
117 p.set_4momentum(momentum);
130 p.set_4position(position);
131 p.set_4momentum(momentum);
142 {random_value(), random_value(), random_value(), random_value()});
144 {random_value(), random_value(), random_value()});
164 if (!overrides.empty()) {
185 inline std::unique_ptr<ExperimentBase>
experiment(
const char *configOverrides) {
192 template <
typename G>
196 for (
auto i =
n; i; --i) {
197 list.emplace_back(generator());
209 template <
typename G>
212 for (
auto i =
n; i; --i) {
213 p->insert(generator());
223 const std::initializer_list<ParticleData> &init) {
225 for (
const auto &data : init) {
249 int testparticles = 1,
double dt = 0.1,
252 make_unique<UniformClock>(0., dt),
253 make_unique<UniformClock>(0., 1.),
277 bool empty_event =
false) {
278 return EventInfo{impact_parameter, 0.0, 0.0, 0.0, 0.0, 0.0, 1, empty_event};
287 #endif // SRC_TESTS_SETUP_H_
ParticleData smashon_random(int id=-1)
Create a particle with random position and momentum vectors and optionally a given id.
static void load_decaymodes(const std::string &input)
Loads the DecayModes map as described in the input string.
void create_smashon_particletypes()
Creates a ParticleType list containing only the smashon test particle.
void create_actual_decaymodes()
Creates the DecayModes list containing the actual decay modes that SMASH uses.
Configuration configuration(std::string overrides={})
Return a configuration object filled with data from src/config.yaml.
ParticleList create_particle_list(std::size_t n, G &&generator)
Generate a list of particles from the given generator function.
std::unique_ptr< Particles > ParticlesPtr
A type alias for a unique_ptr of Particles.
ParticleData smashon(int id=-1)
Create a particle with 0 position and momentum vectors and optionally a given id.
static void create_type_list(const std::string &particles)
Initialize the global ParticleType list (list_all) from the given input data.
EventInfo default_event_info(double impact_parameter=0.0, bool empty_event=false)
Creates default EventInfo object for testing purposes.
std::bitset< 10 > ReactionsBitSet
Container for the 2 to 2 reactions in the code.
Structure to contain custom data for output.
MultiParticleReactionsBitSet no_multiparticle_reactions()
returns BitSet for multi-particle reactions, where everything is off
static const ParticleType & find(PdgCode pdgcode)
Returns the ParticleType object for the given pdgcode.
ExperimentParameters default_parameters(int testparticles=1, double dt=0.1, CollisionCriterion crit=CollisionCriterion::Geometric)
Creates a standard ExperimentParameters object which works for almost all testing purposes.
Interface to the SMASH configuration files.
std::bitset< 2 > MultiParticleReactionsBitSet
Container for the 2 to 2 reactions in the code.
A FourVector that is marked as a momentum vector.
static constexpr double smashon_width
The decay width of the smashon particle.
CollisionCriterion
Criteria used to check collisions.
static constexpr const char smashon_pdg_string[]
The PDG code of the smashon particle.
uniform_dist< T > make_uniform_distribution(T min, T max)
static constexpr double smashon_mass
The mass of the smashon particle.
void merge_yaml(const std::string &yaml)
Merge the configuration in yaml into the existing tree.
A FourVector that is marked as a position vector.
Helper structure for Experiment.
(Default) geometric criterion.
void create_actual_particletypes()
Creates the ParticleType list containing the actual particles that SMASH uses.
std::unique_ptr< ExperimentBase > experiment(const Configuration &c=configuration())
Create an experiment.
static std::unique_ptr< ExperimentBase > create(Configuration config, const bf::path &output_path)
Factory method that creates and initializes a new Experiment<Modus>.
ParticlesPtr create_particles(int n, G &&generator)
Creates a Particles object and fills it with n particles generated by the generator function.
ReactionsBitSet all_reactions_included()
returns BitSet of 2->2 reactions, where everything is on
FourVector()
default constructor nulls the fourvector components