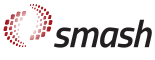 |
Version: SMASH-2.0
|
|
Go to the documentation of this file.
19 static constexpr
int LCollider = LogArea::Collider::id;
92 const std::string particle_list_file_directory =
93 config.
take({
"Custom",
"File_Directory"});
95 const std::string particle_list_file_name =
96 config.
take({
"Custom",
"File_Name"});
99 throw std::runtime_error(
100 "Your Particle List is already filled before reading in from the "
102 "Something went wrong. Please check your config.");
111 std::map<PdgCode, int> particle_list = config.
take({
"Particles"});
112 for (
const auto& particle : particle_list) {
113 if (particle.first ==
pdg::p) {
115 }
else if (particle.first ==
pdg::n) {
118 throw std::runtime_error(
119 "Your nucleus can only contain protons and/or neutrons."
120 "Please check what particles you have specified in the config");
128 const std::string path =
129 file_path(particle_list_file_directory, particle_list_file_name);
133 }
else if (!same_file) {
150 for (
const auto& it : vec) {
152 if (it.isospin == 1) {
154 }
else if (it.isospin == 0) {
157 throw std::runtime_error(
158 "Your particles charges are not 1 = proton or 0 = neutron.\n"
159 "Check whether your list is correct or there is an error.");
163 double current_mass = current_type.
mass();
165 particles_.back().set_4momentum(current_mass, 0.0, 0.0, 0.0);
187 return nucleon_position;
197 for (
auto i =
begin(); i !=
end(); i++) {
199 i->set_4momentum(i->pole_mass(), 0.0, 0.0, 0.0);
212 logg[
LCollider].warn() <<
"Fermi motion activated with a custom nucleus.\n";
213 logg[
LCollider].warn() <<
"Be aware that generating the Fermi momenta\n"
214 <<
"assumes nucleons distributed according to a\n"
215 <<
"Woods-Saxon distribution.";
219 const std::string& file_name) {
220 if (file_directory.back() ==
'/') {
221 return file_directory + file_name;
223 return file_directory +
'/' + file_name;
228 std::ifstream& infile)
const {
229 int proton_counter = 0;
230 int neutron_counter = 0;
232 std::vector<Nucleoncustom> custom_nucleus;
235 std::getline(infile, line);
239 infile.seekg(0, infile.beg);
240 std::getline(infile, line);
243 std::istringstream iss(line);
244 if (!(iss >> nucleon.
x >> nucleon.
y >> nucleon.
z >>
246 throw std::runtime_error(
247 "SMASH could not read in a line from your initial nuclei input file."
248 "\nCheck if your file has the following format: x y z "
249 "spinprojection isospin");
253 }
else if (nucleon.
isospin == 0) {
256 custom_nucleus.push_back(nucleon);
260 throw std::runtime_error(
261 "Number of protons and/or neutrons in the nuclei input file does not "
262 "correspond to the number specified in the config.\nCheck the config "
263 "and your input file.");
265 return custom_nucleus;
ThreeVector distribute_nucleon() override
Returns position of a nucleon as given in the external file.
void random_euler_angles()
Randomly generate Euler angles.
void arrange_nucleons() override
Sets the positions of the nucleons inside a nucleus.
virtual void generate_fermi_momenta()
Generates momenta according to Fermi motion for the nucleons.
CustomNucleus(Configuration &config, int testparticles, bool same_file)
Constructor that needs configuration parameters from input file and the number of testparticles.
void rotate(double phi, double theta, double psi)
Rotate vector by the given Euler angles phi, theta, psi.
std::vector< ParticleData >::iterator begin()
For iterators over the particle list:
double euler_theta_
Euler angel theta.
void align_center()
Shifts the nucleus so that its center is at (0,0,0)
bool isospin
to differentiate between protons isospin=1 and neutrons isospin=0
void generate_fermi_momenta() override
Generates Fermi momenta as it is done in the mother class but in addition prints a warning that the F...
std::array< einhard::Logger<>, std::tuple_size< LogArea::AreaTuple >::value > logg
An array that stores all pre-configured Logger objects.
virtual void set_parameters_automatic()
Sets the deformation parameters of the Woods-Saxon distribution according to the current mass number.
std::vector< ParticleData > particles_
Particles associated with this nucleus.
static const ParticleType & find(PdgCode pdgcode)
Returns the ParticleType object for the given pdgcode.
Interface to the SMASH configuration files.
std::unique_ptr< std::ifstream > filestream_
Filestream variable used if projectile and target are read in from different files and they therefore...
double euler_psi_
Euler angel psi.
std::vector< Nucleoncustom > custom_nucleus_
Vector contianing Data for one nucleus given in the particlelist.
int number_of_nucleons_
Number of nucleons per nucleus Set initally to zero to be modified in the constructor.
std::vector< ParticleData >::iterator end()
For iterators over the particle list:
int number_of_neutrons_
Number of neutrons per nucleus.
static constexpr int LCollider
std::unique_ptr< std::ifstream > * used_filestream_
Pointer to the used filestream pointer.
std::string file_path(const std::string &file_directory, const std::string &file_name)
Generates the name of the stream file.
int number_of_protons_
Number of protons per nucleus.
double euler_phi_
Euler angel phi.
Contains data for one nucleon that is read in from the list.
static std::unique_ptr< std::ifstream > filestream_shared_
Filestream variable used if projectile and target are read in from the same file and they use the sam...
bool spinprojection
spinprojection of the nucleon
Value take(std::initializer_list< const char * > keys)
The default interface for SMASH to read configuration values.
size_t index_
Index needed to read out vector in distribute nucleon.
void fill_from_list(const std::vector< Nucleoncustom > &vec)
Fills Particlelist from vector containing data for one nucleus.
std::vector< Nucleoncustom > readfile(std::ifstream &infile) const
The returned vector contains Data for one nucleus given in the particlelist.